Ternary Conditional Operator in Python
- Use the Ternary Operator in Python 2.5 and Above
- the Ternary Operator in Python Using Tuple
-
the Ternary Operator Using
lambda
for Versions Before 2.5 in Python
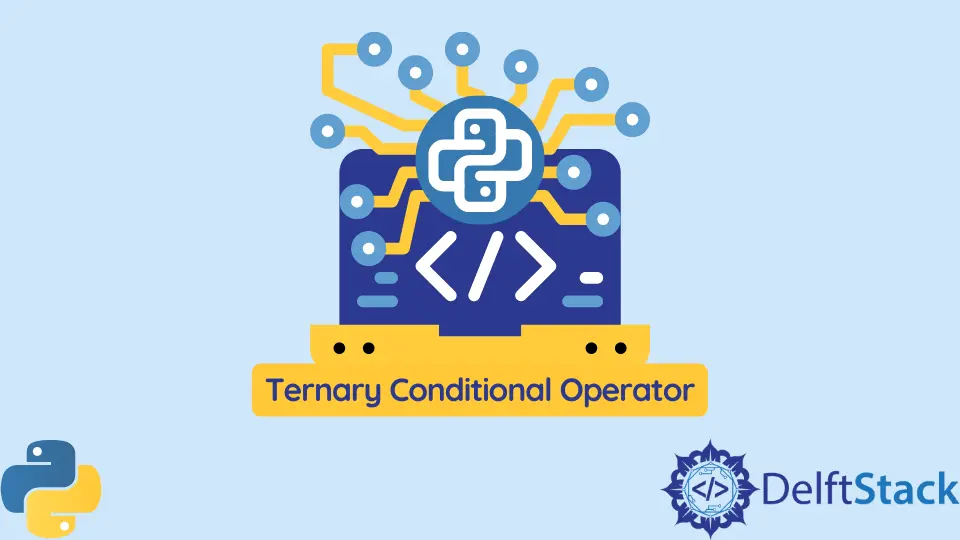
This tutorial will define different methods to use the ternary operator in Python. There is a different way of using the ternary operator in Python as compared to other programming languages. There are various ways to use ternary operators as per compatibility with the Python version. The tutorial will provide example codes to explain how to use the ternary operator in different Python versions.
Use the Ternary Operator in Python 2.5 and Above
The ternary conditional operator was added in Python 2.5. The ternary operator is defined as the operator which takes three operands. In this method, first, the given condition is evaluated, then one of the values is evaluated and sent back based on the boolean operator. It first takes the expression, and then comes the condition again, followed by the else
part of the expression.
The syntax of the ternary operator in Python is as below.
value_if if condition else value_else
As the ternary operator first evaluates the condition
, it allows short-circuiting, and only one of the two expressions will be evaluated. If condition
is true, the first expression value_if
is evaluated otherwise second expression value_else
is evaluated.
The example code below demonstrates how to use the ternary operators in Python.
a = 2
b = 0
1 if a > b else 0
Output:
1
The ternary conditional operator’s output can be assigned to a variable, as shown in the example code below.
a = 2
b = 0
temp = a if a > b else b
print(temp)
Output:
2
the Ternary Operator in Python Using Tuple
The ternary operator in Python can be used by using tuples. It takes the expressions to be evaluated and a Boolean conditional statement. The expression to be returned depends on the boolean condition. If the condition is true, the first value is returned, and if the expression is false, the second value is returned. However, in any case, this method will evaluate everything instead of only the winning expression.
An example code is given below to demonstrate how this ternary operator can be used in Python using tuple.
a, b = 12, 43
temp = (a * 2, b / 2)[a < b]
print(temp)
Output:
21.5
the Ternary Operator Using lambda
for Versions Before 2.5 in Python
For versions prior to Python 2.5, the ternary operators can be used with lambda
. This method takes the values to be returned and a Boolean expression. This method follows a lazy evaluation technique in which the evaluation process is delayed until its value is required. It is a better approach than the tuple method in this sense. The expression in this method is given as (falseValue, trueValue)[Test]()
. If the test condition is evaluated as True
the trueValue
will be printed; otherwise, falseValue
gets printed.
An example code is given below to describe how the ternary operator can be used with lambda
in Python.
val1, val2 = 12, 43
output = (lambda: val2, lambda: val1)[val1 > val2]()
print(output)
Output:
43
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn