How to Solve the TypeError: Nonetype Object Is Not Subscriptable in Python
-
the
TypeError: 'NoneType' object is not subscriptable
in Python -
Solve the
TypeError: 'NoneType' object is not subscriptable
in Python - Conclusion
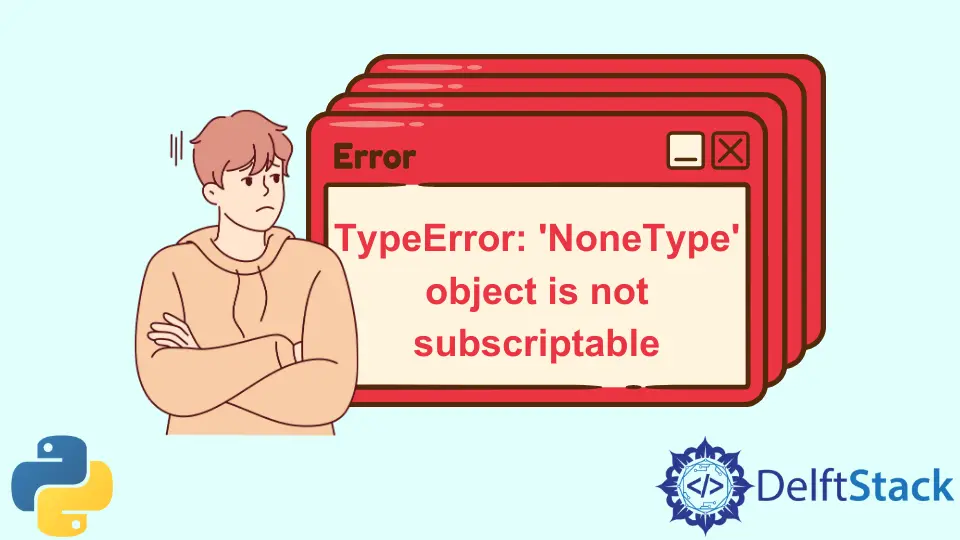
Python has various sequence data types like lists, tuples, and dictionaries that support indexing. Since the term subscript refers to the value used while indexing, these objects are also known as subscriptable objects.
In Python, incorrect indexing of such subscriptable objects often results in TypeError: 'NoneType' object is not subscriptable
. In this article, we will discuss this error and the possible solutions to fix the same. If you’re dealing with None
values frequently, check out [Check if a Variable is None in Python](HowTo/Python/check if variable is none python.en.md).
Ready? Let’s begin!
the TypeError: 'NoneType' object is not subscriptable
in Python
Before we look at why the TypeError: 'NoneType' object is not subscriptable
occurs and how to fix it, let us first get some basics out of the way.
Introduction to NoneType and Subscriptable Objects in Python
In Python, a NoneType
object is an object that has no value. In other words, it is an object that does not return anything.
The value None
is often returned by functions searching for something but not finding it. Below is an example where a function returns the None
value.
def none_demo():
for i in [1, 2, 3, 4, 5]:
if i == 10:
return yes
ans = none_demo()
print(ans)
Output:
None
Talking about subscriptable objects, as the name says, these are Python objects that can be subscripted or indexed. In simpler words, subscriptable objects are those objects that can be accessed or traversed with the help of index values like 0, 1, and so on.
Lists, tuples, and dictionaries are examples of such objects. Below is a code that shows how you can traverse a list with the help of indexing.
cakes = ["Mango", "Vanilla", "Chocolate"]
for i in range(0, 3):
print(cakes[i])
Output:
Mango
Vanilla
Chocolate
Now you know the basics well, so let’s move forward!
Solve the TypeError: 'NoneType' object is not subscriptable
in Python
In Python, there are some built-in functions like reverse()
, sort()
, and append()
that we can use on subscriptable objects. But if we assign the results of these built-in functions to a variable, it results in the TypeError: 'NoneType' object is not subscriptable
.
Look at the example given below. Here, we used the reverse()
function on the list called desserts
and stored the resultant in the variable ans
.
Then, we printed the variable ans
value, which turns out to be None
as seen in the output. However, the last statement leads to the TypeError: 'NoneType' object is not subscriptable
.
Any guesses why this happened?
desserts = ["cakes", "pie", "cookies"]
ans = desserts.reverse()
print("The variable ans contains: ", ans)
print(ans[0])
Output:
The variable ans contains: None
Traceback (most recent call last):
File "<string>", line 4, in <module>
TypeError: 'NoneType' object is not subscriptable
This happened because, in the last line, we are subscripting the variable ans
. We know that the variable ans
contains the value None
, which is not even a sequence data type; hence, it can’t be accessed by indexing.
The important thing to understand here is that although we are assigning the reversed list to the variable ans
, that does not make that variable ans
of a list type. Thus, we cannot access the variable ans
using indexing, thinking of it as a list.
In reality, the variable ans
is a NoneType
object, and Python does not support indexing such objects. Thus, one must not try to index a non-subscriptable object, or it will lead to the TypeError: 'NoneType' object is not subscriptable
.
To rectify this issue from the above code, follow the approach below.
This time we do not assign the result of the reverse()
operation to any variable. That way, the function will automatically replace the current list with the reversed list without any extra space.
Later, we can print the list as we want. Here, we are first printing the entire reversed list and then accessing the first element using the subscript 0.
As you can see, the code runs fine and gives the desired output.
desserts = ["cakes", "pie", "cookies"]
desserts.reverse()
print(desserts)
print(desserts[0])
Output:
['cookies', 'pie', 'cakes']
cookies
The same rule of not assigning the result to a variable and then indexing it applies to other functions like sort()
and append()
too. Below is an example that uses the sort()
function and runs into the TypeError: 'NoneType' object is not subscriptable
.
desserts = ["cakes", "pie", "cookies"]
ans = desserts.sort()
print("The value of the variable is: ", ans)
print(ans[1])
Output:
The value of the variable is: None
Traceback (most recent call last):
File "<string>", line 4, in <module>
TypeError: 'NoneType' object is not subscriptable
Again, this happens for the same reason. We have to drop the use of another variable to store the sorted list to get rid of this error.
This is done below.
desserts = ["cakes", "pie", "cookies"]
desserts.sort()
print(desserts)
print(desserts[1])
Output:
['cakes', 'cookies', 'pie']
cookies
You can see that, this time, the sort()
method replaces the list with the new sorted list without needing any extra space. Later, we can access the individual elements of the sorted list using indexing without worrying about this error!
And here is something interesting. Ready to hear it?
In the case of the sort()
function, if you still want to use a separate variable to store the sorted list, you can use the sorted()
function. This is done below.
desserts = ["cakes", "pie", "cookies"]
ans = sorted(desserts)
print(ans)
print(ans[0])
Output:
['cakes', 'cookies', 'pie']
cakes
You can see that this does not lead to any error because the sorted()
function returns a sorted list, unlike the sort()
method that sorts the list in place.
But unfortunately, we do not have such alternate functions for reverse()
and append()
methods.
This is it for this article. Refer to this documentation to know more about this topic.
Conclusion
This article taught us about the 'NoneType' object not subscriptable
TypeError in Python. We saw how assigning the values of performing operations like reverse()
and append()
on sequence data types to variables leads to this error.
We also saw how we could fix this error by using the sorted()
function instead of the sort()
function to avoid getting this error in the first place.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python