How to Fix IndexError: Tuple Index Out of Range in Python
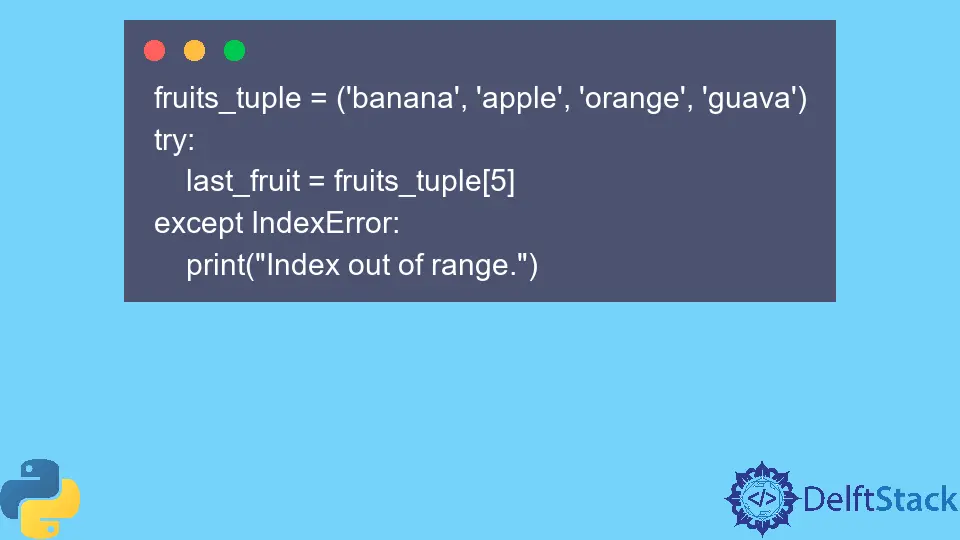
In this article, we will demonstrate why the error IndexError: tuple index out of range
occurs and how we can resolve it in Python with the help of examples.
IndexError: tuple index out of range
in Python
Before getting into the error, we must understand what a tuple is. A tuple is a collection of ordered elements enclosed in parentheses and separated by commas.
We cannot change the elements in the tuple. Hence they are also known as immutable elements
.
The most common issue we face while working with Tuples is the same error, Index out of range
. This error is because sometimes we try to access the index inside the tuple that doesn’t exist, returning the error. There can be two main reasons behind this error.
For example, if we have a tuple whose length is 7
and we want to call the last index of this tuple, instead of calling with 6
, we call it 7
. It will return the tuple index out of range
error because, like arrays, tuples also start from 0
.
The second reason is that if we are trying to access the elements using tuple syntax, the elements are not stored in a tuple; instead, they are stored in an array or a variable. It can return the same error.
To avoid these situations, we must ensure that we have a tuple and are trying to access the elements inside the range of tuples.
Let’s have an example where we will create a tuple of fruits and try to access the last index of this tuple, as shown below.
fruits_tuple = ("banana", "apple", "orange", "guava")
last_fruit = fruits_tuple[len(fruits_tuple) - 1]
print(last_fruit)
As we can see in the above code, we have created a tuple that consists of the name of 4 fruits. When calling the last element from our tuple, we have used the len()
function that will get us the length of the tuple and subtracted 1 from it so that we can get the last element of the tuple no matter the length of the tuple.
The output of the above code is shown below.
Another method to get the last element of a tuple is using the negative index, as shown below.
fruits_tuple = ("banana", "apple", "orange", "guava")
last_fruit = fruits_tuple[-1]
print(last_fruit)
Output:
There’s another way to ensure that we never get the error and lose the important data that could benefit you. The best practice is to always use try-except
statements, as shown below.
fruits_tuple = ("banana", "apple", "orange", "guava")
try:
last_fruit = fruits_tuple[5]
except IndexError:
print("Index out of range.")
Using the try-except
statements, we can ensure that if there is any error, our application doesn’t crash or stop working. Instead, we can display or store the error in the log using the except
statement.
The result of the above code is shown below.
As seen from the above example, we tried to call the 6th index of a tuple by using 5
, and our code outputs the code instead of crashing or stopping the application.
This way, if an index out of range
error is raised, it will be caught by the except
block, and we can handle it in a way that is appropriate for our program.
The solution for the second problem in which we are not sure whether we are calling an element from a variable or a tuple is using the type()
function in Python and the if-else
statement.
If the variable type is a tuple, it will execute the function. Otherwise, it will execute another function, as shown below.
fruits_tuple = ("banana", "apple", "orange", "guava")
if type(fruits_tuple) == tuple:
print(fruits_tuple[2])
else:
print("Variable is not a tuple.")
The output of the above code is shown below.
In conclusion, the tuple index out of range
error occurs when we try to access an element of a tuple using an index that is not within the range of our tuple.
It is important to use the len()
function if we are unfamiliar with the length of our tuple, we can also use try-except
blocks to catch the error before it is raised and variable type check to ensure that the index we are trying to access is within the range of the tuple and variable is of tuple
type respectively, to avoid this error.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python