How to Fix Resolve TypeError: Module Object Is Not Callable in Python
-
Cause of the
TypeError: 'module' object is not callable
in Python -
Resolve the
TypeError: 'module' object is not callable
in Python
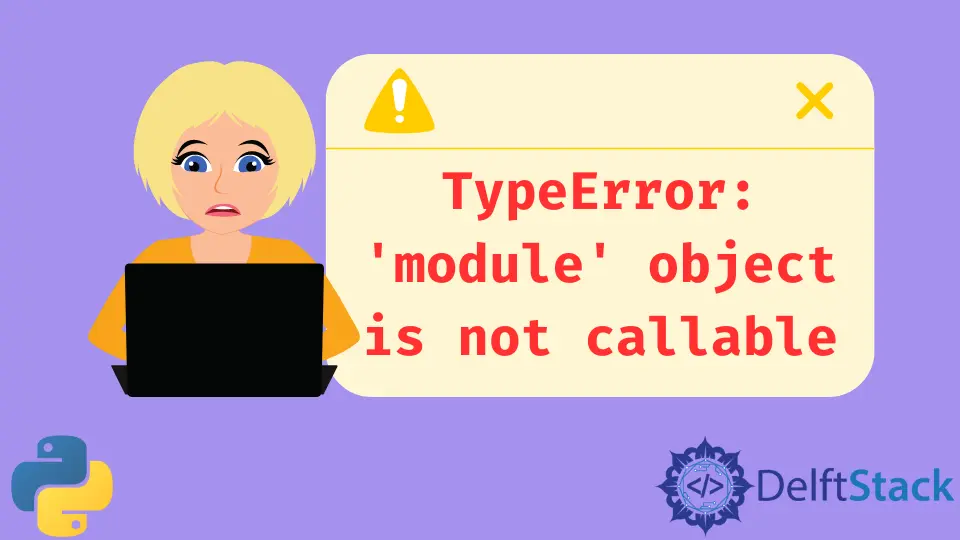
Every programming language encounters many errors. Some occur at the compile time, some at run time.
This article will discuss the TypeError: 'module' object is not callable
. This error arises when the class/method
and module
have the same names; we get confused between them because of the same names.
When we import a module, not a method, calling that module will give the error because the module is not callable. We can only call the method.
Cause of the TypeError: 'module' object is not callable
in Python
We will import a built-in module, socket
, in the following code. This module further contains a class named socket()
.
Here, the method name and the class name are the same. If we only import the socket
and call it, the interpreter will throw a TypeError: 'module' object is not callable
.
Example Code:
# Python 3.x
import socket
socket()
Output:
#Python 3.x
Traceback (most recent call last):
File "<string>", line 2, in <module>
TypeError: 'module' object is not callable
We can also face this error in the case of custom modules that are defined by ourselves. We have created the following code and saved it in a file named infomodule.py
.
Example Code:
# Python 3.x
def infomodule():
info = "meeting at 10:00 am."
print(info)
Then we created another Python file and wrote the following code, calling the module infomodule.py
. We will get the TypeError: 'module' object is not callable
.
Example Code:
# Python 3.x
import infomodule
Print(infomodule())
Output:
#Python 3.x
Traceback (most recent call last):
File "mycode.py", line 3, in <module>
print(infomodule())
TypeError: 'module' object is not callable
Resolve the TypeError: 'module' object is not callable
in Python
Call the Method/Class From the Module
To fix this error, we can import the class from the module instead of importing the module directly. It will fix the TypeError : module object is not callable
.
Here, we successfully created an object of the class socket
.
Example Code:
# Python 3.x
import socket
socket.socket()
Output:
<socket.socket fd=876, family=AddressFamily.AF_INET, type=SocketKind.SOCK_STREAM, proto=0>
In the case of the custom module, we can fix the error similarly. Here, we have called the method infomodule()
of the module infomodule
.
Example Code:
import infomodule
print(infomodule.infomodule())
Output:
meeting at 10:00 am.
Import the Method/Class From the Module
Another way to fix this error is to import the class from the module and make its object.
Here, we have imported the class socket
from the socket
module and made its object.
Example Code:
# Python 3.x
from socket import socket
socket()
Output:
<socket.socket fd=876, family=AddressFamily.AF_INET, type=SocketKind.SOCK_STREAM, proto=0>
We will also follow the same procedure in the case of custom modules. Here, we imported the method from the module and called it.
Example Code:
# Python 3.x
from infomodule import infomodule
print(infomodule())
Output:
meeting at 10:00 am.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python