How to Fix Python TypeError: 'DataFrame' Object Is Not Callable
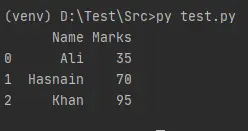
We will introduce how to call data from a DataFrame based on a query in Python. We will also introduce how to resolve the TypeError: 'DataFrame' object is not callable
in Python with examples.
TypeError: 'DataFrame' object is not callable
in Python
DataFrames are the object of Pandas, two dimensional labeled data structure with columns. DataFrames are the same as Spreadsheets and SQL tables used to store data.
While working on spreadsheets or scraping data from websites and saving them in spreadsheets, we often need to use data frames to organize the data correctly for users and us to understand and use later.
Let’s understand how we can create a data frame from multiple arrays in Python. We will create arrays containing students’ data, then utilize them in a data frame and save them in a spreadsheet.
The arrays are shown below.
Code:
# python
import pandas as pd
name = ["Ali", "Hasnain", "Khan"]
marks = ["35", "70", "95"]
data = {"Name": name, "Marks": marks}
df = pd.DataFrame(data)
print(df)
Output:
Now let’s add another column of Result
in which we will add whether a student passed or failed, as shown below.
Code:
# python
import pandas as pd
name = ["Ali", "Hasnain", "Khan"]
marks = ["35", "70", "95"]
result = ["Fail", "Pass", "Pass"]
data = {"Name": name, "Marks": marks, "Result": result}
df = pd.DataFrame(data)
print(df)
Output:
Now, if we have a data frame the same as the one we created but with a large amount of data, we want to extract the students who fail or pass using Boolean indexing. We will replace the last line with Boolean indexing, as shown below.
# python
print(df[df.Result == "Pass"])
The result of the above change will be shown below.
Output:
Many people need help getting the results from the data frame based on Boolean indexing. It is very simple to use.
We only need to create a new data frame and use []
brackets to mention the Boolean indexing based on which we want to get the results.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python