How to Fix STR Object Does Not Support Item Assignment Error in Python
- Understanding the Error
- Method 1: Create a New String
- Method 2: Convert to a List
- Method 3: Use String Methods
- Conclusion
- FAQ
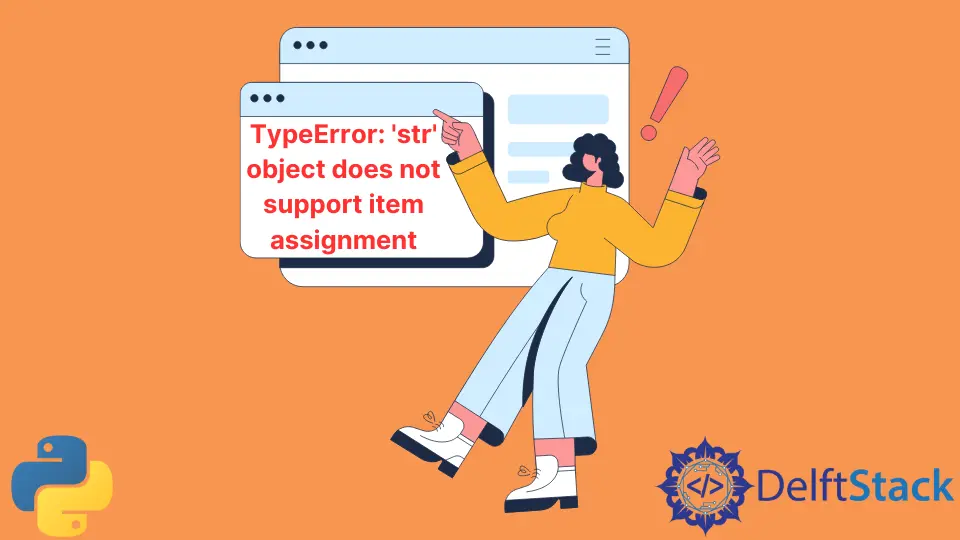
When working with Python, you may encounter the “str object does not support item assignment” error. This issue arises when you attempt to modify a string directly, which is immutable in Python. Understanding why this error occurs and how to fix it is essential for any Python developer.
In this article, we will explore various methods to resolve this error, including creating new strings, using list conversions, and leveraging string methods. By the end, you will have a solid grasp of how to handle this common problem effectively. Let’s dive in!
Understanding the Error
Before we jump into the solutions, it’s crucial to understand what causes the “str object does not support item assignment” error. In Python, strings are immutable, meaning once a string is created, it cannot be changed. When you try to assign a new value to a specific index of a string, Python raises a TypeError because it does not allow changes to the existing string.
For example, consider the following code snippet:
my_string = "Hello"
my_string[0] = "h"
Output:
TypeError: 'str' object does not support item assignment
In this case, you are trying to change the first character of the string “Hello” to “h”, which leads to the error. Now, let’s explore methods to fix this issue.
Method 1: Create a New String
One of the simplest ways to fix the “str object does not support item assignment” error is by creating a new string. Instead of trying to modify the existing string, you can concatenate parts of the string or use slicing to create a new version.
Here’s how you can do that:
my_string = "Hello"
new_string = "h" + my_string[1:]
Output:
hallo
In this code, we create a new string called new_string
. We take the first character “h” and concatenate it with the rest of the original string (from index 1 onward). This approach effectively changes the first character while keeping the rest of the string intact.
By using this method, you avoid the immutability issue entirely. Instead of modifying the original string, you simply create a new one that reflects the desired changes. This practice is common in Python, where string manipulation often involves constructing new strings rather than altering existing ones.
Method 2: Convert to a List
Another effective method to resolve the error is by converting the string into a list. Lists in Python are mutable, which means you can modify their elements directly. Once you’ve made the required changes, you can convert the list back into a string.
Here’s how you can achieve this:
my_string = "Hello"
string_list = list(my_string)
string_list[0] = "h"
new_string = ''.join(string_list)
Output:
hallo
In this example, we first convert the string my_string
into a list called string_list
. This allows us to modify the first element of the list directly. After changing the first character to “h”, we then use the join()
method to combine the list back into a single string, resulting in new_string
.
This method is particularly useful when you need to make multiple modifications to a string or when the string is long. Converting to a list can simplify the process of making changes, as lists provide more flexibility compared to strings.
Method 3: Use String Methods
Python also offers various string methods that can help you achieve the desired modifications without directly assigning values to string indices. Methods like replace()
, upper()
, and lower()
can be very handy.
For instance, if you want to change a specific character in a string, you can use the replace()
method:
my_string = "Hello"
new_string = my_string.replace("H", "h", 1)
Output:
hallo
In this code, we utilize the replace()
method to replace the first occurrence of “H” with “h”. The third argument specifies that only the first occurrence should be replaced. This method is not only straightforward but also quite powerful, as it allows you to make changes without worrying about the immutability of strings.
Using string methods can lead to cleaner and more readable code. It allows you to express your intent clearly and can often reduce the amount of code you need to write.
Conclusion
The “str object does not support item assignment” error is a common pitfall for Python developers, especially those new to the language. Understanding that strings are immutable is crucial for effective coding. By employing techniques such as creating new strings, converting to lists, or using string methods, you can easily work around this limitation. Each method has its advantages, so choose the one that best fits your specific use case. With these strategies in your toolkit, you will navigate string manipulation in Python with confidence.
FAQ
-
What causes the “str object does not support item assignment” error?
The error occurs because strings in Python are immutable, meaning you cannot change their content directly. -
How can I modify a specific character in a string?
You can create a new string that reflects the desired changes by concatenating or using thereplace()
method. -
Is there a way to convert a string to a list in Python?
Yes, you can use thelist()
function to convert a string into a list of characters, allowing for direct modifications. -
Can I use string methods to change parts of a string?
Absolutely! Methods likereplace()
,upper()
, andlower()
provide a way to modify strings without direct assignment. -
What is the best method to fix the error?
The best method depends on your specific needs. Creating a new string is straightforward, while converting to a list may be more efficient for multiple changes.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python