Python TypeError: + のサポートされていないオペランド型: 'NoneType' および 'Int'
-
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
in Python -
Python の
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
を修正する -
関数内の
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
を修正する
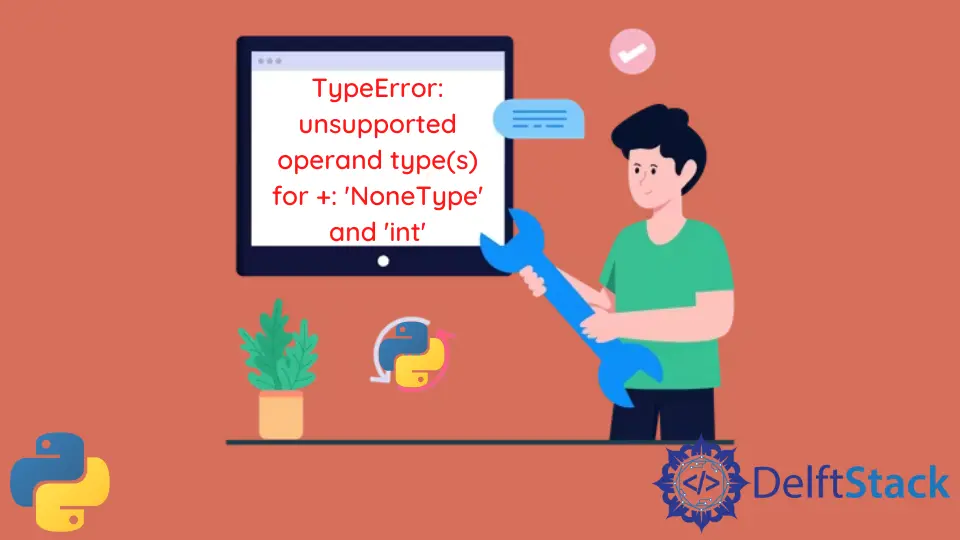
Python では、null
値を持つ整数値を追加すると、TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
が発生します。 この記事では、Python エラーとその解決方法について説明します。
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
in Python
Python コンパイラは TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
をスローします。これは、データ型が異なる 2つの値を操作しているためです。 この場合、これらの値のデータ型は int
と null
であり、+
演算子のオペランド int
と null
が無効であるため、操作がサポートされていないというエラーが表示されます。
コード例:
a = None
b = 32
print("The data type of a is ", type(a))
print("The data type of b is ", type(b))
# TypeError --> unsupported operand type(s) for +: 'NoneType' and 'int'
result = a + b
出力:
The data type of a is <class 'NoneType'>
The data type of b is <class 'int'>
TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
上記のプログラムの出力に見られるように、a
のデータ型は NoneType
ですが、b
のデータ型は int
です。 変数 a
と b
を追加しようとすると、TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
が発生します。
以下は、データ型が異なるために TypeError が発生する同様のケースです。
# Adding a string and an integer
a = "string"
b = 32
a + b # --> Error
# Adding a Null value and a string
a = None
b = "string"
a + b # --> Error
# Adding char value and a float
a = "D"
b = 1.1
a + b # --> Error
Python の TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
を修正する
null
を使用して算術演算を実行することはできず、上記のプログラムはエラーをスローすることを示しています。 さらに、同様のケースがある場合は、算術演算やその他の必要なタスクを実行する前に値を型キャストします。
このエラーを修正するには、有効なデータ型を使用して算術演算を実行し、値を型キャストするか、関数が null
値を返す場合、try-catch
ブロックを使用してプログラムがクラッシュしないようにします。
コード例:
a = "Delf"
b = "Stack"
print("The data type of a is ", type(a))
print("The data type of b is ", type(b))
result = a + b
print(result)
出力:
The data type of a is <class 'str'>
The data type of b is <class 'str'>
DelfStack
ご覧のとおり、a
と b
のデータ型は似ていますが、両方の変数の性質が同じであるため、エラーをスローすることなく完全に連結されています。
関数内の TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
を修正する
コード例:
def sum_ab(a, b=None):
# TypeError: unsupported operand type(s) for +: 'int' and 'NoneType'
return a + b
sum_ab(3)
出力:
TypeError: unsupported operand type(s) for +: 'int' and 'NoneType'
上記のコードでは、sum_ab()
関数は a
と b
の 2つの引数を持ちますが、b
はデフォルトの引数である null
値に割り当てられ、関数は a の合計を返します。
およびb
。
sum_ab(3)
という 1つのパラメーターのみを指定したとします。 上記の例に見られるように、この関数は自動的にデフォルト パラメータを None
にトリガーしますが、これは追加できません。
この場合、TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
を引き起こした関数がわからない場合は、try-catch
メカニズムを使用してそのようなエラーを克服できます。
コード例:
try:
def sum_ab(a, b=None):
return a + b
sum_ab(3)
except TypeError:
print(
" unsupported operand type(s) for +: 'int' and 'NoneType' \n The data types are a and b are invalid"
)
出力:
unsupported operand type(s) for +: 'int' and 'NoneType'
The data types are a and b are invalid
try-catch
ブロックは、エラーを解釈し、プログラムをクラッシュから保護するのに役立ちます。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn関連記事 - Python Error
- AttributeError の解決: 'list' オブジェクト属性 'append' は読み取り専用です
- AttributeError の解決: Python で 'Nonetype' オブジェクトに属性 'Group' がありません
- AttributeError: 'generator' オブジェクトに Python の 'next' 属性がありません
- AttributeError: 'numpy.ndarray' オブジェクトに Python の 'Append' 属性がありません
- AttributeError: Int オブジェクトに属性がありません
- AttributeError: Python で 'Dict' オブジェクトに属性 'Append' がありません