Python ValueError: 알 수 없는 레이블 유형: '연속'
-
파이썬에서
ValueError: Unknown label type: 'continuous'
의 원인 -
Scikit의
LabelEncoder()
함수를 사용하여ValueError: Unknown label type: 'continuous'
수정 -
수정할 데이터 평가
ValueError: Unknown label type: 'continuous'
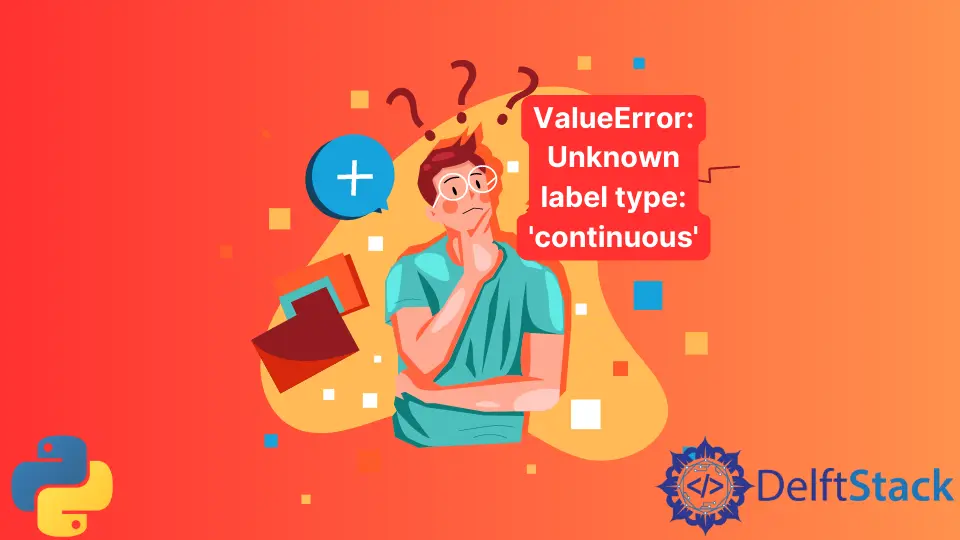
이 기사에서는 Python에서 ValueError: Unknown label type: 'continuous'
오류의 원인과 해결 방법을 다룹니다.
파이썬에서 ValueError: Unknown label type: 'continuous'
의 원인
Python 인터프리터는 연속 대상 변수에서 sklearn 가져온 분류자를 훈련하려고 할 때 이 오류를 발생시킵니다.
K Nearest Neighbor, Decision Tree, Logistic Regression 등과 같은 분류기는 입력 변수의 클래스를 예측합니다. 클래스 변수는 0 또는 1
, 참 또는 거짓
및 통과 또는 실패
와 같은 불연속형 또는 범주형 형식입니다.
sklearn 가져온 분류 알고리즘, 즉 Logistic Regression이 연속 대상 변수에 대해 학습되면 ValueError: Unknown label type:'continuous'
가 발생합니다.
암호:
import numpy as np
from sklearn.linear_model import LogisticRegression
input_var = np.array([[1.1, 1.2, 1.5, 1.6], [0.5, 0.9, 0.6, 0.8]])
target_var = np.array([1.4, 0.4])
classifier_logistic_regression = LogisticRegression()
classifier_logistic_regression.fit(input_var, target_var)
출력:
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Input In [6], in <module>
----> 1 lr.fit(x,y)
File c:\users\hp 840 g3\appdata\local\programs\python\python39\lib\site-packages\sklearn\linear_model\_logistic.py:1516, in LogisticRegression.fit(self, X, y, sample_weight)
1506 _dtype = [np.float64, np.float32]
1508 X, y = self._validate_data(
1509 X,
1510 y,
(...)
1514 accept_large_sparse=solver not in ["liblinear", "sag", "saga"],
1515 )
-> 1516 check_classification_targets(y)
1517 self.classes_ = np.unique(y)
1519 multi_class = _check_multi_class(self.multi_class, solver, len(self.classes_))
File c:\users\hp 840 g3\appdata\local\programs\python\python39\lib\site-packages\sklearn\utils\multiclass.py:197, in check_classification_targets(y)
189 y_type = type_of_target(y)
190 if y_type not in [
191 "binary",
192 "multiclass",
(...)
195 "multilabel-sequences",
196 ]:
--> 197 raise ValueError("Unknown label type: %r" % y_type)
ValueError: Unknown label type: 'continuous'
대상 레이블 y로 부동 소수점 값은 범주형 또는 불연속 클래스 레이블을 허용하는 로지스틱 회귀 분류기로 전달됩니다. 결과적으로 코드는 lr.fit()
함수에서 오류를 발생시키고 모델은 주어진 데이터에 대한 학습을 거부합니다.
Scikit의 LabelEncoder()
함수를 사용하여 ValueError: Unknown label type: 'continuous'
수정
LabelEncoder()
함수는 연속 대상 변수를 불연속 또는 범주 레이블로 인코딩합니다.
분류자는 이제 이러한 값을 허용합니다. 분류기는 주어진 데이터를 학습하고 출력 클래스를 예측합니다.
암호:
import numpy as np
from sklearn.linear_model import LogisticRegression
from sklearn import preprocessing
from sklearn import utils
input_var = np.array([[1.1, 1.2, 1.5, 1.6], [0.5, 0.9, 0.6, 0.8]])
target_var = np.array([1.4, 0.4])
predict_var = np.array([[1.3, 1.7, 1.8, 1.4], [0.2, 0.6, 0.3, 0.4]])
encoded = preprocessing.LabelEncoder()
encoded_target = encoded.fit_transform(target_var)
print(encoded_target)
classifier_logistic_regression = LogisticRegression()
classifier_logistic_regression.fit(input_var, encoded_target)
predict = classifier_logistic_regression.predict(predict_var)
print(predict)
출력:
[1 0]
[1 0]
대상 변수 target_var
의 부동 소수점 값은 LabelEncoder()
기능을 사용하여 불연속 또는 범주형 즉 encoded_target
값으로 인코딩됩니다.
분류자는 이제 이러한 값을 허용합니다. 분류기는 predict_var
로 표시되는 새 데이터의 클래스를 예측하도록 훈련됩니다.
수정할 데이터 평가 ValueError: Unknown label type: 'continuous'
경우에 따라 문제가 회귀 또는 분류 중 하나인지 확인하기 위해 데이터를 주의 깊게 조사해야 합니다. 주택 가격과 같은 일부 출력 변수는 분류하거나 이산화할 수 없습니다.
이러한 경우 문제는 회귀 중 하나입니다. 회귀 모델은 연속 대상 변수를 허용하므로 대상 변수를 인코딩할 필요가 없습니다.
암호:
import numpy as np
from sklearn.linear_model import LinearRegression
input_var = np.array([[1.1, 1.2, 1.5, 1.6], [0.5, 0.9, 0.6, 0.8]])
target_var = np.array([1.4, 0.4])
predict_var = np.array([[1.3, 1.7, 1.8, 1.4], [0.2, 0.6, 0.3, 0.4]])
linear_Regressor_model = LinearRegression()
linear_Regressor_model.fit(input_var, target_var)
predict = linear_Regressor_model.predict(predict_var)
print(predict)
출력:
[ 1.6 -0.05263158]
출력 변수 target_var
의 부동 소수점 값은 문제가 회귀임을 나타냅니다. 모델은 해당 클래스가 아닌 입력 변수의 값을 예측해야 합니다.
선형 회귀 모델을 학습하고 새 데이터의 결과 값을 예측합니다.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn관련 문장 - Python Error
- AttributeError 수정: Python에서 'generator' 객체에 'next' 속성이 없습니다.
- AttributeError 해결: 'list' 객체 속성 'append'는 읽기 전용입니다.
- AttributeError 해결: Python에서 'Nonetype' 객체에 'Group' 속성이 없습니다.
- AttributeError: 'Dict' 객체에 Python의 'Append' 속성이 없습니다.
- AttributeError: 'NoneType' 객체에 Python의 'Text' 속성이 없습니다.
- AttributeError: Int 객체에 속성이 없습니다.