How to Implement Recursive Multiplication in Python
- Understanding Recursion
- Method 1: Basic Recursive Multiplication
- Method 2: Optimized Recursive Multiplication Using Bitwise Operations
- Method 3: Tail Recursion for Multiplication
- Conclusion
- FAQ
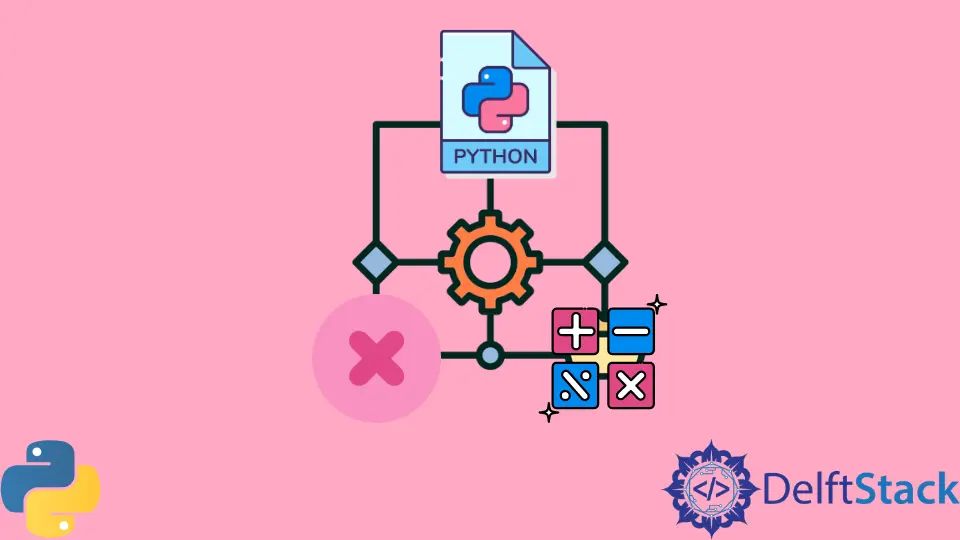
Multiplication is one of the fundamental operations in programming, yet it can be implemented in various creative ways. One such method is through recursion, a powerful concept where a function calls itself to solve a problem.
In this article, we’ll explore how to implement recursive multiplication in Python. This technique not only showcases the elegance of recursion but also helps deepen your understanding of how functions can interact with one another. Whether you’re a beginner looking to grasp the basics or an experienced developer wanting to refresh your skills, this guide will walk you through the process step by step. Let’s dive into the world of recursive multiplication and discover how to make Python work for us.
Understanding Recursion
Before we jump into coding, it’s essential to understand what recursion is. In simple terms, recursion occurs when a function calls itself to break down a problem into smaller, more manageable parts. This method is particularly useful for problems that can naturally be divided into similar sub-problems.
To implement recursive multiplication, we need to define a base case to stop the recursion and a recursive case to continue the multiplication process. The base case is crucial because it prevents the function from calling itself indefinitely, leading to a stack overflow error.
Now that we have a grasp of recursion, let’s look at how we can apply this concept to multiply two integers in Python.
Method 1: Basic Recursive Multiplication
The simplest approach to recursive multiplication involves breaking down the multiplication into repeated addition. If you want to multiply a
and b
, you can think of it as adding a
to itself b
times.
Here’s how you can implement this in Python:
def recursive_multiply(a, b):
if b == 0:
return 0
elif b < 0:
return -recursive_multiply(a, -b)
return a + recursive_multiply(a, b - 1)
result = recursive_multiply(5, 3)
Output:
15
In this code, the function recursive_multiply
takes two integers, a
and b
. The base case checks if b
is zero, in which case the function returns zero since any number multiplied by zero is zero. If b
is negative, the function calls itself with the negative of b
, ensuring that the result is negated. For positive values of b
, the function adds a
to the result of recursive_multiply(a, b - 1)
, effectively counting down until b
reaches zero.
This method is straightforward but can be inefficient for large values of b
, as it leads to many recursive calls. Nevertheless, it serves as a great introduction to the concept of recursion in Python.
Method 2: Optimized Recursive Multiplication Using Bitwise Operations
While the previous method is effective, it can be optimized further. One way to do this is by using bitwise operations, which can reduce the number of recursive calls. This method leverages the property that multiplication can be broken down using shifts and additions. Specifically, multiplying by 2 can be achieved by shifting left.
Here’s how you can implement this optimized version:
def optimized_recursive_multiply(a, b):
if b == 0:
return 0
elif b < 0:
return -optimized_recursive_multiply(a, -b)
half = optimized_recursive_multiply(a, b // 2)
if b % 2 == 0:
return half + half
else:
return half + half + a
result = optimized_recursive_multiply(5, 3)
Output:
15
In this implementation, the function optimized_recursive_multiply
first checks if b
is zero or negative, similar to the previous method. However, instead of just adding a
, it calculates half
by calling itself with b // 2
. This effectively reduces the number of additions needed. If b
is even, it simply doubles the half
value. If b
is odd, it adds one more instance of a
to the result.
This method is significantly more efficient for larger values of b
, as it reduces the depth of recursion and the number of addition operations. By leveraging the properties of binary numbers, we can achieve multiplication in a more optimized way.
Method 3: Tail Recursion for Multiplication
Another interesting approach is to use tail recursion. Tail recursion is a special case where the recursive call is the last operation in the function. This can lead to optimizations by some compilers or interpreters, allowing them to reuse stack frames.
Here’s how you can implement tail recursion for multiplication:
def tail_recursive_multiply(a, b, accumulator=0):
if b == 0:
return accumulator
elif b < 0:
return tail_recursive_multiply(a, -b, accumulator - a)
return tail_recursive_multiply(a, b - 1, accumulator + a)
result = tail_recursive_multiply(5, 3)
Output:
15
In this code, tail_recursive_multiply
takes an additional parameter, accumulator
, which keeps track of the accumulated result. The base case remains the same: when b
is zero, it returns the accumulated value. If b
is negative, it calls itself with the negated value of b
and subtracts a
from the accumulator. For positive values of b
, it adds a
to the accumulator and decrements b
.
This method is elegant and can be optimized further by Python interpreters that support tail call optimization. However, it’s worth noting that Python does not inherently optimize tail recursive calls, so while this method is conceptually interesting, it may not provide significant performance benefits in practice.
Conclusion
In this article, we’ve explored several methods to implement recursive multiplication in Python. From the basic approach of repeated addition to optimized versions using bitwise operations and tail recursion, each method offers unique insights into how recursion can be applied to solve problems. Understanding these techniques not only enhances your Python skills but also deepens your grasp of algorithmic thinking. Whether you’re working on academic projects or real-world applications, mastering recursion can be a valuable asset. Happy coding!
FAQ
-
What is recursion in programming?
Recursion in programming is a technique where a function calls itself to solve a problem by breaking it down into smaller sub-problems. -
Can recursion be used for multiplication in Python?
Yes, recursion can be effectively used to implement multiplication in Python, either through repeated addition or more optimized techniques. -
What are the advantages of using recursion?
Recursion can simplify code, reduce the need for loops, and make it easier to solve problems that can be divided into similar sub-problems. -
Is Python optimized for tail recursion?
Python does not optimize tail recursion, meaning that deep recursive calls can lead to a stack overflow error. -
How can I improve the performance of recursive multiplication?
You can improve performance by using techniques like bitwise operations or tail recursion, which reduce the number of recursive calls and operations.