How to Fix Bytes-Like Object Is Required Not STR Error in Python
- Understanding the Error
- Method 1: Encoding Strings to Bytes
- Method 2: Decoding Bytes to Strings
- Method 3: Using the Correct Data Type in Functions
- Conclusion
- FAQ
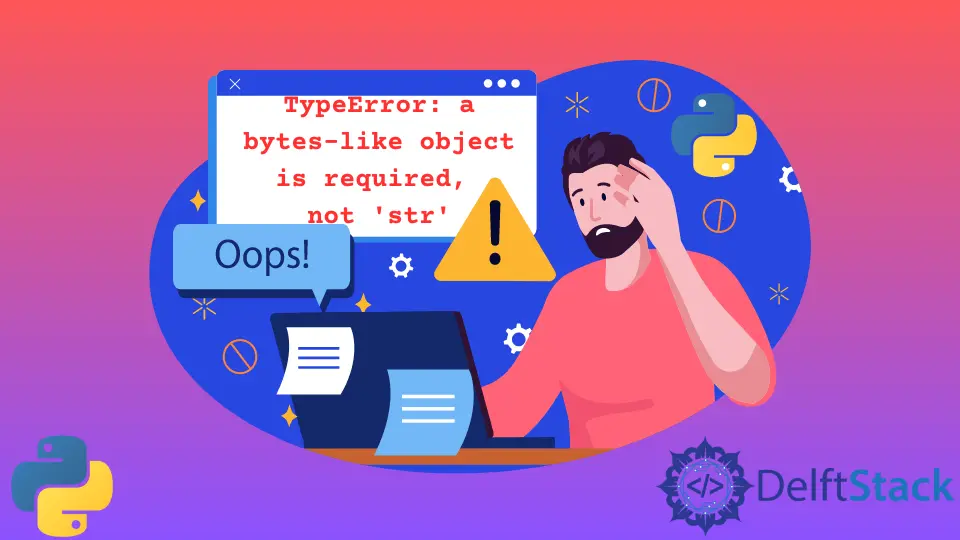
When working with Python, developers often encounter various errors that can be frustrating, especially when they seem cryptic. One common issue is the “bytes-like object is required, not str” error. This error typically occurs when you’re trying to perform operations that expect a bytes object but receive a string instead.
In this tutorial, we will delve into the reasons behind this error, its implications, and how to effectively resolve it. Whether you’re a novice or an experienced programmer, understanding this error can enhance your coding skills and improve your debugging process.
Understanding the Error
Before we jump into the solutions, let’s clarify what this error means. Python has two primary types for text and binary data: str
for strings and bytes
for binary data. When a function or method expects a bytes
object and receives a str
instead, Python raises this error. This often happens when dealing with file operations, network communications, or any situation where data encoding and decoding are involved.
Method 1: Encoding Strings to Bytes
One of the simplest ways to fix the “bytes-like object is required, not str” error is to convert your string into a bytes object. This is done using the encode()
method. The encode()
method converts a string into a bytes object by specifying the desired encoding, typically UTF-8.
Here’s how you can do it:
my_string = "Hello, World!"
my_bytes = my_string.encode('utf-8')
print(my_bytes)
Output:
b'Hello, World!'
When you run this code, the string “Hello, World!” is converted into a bytes object. The b
prefix indicates that the output is in bytes format. This conversion is essential when you are interacting with functions or APIs that require bytes input, such as writing to a binary file or sending data over a network. By encoding your strings, you ensure compatibility with these operations, thus avoiding the error.
Method 2: Decoding Bytes to Strings
Conversely, if you’re working with bytes data and need to convert it back to a string, you can use the decode()
method. This method transforms a bytes object into a string, allowing you to manipulate or display the data as needed.
Here’s a quick example:
my_bytes = b'Hello, World!'
my_string = my_bytes.decode('utf-8')
print(my_string)
Output:
Hello, World!
In this example, the bytes object is successfully converted back to a string using the decode()
method. It’s crucial to use the same encoding that was applied during the encoding process to avoid any errors. In most cases, UTF-8 is the standard encoding used, but other encodings can be specified as needed. This method is particularly useful when reading binary data from files or streams where the data is originally in bytes format.
Method 3: Using the Correct Data Type in Functions
Another effective way to resolve the “bytes-like object is required, not str” error is to ensure that you’re passing the correct data type to functions. If a function is designed to work with bytes, make sure that you are not unintentionally passing a string.
For example, consider a scenario where you’re writing data to a binary file. If you pass a string instead of bytes, you’ll encounter this error. Here’s how you can correctly write data to a binary file:
data = "This is a test."
with open("test.bin", "wb") as file:
file.write(data.encode('utf-8'))
Output:
None
In this case, the open()
function is used to create a binary file (test.bin
) in write mode. The write()
method requires bytes, so we encode the string before writing. By ensuring that the correct data type is used, you can prevent the error from occurring in the first place. This practice not only helps in avoiding errors but also leads to cleaner and more efficient code.
Conclusion
The “bytes-like object is required, not str” error can be a common stumbling block for Python developers, but with a clear understanding of how to handle strings and bytes, it can be easily resolved. By utilizing the encode()
and decode()
methods appropriately and ensuring you’re using the correct data types in your functions, you can effectively manage this error and enhance your coding experience. Remember, the key to successful programming lies in understanding the data types you’re working with and how they interact within your code.
FAQ
-
What causes the bytes-like object is required not str error?
This error occurs when a function expects a bytes object but receives a string instead. -
How can I convert a string to bytes in Python?
Use theencode()
method on the string, specifying the desired encoding, like UTF-8. -
Can I convert bytes back to a string?
Yes, you can use thedecode()
method on the bytes object to convert it back to a string.
-
What is the difference between str and bytes in Python?
str
is used for text data, whilebytes
represents binary data. -
How can I avoid this error in my code?
Always ensure that you’re using the correct data types when passing arguments to functions, particularly when dealing with file operations or network communications.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python