How to Truncate Float in Python
-
Use the
round()
Function to Truncate a Float in Python -
Use the
int()
Function to Truncate a Float in Python -
Use the
str()
Function to Truncate a Float in Python -
Use the
math.trunc()
Function to Truncate a Float in Python -
Truncating Floats with
math.trunc()
-
Use String Formatting (
f-strings
) to Truncate a Float in Python -
Truncating Floats with
f-strings
- Truncation with Rounding
- Customized Formatting
- Conclusion
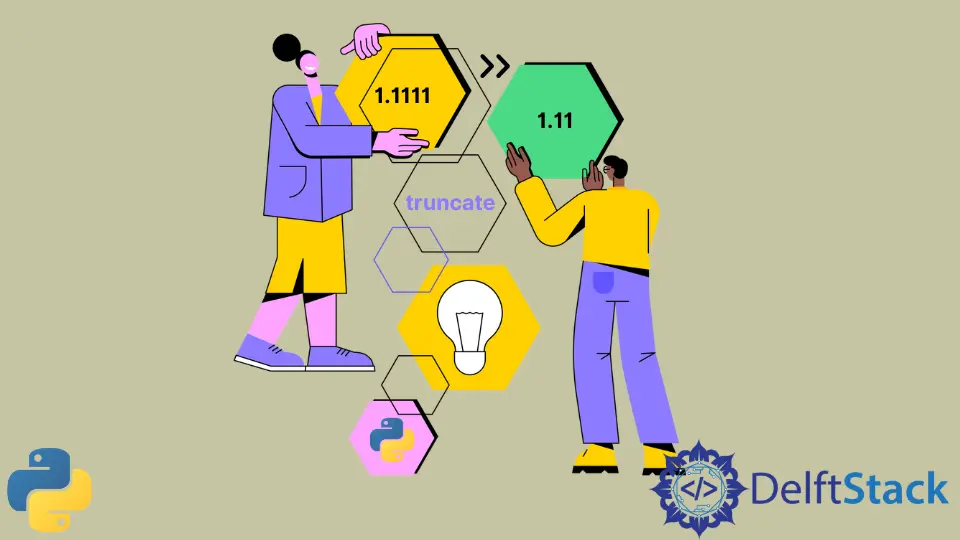
Floating-point numbers in Python can sometimes lead to precision issues.
To address this, you should truncate a float, which means removing the digits after a certain point. We can truncate a float by either dropping the remaining digits or rounding it.
The float truncation is used to make the calculation easier, and Python offers several methods to truncate floats, each with its own set of advantages and use cases. This tutorial will explain these methods, so let’s get started.
Use the round()
Function to Truncate a Float in Python
The round(number, n)
function takes a number as input and rounds it to n
decimal places. It takes two arguments:
- The number you want to round.
- The number of decimal places to round to.
Here’s the basic syntax of the round()
function:
rounded_number = round(number, ndigits)
number
: This is the floating-point number you want to round.ndigits
: This is the number of decimal places to round to. If omitted, it defaults to 0, which rounds the number to the nearest integer.
In case we want to truncate the float by rounding it, we can do so by using the round()
function in Python. The below example code demonstrates how to use the round()
function to truncate a float.
# Truncating to the nearest integer
number = 5.67
truncated_number = round(number)
print(truncated_number)
Output:
6
In the first example, we round the float 5.67
to the nearest integer, which is 6
.
# Truncating a negative number
negative_number = -3.45
truncated_negative = round(negative_number)
print(truncated_negative)
Output:
-3
In the second example, we round the negative float -3.45
to the nearest integer, which is -3
.
Truncating to a Specific Number of Decimal Places
Truncating a float to a specific number of decimal places involves specifying the ndigits
argument in the round()
function. Here’s how you can do it:
# Truncate to 2 decimal places
number = 3.14159
truncated_to_2_decimals = round(number, 2)
print(truncated_to_2_decimals)
Output:
3.14
In the first example, we truncate the float 3.14159
to 2 decimal places, resulting in 3.14
.
# Truncate to 3 decimal places
number = 2.71828
truncated_to_3_decimals = round(number, 3)
print(truncated_to_3_decimals)
Output:
2.718
In the second example, we truncate the float 2.71828
to 3 decimal places, yielding 2.718
.
Handling Half-Up Rounding
By default, the round()
function uses half-up
rounding. That means if the number is exactly halfway between two rounded values, it rounds to the nearest even number. This behavior is known as banker's rounding
. For example:
# Half-up rounding
number = 0.5
rounded_number = round(number)
print(rounded_number)
Output:
0
In this example, 0.5
is rounded to 0
because it is halfway between 0
and 1
, and the round()
function follows the half-up rounding rule.
Use the int()
Function to Truncate a Float in Python
The int()
function in Python is primarily used to convert other data types, such as strings or floating-point numbers, into integer values. When applied to a floating-point number, it effectively truncates the decimal part, keeping only the integer component. The basic syntax of the int()
function is as follows.
integer_value = int(float_number)
float_number
: This is the floating-point number you want to truncate.
Truncating a float using the int()
function is a simple and efficient way to remove the decimal portion and obtain an integer value. Let’s see some examples:
# Truncate to an integer
float_number = 5.67
truncated_integer = int(float_number)
print(truncated_integer)
Output:
5
In this example, we use the int()
function to truncate the float 5.67
to an integer value, resulting in 5
.
# Truncate a negative number
negative_float = -3.45
truncated_negative_integer = int(negative_float)
print(truncated_negative_integer)
Output:
-3
In the second example, we truncate the negative float -3.45
, and the int()
function correctly removes the decimal portion, resulting in -3
.
When using the int()
function to truncate a float, it’s important to note that it performs a simple truncation, effectively removing the decimal part of the number.
Use the str()
Function to Truncate a Float in Python
We can also truncate a float by dropping the remaining digits after n
decimal places by first converting it into a string.
Once we convert the float into a string, we can look for the decimal point .
in the string; if it exists, we can keep the n
digits after it or complete the float if it has less than n
digits after the decimal point.
The below example code demonstrates how to use the str()
function to truncate a float in Python.
def truncate(num, n):
temp = str(num)
for x in range(len(temp)):
if temp[x] == ".":
try:
return float(temp[: x + n + 1])
except:
return float(temp)
return float(temp)
print(truncate(1923334567124, 4))
print(truncate(2345.1252342, 4))
print(truncate(192.67, 4))
This code’s truncate
function aims to truncate a given number to a specified number of decimal places (n
).
It does this by converting the number to a string, searching for the decimal point, and then returning a new float with the desired precision. If the decimal point is not found, it returns the original number as a float.
Output:
1923334567124.0
2345.1252
192.67
Use the math.trunc()
Function to Truncate a Float in Python
The math.trunc()
function is part of Python’s built-in math
module, which provides a comprehensive set of mathematical operations and functions.
Specifically, math.trunc()
is designed to truncate a floating-point number to an integer value by discarding the decimal part. This function is particularly useful when you want to obtain the integer component of a float without rounding to the nearest integer.
The basic syntax of the math.trunc()
function is as follows:
import math
truncated_integer = math.trunc(float_number)
float_number
: This is the floating-point number you want to truncate.
Truncating Floats with math.trunc()
Truncating a float using the math.trunc()
function is straightforward and efficient. It allows you to remove the decimal portion while keeping the integer component intact. Let’s explore some examples:
import math
# Truncate to an integer
float_number = 5.67
truncated_integer = math.trunc(float_number)
print(truncated_integer)
Output:
5
In this example, we use the math.trunc()
function to truncate the float 5.67
to an integer value, resulting in 5
.
# Truncate a negative number
negative_float = -3.45
truncated_negative_integer = math.trunc(negative_float)
print(truncated_negative_integer)
Output:
-3
In the second example, we truncate the negative float -3.45
, and the math.trunc()
function correctly removes the decimal portion, resulting in -3
.
Use String Formatting (f-strings
) to Truncate a Float in Python
f-strings
, also known as formatted string literals
, provide a concise and readable way to embed expressions inside string literals, using curly braces {}
to enclose expressions. Introduced in Python 3.6 and later versions, f-strings
are a powerful tool for string formatting, offering both simplicity and flexibility.
The basic syntax of an f-string
is as follows:
formatted_string = f"Text and {expression} within the string."
expression
: This is the Python expression you want to include within the string.
Truncating Floats with f-strings
To truncate a float number using f-strings
, you can incorporate the float within the string and specify the desired number of decimal places using format specifiers. The format specifier consists of a colon :
followed by various format options. To truncate a float, you can use the :.nf
format specifier, where n
is the number of decimal places you want to keep.
Let’s see some examples:
# Truncate to 2 decimal places
float_number = 3.14159
truncated_string = f"Truncated value: {float_number:.2f}"
print(truncated_string)
Output:
Truncated value: 3.14
In this example, we use the f-string
to truncate the float 3.14159
to 2 decimal places, resulting in the string “3.14.”
# Truncate to 3 decimal places
float_number = 2.71828
truncated_string = f"Truncated value: {float_number:.3f}"
print(truncated_string)
Output:
Truncated value: 2.718
In the second example, we truncate the float 2.71828
to 3 decimal places, yielding the string "2.718"
.
Truncate A Float to Integer
To truncate a float to an integer using f-strings
, you can simply use the :.0f
format specifier, which specifies zero decimal places. Here’s an example:
# Truncate to an integer using f-strings
float_number = 8.99
truncated_string = f"Truncated to integer: {float_number:.0f}"
print(truncated_string)
Output:
Truncated to integer: 9
In this example, we use f-strings
to truncate the float 8.99
to an integer value, resulting in the string “9.” The :.0f
format specifier ensures that no decimal places are displayed, effectively truncating the float to the nearest integer.
Truncation with Rounding
If you want to combine truncation with rounding using f-strings
, you can use the round()
function within the expression to round the float before formatting it. For example:
value = 5.6789
rounded_and_truncated = f"Rounded and truncated: {round(value, 2):.2f}"
print(rounded_and_truncated)
Output:
Rounded and truncated: 5.68
In this case, we first round the float 5.6789
to 2 decimal places using round()
and then truncate it to the same precision using the f-string
. The result is 5.68
.
Customized Formatting
f-strings
offer extensive flexibility in formatting float numbers. You can include additional formatting options, such as specifying the minimum width of the field, aligning the value within the field, and adding leading zeros, among others. Here’s an example:
number = 7.5
formatted_number = f"Formatted: {number:07.2f}"
print(formatted_number)
Output:
Formatted: 007.50
In this example, we format the float 7.5
with a minimum field width of 7 characters, including two decimal places. The result is "007.50"
.
Conclusion
In this guide, we’ve explored various methods to truncate a float in Python. Each approach offers unique advantages and can be applied based on specific requirements.
Whether you need a basic truncation or a more complex manipulation of the float’s representation, these techniques provide you with the flexibility to handle precision issues effectively.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn