How to Fix Python TypeError: Unsupported Operand Type(s) for +: 'NoneType' and 'Int'
-
the
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
in Python -
Fix the
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
in Python -
Fix the
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
Inside a Function
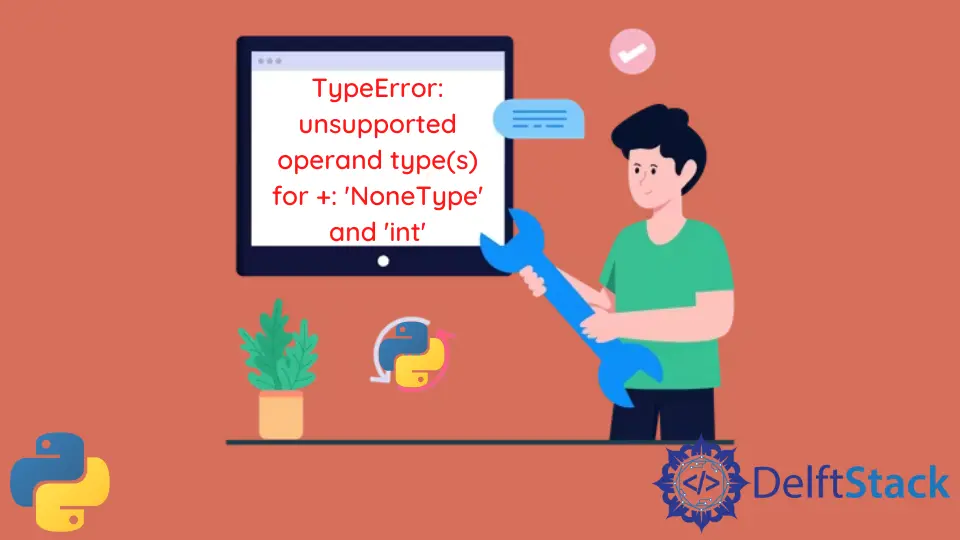
In Python the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
occurs when you add an integer value with a null
value. We’ll discuss in this article the Python error and how to resolve it.
the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
in Python
The Python compiler throws the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
because we are manipulating two values with different datatypes. In this case, the data types of these values are int
and null
, and the error is educating you that the operation is not supported because the operand int
and null
for the +
operator are invalid.
Code Example:
a = None
b = 32
print("The data type of a is ", type(a))
print("The data type of b is ", type(b))
# TypeError --> unsupported operand type(s) for +: 'NoneType' and 'int'
result = a + b
Output:
The data type of a is <class 'NoneType'>
The data type of b is <class 'int'>
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
As we see in the output of the above program, the data type of a
is NoneType
, whereas the data type of b
is int
. When we try to add the variables a
and b
, we encounter the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
.
Here are a few similar cases that also cause TypeError because their data types differ.
# Adding a string and an integer
a = "string"
b = 32
a + b # --> Error
# Adding a Null value and a string
a = None
b = "string"
a + b # --> Error
# Adding char value and a float
a = "D"
b = 1.1
a + b # --> Error
Fix the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
in Python
You cannot use null
to perform arithmetic operations on it, and the above program has demonstrated that it throws an error. Furthermore, if you have any similar case, you typecast the values before performing any arithmetic operations or other desired tasks.
To fix this error, you can use valid data types to perform any arithmetic operations on it, typecast the values, or if your function returns null
values, you can use try-catch
blocks to save your program from crashing.
Code Example:
a = "Delf"
b = "Stack"
print("The data type of a is ", type(a))
print("The data type of b is ", type(b))
result = a + b
print(result)
Output:
The data type of a is <class 'str'>
The data type of b is <class 'str'>
DelfStack
As you can see, we have similar data types for a
and b
, which are perfectly concatenated without throwing any error because the nature of both variables is the same.
Fix the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
Inside a Function
Code Example:
def sum_ab(a, b=None):
# TypeError: unsupported operand type(s) for +: 'int' and 'NoneType'
return a + b
sum_ab(3)
Output:
TypeError: unsupported operand type(s) for +: 'int' and 'NoneType'
In the above code, the sum_ab()
function has two arguments, a
and b
, whereas b
is assigned to a null
value its the default argument, and the function returns the sum of a
and b
.
Let’s say you have provided only one parameter, sum_ab(3)
. The function will automatically trigger the default parameter to None
, which cannot be added, as seen in the above examples.
In this case, if you are unsure what function raised the TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
, you can use the try-catch
mechanism to overcome such errors.
Code Example:
try:
def sum_ab(a, b=None):
return a + b
sum_ab(3)
except TypeError:
print(
" unsupported operand type(s) for +: 'int' and 'NoneType' \n The data types are a and b are invalid"
)
Output:
unsupported operand type(s) for +: 'int' and 'NoneType'
The data types are a and b are invalid
The try-catch
block helps you interpret the errors and protect your program from crashing.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python