How to Fix Python ValueError: Unknown Label Type: 'continuous'
-
Causes of
ValueError: Unknown label type: 'continuous'
in Python -
Use Scikit’s
LabelEncoder()
Function to FixValueError: Unknown label type: 'continuous'
-
Evaluate the Data to Fix
ValueError: Unknown label type: 'continuous'
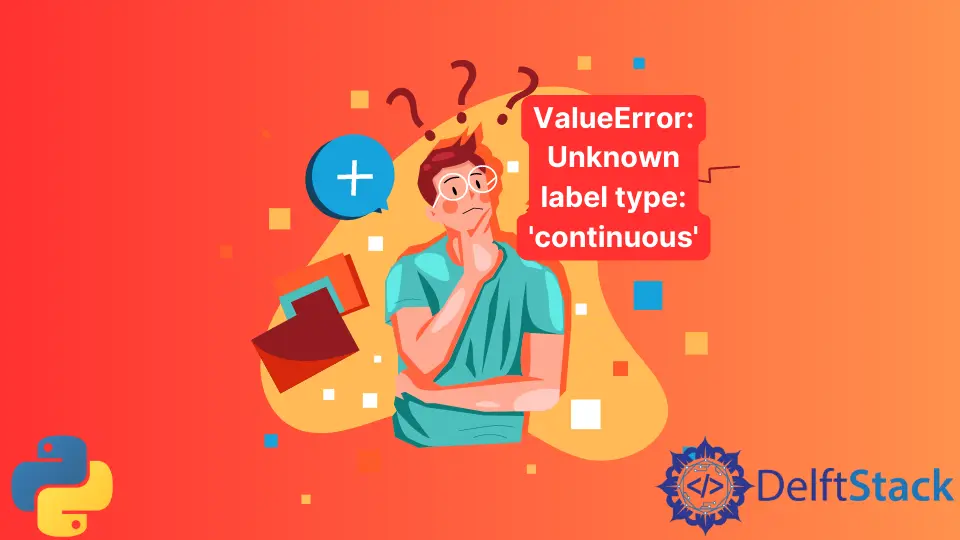
This article will tackle the causes and solutions to the ValueError: Unknown label type: 'continuous'
error in Python.
Causes of ValueError: Unknown label type: 'continuous'
in Python
Python interpreter throws this error when we try to train sklearn imported classifier on the continuous target variable.
Classifiers such as K Nearest Neighbor, Decision Tree, Logistic Regression, etc., predict the class of input variables. Class variables are in discrete or categorical forms such that 0 or 1
, True or False
, and Pass or Fail
.
If sklearn imported classification algorithm, i.e., Logistic Regression is trained on the continuous target variable, it throws ValueError: Unknown label type:'continuous'
.
Code:
import numpy as np
from sklearn.linear_model import LogisticRegression
input_var = np.array([[1.1, 1.2, 1.5, 1.6], [0.5, 0.9, 0.6, 0.8]])
target_var = np.array([1.4, 0.4])
classifier_logistic_regression = LogisticRegression()
classifier_logistic_regression.fit(input_var, target_var)
Output:
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Input In [6], in <module>
----> 1 lr.fit(x,y)
File c:\users\hp 840 g3\appdata\local\programs\python\python39\lib\site-packages\sklearn\linear_model\_logistic.py:1516, in LogisticRegression.fit(self, X, y, sample_weight)
1506 _dtype = [np.float64, np.float32]
1508 X, y = self._validate_data(
1509 X,
1510 y,
(...)
1514 accept_large_sparse=solver not in ["liblinear", "sag", "saga"],
1515 )
-> 1516 check_classification_targets(y)
1517 self.classes_ = np.unique(y)
1519 multi_class = _check_multi_class(self.multi_class, solver, len(self.classes_))
File c:\users\hp 840 g3\appdata\local\programs\python\python39\lib\site-packages\sklearn\utils\multiclass.py:197, in check_classification_targets(y)
189 y_type = type_of_target(y)
190 if y_type not in [
191 "binary",
192 "multiclass",
(...)
195 "multilabel-sequences",
196 ]:
--> 197 raise ValueError("Unknown label type: %r" % y_type)
ValueError: Unknown label type: 'continuous'
Float values as target label y are passed to the logistic regression classifier, which accepts categorical or discrete class labels. As a result, the code throws an error at the lr.fit()
function, and the model refuses to train on the given data.
Use Scikit’s LabelEncoder()
Function to Fix ValueError: Unknown label type: 'continuous'
LabelEncoder()
Function encodes the continuous target variables into discrete or categorical labels.
The classifier now accepts these values. The classifier trains on the given data and predicts the output class.
Code:
import numpy as np
from sklearn.linear_model import LogisticRegression
from sklearn import preprocessing
from sklearn import utils
input_var = np.array([[1.1, 1.2, 1.5, 1.6], [0.5, 0.9, 0.6, 0.8]])
target_var = np.array([1.4, 0.4])
predict_var = np.array([[1.3, 1.7, 1.8, 1.4], [0.2, 0.6, 0.3, 0.4]])
encoded = preprocessing.LabelEncoder()
encoded_target = encoded.fit_transform(target_var)
print(encoded_target)
classifier_logistic_regression = LogisticRegression()
classifier_logistic_regression.fit(input_var, encoded_target)
predict = classifier_logistic_regression.predict(predict_var)
print(predict)
Output:
[1 0]
[1 0]
Float values of target variable target_var
are encoded into discrete or categorical i.e. encoded_target
value using LabelEncoder()
function.
The classifier now accepts these values. The classifier is trained to predict the class of new data, denoted by predict_var
.
Evaluate the Data to Fix ValueError: Unknown label type: 'continuous'
Sometimes the data must be carefully examined to determine whether the issue is one of regression or classification. Some output variables, such as house price, cannot be classified or discretized.
In such cases, the issue is one of regression. Because the regression model accepts continuous target variables, the target variable does not need to be encoded.
Code:
import numpy as np
from sklearn.linear_model import LinearRegression
input_var = np.array([[1.1, 1.2, 1.5, 1.6], [0.5, 0.9, 0.6, 0.8]])
target_var = np.array([1.4, 0.4])
predict_var = np.array([[1.3, 1.7, 1.8, 1.4], [0.2, 0.6, 0.3, 0.4]])
linear_Regressor_model = LinearRegression()
linear_Regressor_model.fit(input_var, target_var)
predict = linear_Regressor_model.predict(predict_var)
print(predict)
Output:
[ 1.6 -0.05263158]
Float values in the output variable target_var
shows that the problem is the regression. The model must predict the value of the input variable rather than its class.
A linear regression model is trained and predicts the outcome value of new data.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python