How to Solve the ValueError: I/O Operation on Closed File in Python
-
Solve the
ValueError: I/O operation on closed file
Due to Improper Indentation in Python -
Solve the
ValueError: I/O operation on closed file
Due to Closing File Inside thefor
Loop in Python -
Solve the
ValueError: I/O operation on closed file
Due to Performing a Write Operation on a Closed File - Conclusion
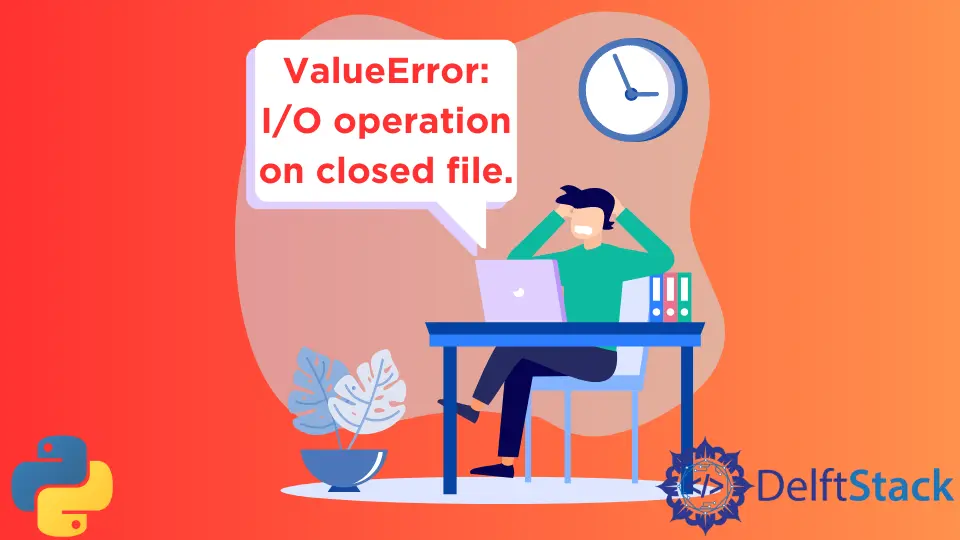
Resource management is an important factor in programming. But often, programmers unknowingly leave a memory block open, which can cause memory overflows.
This article, however, looks upon an error in Python: ValueError: I/O operation on closed file
. This happens when the programmer tries to perform operations on a file that gets somehow closed in between operations.
There are mainly three cases where the ValueError: I/O operation on closed file
can occur:
Solve the ValueError: I/O operation on closed file
Due to Improper Indentation in Python
Suppose a programmer has a .csv
file that she tries to load into the memory using a Python compiler. In Python, an object variable must be created to load the file’s contents to read or write on a file.
Let’s understand this through the below program:
import csv
# Open the file in Read mode
with open("sample_submission.csv", "r") as employees:
read_csv = csv.reader(employees)
This program imports the library package csv
to read .csv
files. In the second line of code, the program uses the with
statement to create an exception handling block and stores the .csv
file, sample_submission.csv
, inside object employees
as a readable entity by using the keyword r
.
We need to allocate some memory to read the entity employees
. Line 3 of the code allocates a memory block to read_csv
to store the contents from employees
.
If the programmer wants to display the rows from the .csv
file, she needs to print the rows from the object read_csv
inside a for
loop, just like in the code below:
import csv
# Open the file in Read mode
with open("sample_submission.csv", "r") as employees:
read_csv = csv.reader(employees)
# iterate and print the rows of csv
for row in read_csv:
print("Rows: ", row)
But when the programmer tries to compile this piece of code, she receives an error:
"C:\Users\Win 10\main.py"
Traceback (most recent call last):
File "C:\Users\Win 10\main.py", line 8, in <module>
for row in read_csv:
ValueError: I/O operation on closed file.
Process finished with exit code 1
The ValueError: I/O operation on closed file
happened because of the exception handling statement with
. As said earlier, the with
statement creates an exception handling block, and any operation initiated inside will terminate as soon as the compiler gets out of this block.
In the above program, an indention mistake caused the error. Python compilers do not use a semi-colon to identify the end of a line; instead, it uses space.
In the code, the for
loop was created outside the with
block, thus closing the file. Even though the for
loop was written just below with
, improper indention made the compiler think that the for
loop is outside the with
block.
A solution to this problem is identifying the proper indents like the following:
import csv
with open("sample_submission.csv", "r") as employees:
read_csv = csv.reader(employees)
# for loop is now inside the with block
for row in read_csv:
print("Rows: ", row)
Output:
"C:\Users\Win 10\main.py"
Rows: ['Employee ID', 'Burn Rate']
Rows: ['fffe32003000360033003200', '0.16']
Rows: ['fffe3700360033003500', '0.36']
Rows: ['fffe31003300320037003900', '0.49']
Rows: ['fffe32003400380032003900', '0.2']
Rows: ['fffe31003900340031003600', '0.52']
Process finished with exit code 0
Solve the ValueError: I/O operation on closed file
Due to Closing File Inside the for
Loop in Python
This example shows how the ValueError: I/O operation on closed file
can occur without using the with
statement. When a Python script opens a file and writes something on it inside a loop, it must be closed at the end of the program.
But the ValueError: I/O operation on closed file
can arise due to an explicit file closing inside the loop. As explained above, the with
block closes whatever has been initiated inside it.
But in cases where for
loops, etc., have been used, the ValueError: I/O operation on closed file
occurs when the file gets closed midway in the loop. Let’s see how this happens through the program below:
a = 0
b = open("sample.txt", "r")
c = 5
f = open("out" + str(c) + ".txt", "w")
for line in b:
a += 1
f.writelines(line)
if a == c:
a = 0
f.close()
f.close()
The above code reads contents from the file sample.txt
and then writes those contents in a new file with the name out(value of c).txt
.
The variable b
is loaded with the sample.txt
file, while the variable f
is used to write on a new file. The for
loop runs for the number of lines inside the file loaded inside b
.
Each iteration increases a
, while at the iteration when a=5
, the value of a
is reset to zero.
After completing the process, f.close()
is used twice. The first f.close
clears f
, while the second one clears b
.
But the program had to run more iterations before the file got closed. When the program is compiled, it gives the following output:
"C:\Users\Win 10\main.py"
Traceback (most recent call last):
File "C:\Users\Win 10\main.py", line 8, in <module>
f.writelines(line)
ValueError: I/O operation on closed file.
Process finished with exit code 1
This happens because the file is closed inside the for
loop, which makes it unable to read the file for subsequent iterations.
As this error is caused accidentally, solving it requires going back to the code and rechecking where the file gets closed. If it is a for
loop, then a file should be closed outside the indent of the loop, which allows the loop to complete all its iterations, and then release the memory.
a = 0
b = open("sample.txt", "r")
c = 5
f = open("out" + str(c) + ".txt", "w")
for line in b:
a += 1
f.writelines(line)
if a == c:
a = 0
f.close()
Here, the file is closed outside the for
loop’s indent, so the compiler closes the file after completing all the iterations.
When the code is compiled, it throws no errors while creating a file named out5.txt
:
"C:\Users\Win 10\main.py"
Process finished with exit code 0
Solve the ValueError: I/O operation on closed file
Due to Performing a Write Operation on a Closed File
This is a case scenario where the programmer gives written instructions to a file that has been closed before, and compiling it produces the ValueError: I/O operation on closed file
error.
Let’s look at an example:
with open("gh.txt", "w") as b:
b.write("Apple\n")
b.write("Orange \n")
b.write("Guava \n")
b.close()
b.write("grapes")
The program loads a .txt
file as object b
. Then this object variable b
is used to perform write operations inside the .txt
file.
When this code is compiled, the ValueError: I/O operation on closed file
occurs.
"C:\Users\Win 10\main.py"
Traceback (most recent call last):
File "C:\Users\Win 10\main.py", line 6, in <module>
b.write("grapes")
ValueError: I/O operation on closed file.
Process finished with exit code 1
This was caused by the b.close()
statement, which was written over a written statement. The compiler does not let the file be written anymore, even inside the with
block.
To solve this issue, either the program should be re-written without the with
statement if adding b.close
is required:
b = open("gh.txt", "w")
b.write("Apple\n")
b.write("Orange \n")
b.write("Guava \n")
b.write("grapes")
b.close()
Or the b.close()
statement must be removed from the with
statement to make it run:
with open("gh.txt", "w") as b:
b.write("Apple\n")
b.write("Orange \n")
b.write("Guava \n")
b.write("grapes")
Both the code blocks execute the same work, but using the with
statement adds exception handling and clears up the code.
Conclusion
This article has explained the various reasons that can cause the ValueError: I/O operation on closed file
. The reader should understand the with
statements and use them correctly in the future.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python