How to Calculate Square Root in Python
-
Use the
math.sqrt()
to Calculate the Square Root of a Number in Python -
Use the
pow()
Function to Calculate the Square Root of a Number in Python -
Use the
**
Operator to Calculate the Square Root of a Number in Python -
Use the
cmath.sqrt()
to Calculate the Square Root of a Number in Python -
Use the
numpy.sqrt()
Method to Calculate the Square Root of a Number in Python
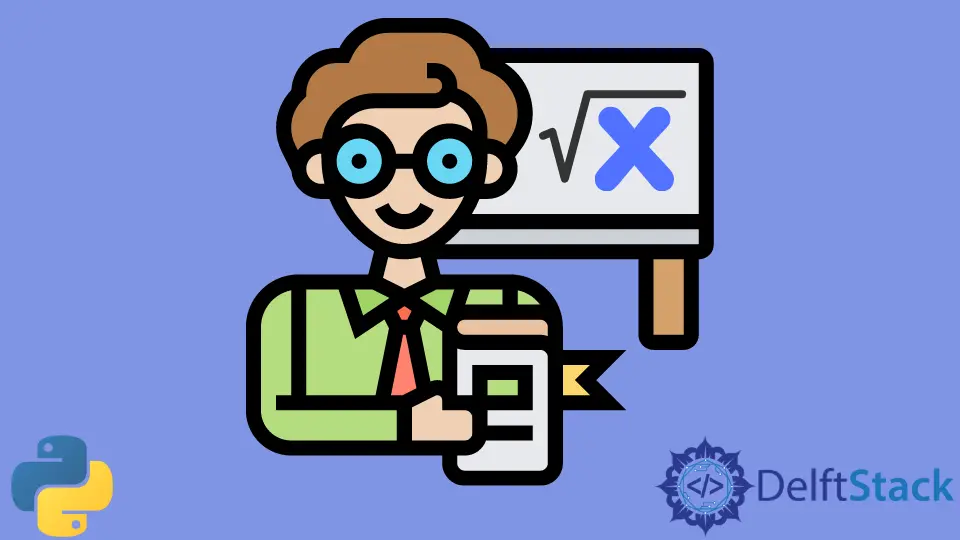
For a given number, its square root is a number whose square is equal to this number.
In Python, we can calculate the square root of a number using built-in functions and operators, which will be discussed in this tutorial.
Use the math.sqrt()
to Calculate the Square Root of a Number in Python
The math
module has different functions to perform mathematical operations in Python. The sqrt()
function returns the square root of a positive number. For example:
import math
print(math.sqrt(16))
Output:
4.0
We can also calculate the square root of a list of numbers using this function. We iterate through the list using a for
loop and apply the math.sqrt()
function to each element. See the below example code.
import math
a = [4, 2, 6]
b = []
for i in a:
b.append(math.sqrt(i))
print(b)
Output:
[2.0, 1.4142135623730951, 2.449489742783178]
Use the pow()
Function to Calculate the Square Root of a Number in Python
The square root of a number is nothing but the number raised to power 0.5. The pow()
function in Python returns the value of a number raised to some specified power and can be used to calculate the square root of a number as shown below. The first parameter of the pow()
function is the base, and the second one is the exponent.
print(pow(9, 0.5))
Output:
3.0
Use the **
Operator to Calculate the Square Root of a Number in Python
The **
operator performs the same function as the pow
method. We can use it to calculate the square root of a number as shown below.
print(9 ** (0.5))
Output:
3.0
Use the cmath.sqrt()
to Calculate the Square Root of a Number in Python
The cmath
module has methods to deal with complex numbers. The cmath.sqrt()
returns the square-root of negative or imaginary numbers. For example:
import cmath
x = -16
print(cmath.sqrt(x))
Output:
4j
Use the numpy.sqrt()
Method to Calculate the Square Root of a Number in Python
The numpy.sqrt()
method can calculate the square root of all the elements in a data object like a list or an array at once. It returns an array that contains the square root of all the elements. For example:
import numpy as np
a = [4, 2, 6]
print(np.sqrt(a))
Output:
[2. 1.41421356 2.44948974]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn