Series Summation in Python
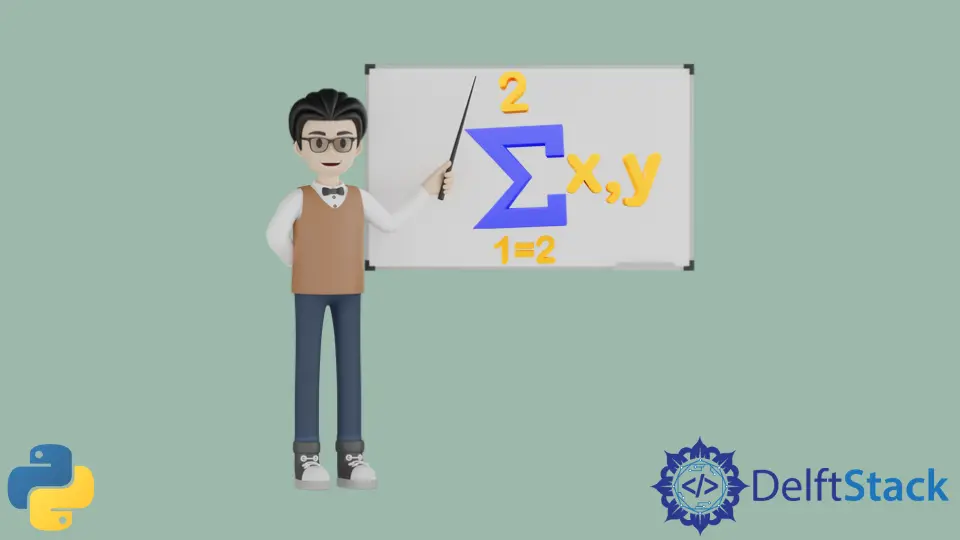
When it comes to working with numerical data in Python, summing up a series is a common task. Whether you’re analyzing data, performing calculations, or simply trying to understand a sequence of numbers, Python offers straightforward methods to achieve this.
In this article, we will explore two primary techniques for summing a series: the simple for loop method and the built-in sum()
function. Each method has its advantages and use cases, making it essential to understand how they work. By the end of this article, you will be equipped with the knowledge to choose the best approach for your specific needs.
Method 1: Using a for
Loop
The for loop is one of the most fundamental constructs in Python. It allows you to iterate over a sequence and perform operations on each element. To sum a series using a for loop, you can initialize a variable to hold the total and then iterate through each number in the series, adding it to the total. Here’s a simple example:
series = [1, 2, 3, 4, 5]
total = 0
for number in series:
total += number
print(total)
Output:
15
In this code snippet, we first define a list called series
containing the numbers we want to sum. We then initialize a variable total
to zero. The for loop iterates through each number in the series
, adding it to total
. Finally, we print the result. The advantage of using a for loop is its clarity; it is straightforward and easy to understand, especially for beginners. However, this method may be less efficient for larger datasets, as it requires multiple iterations.
Method 2: Using the sum() Function
Python’s built-in sum()
function provides a more concise way to sum a series. This function takes an iterable, such as a list or tuple, and returns the total sum of its elements. This method is not only cleaner but also more efficient for larger datasets. Here’s how to use the sum()
function:
series = [1, 2, 3, 4, 5]
total = sum(series)
print(total)
Output:
15
In this example, we create the same list series
as before. Instead of using a for loop, we call the sum()
function and pass the series
as an argument. The function computes the sum and returns it, which we then print. The beauty of the sum()
function lies in its simplicity and efficiency. It abstracts away the looping mechanism, allowing you to focus on what you want to achieve rather than how to achieve it. This can lead to cleaner, more maintainable code, especially in larger projects.
Conclusion
Summing a series in Python can be accomplished through various methods, but the for loop and the built-in sum()
function stand out as the most common. The for loop is excellent for those who appreciate clarity and control, while the sum()
function offers a more efficient and concise approach. Depending on your specific needs, you can choose the method that best suits your coding style and the complexity of your data. With these tools in your arsenal, you can easily tackle numerical data in Python.
FAQ
-
What is the difference between using a for loop and the sum() function in Python?
The for loop provides more control and clarity, while the sum() function is more concise and efficient for summing elements in a sequence. -
Can I sum a series of numbers stored in a different data structure, like a tuple?
Yes, the sum() function can work with any iterable, including lists, tuples, and sets. -
Is there a performance difference between the two methods for very large datasets?
Yes, the sum() function is generally more efficient for larger datasets as it is optimized in Python’s implementation. -
Can I sum only specific elements of a series?
Yes, you can use a for loop with a condition to sum only specific elements or use list comprehensions to filter the series before summing. -
What are some other built-in functions in Python for numerical operations?
Python offers several built-in functions for numerical operations, including min(), max(), and statistics like mean() and median() from the statistics module.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn