How to Manage Segmentation Fault in Python
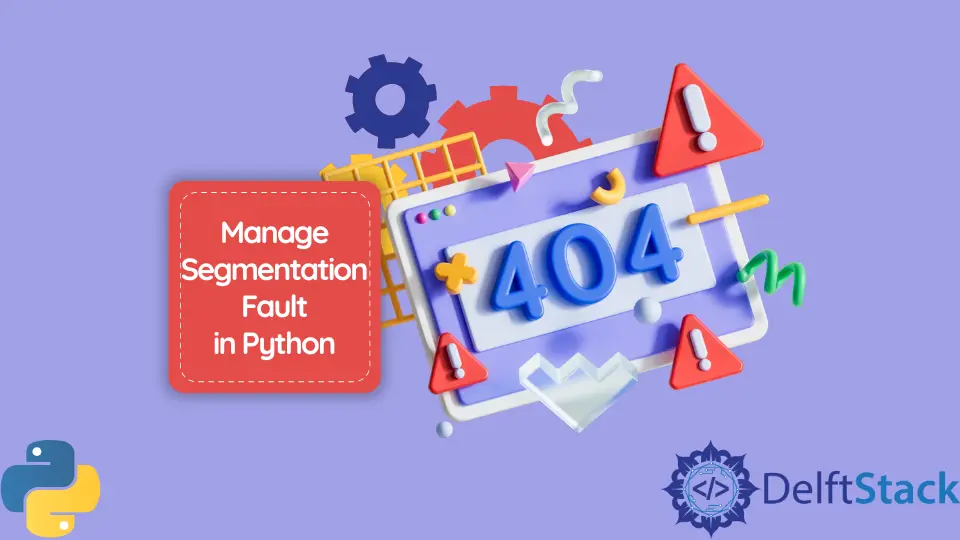
Developing especially complex applications can lead to crazy technical situations such as segmentation faults. This situation can happen due to different factors, and tracing the issue with your code is important.
Some of the biggest causes of segmentation faults are illegal memory location (using your code to access memory that you don’t have access to), fetching huge datasets, infinite recursion, etc. In this article, we will show you how to manage segmentation faults in Python.
Use settrace
to Manage Segmentation Fault in Python
When faced with a segmentation fault
error, it is important to know that you will have to rewrite your code.
Knowing the part to rewrite is a good starting point. That’s where sys.trace
comes in.
The sys
module allows us to check some variables and interact with the interpreter, and the settrace
function allows us to trace the program execution and serves a Python source code debugger.
So with a case of segmentation fault
, we can easily trace what calls are made and when everything happens.
The trace functions are important to the whole process, taking three arguments: frame
, event
, and arg
. The frame
takes the current stack frame, the event
takes a string, and the arg
takes shape depending on the event we parse.
So, within your code, you can import the sys
module, create your trace
function, and pass the trace
function through the settrace
method, which makes for a higher-order function. Then, you place your code that creates a segmentation fault
.
Let’s create a trace for a test()
function that prints two lines of code.
import sys
def trace(frame, event, arg):
print("%s, %s:%d" % (event, frame.f_code.co_filename, frame.f_lineno))
return trace
def test():
print("Line 8")
print("Line 9")
sys.settrace(trace)
test()
Output:
call, c:\Users\akinl\Documents\Python\segment.py:7
line, c:\Users\akinl\Documents\Python\segment.py:8
call, C:\Python310\lib\encodings\cp1252.py:18
line, C:\Python310\lib\encodings\cp1252.py:19
return, C:\Python310\lib\encodings\cp1252.py:19
Line 8call, C:\Python310\lib\encodings\cp1252.py:18
line, C:\Python310\lib\encodings\cp1252.py:19
return, C:\Python310\lib\encodings\cp1252.py:19
line, c:\Users\akinl\Documents\Python\segment.py:9
call, C:\Python310\lib\encodings\cp1252.py:18
line, C:\Python310\lib\encodings\cp1252.py:19
return, C:\Python310\lib\encodings\cp1252.py:19
Line 9call, C:\Python310\lib\encodings\cp1252.py:18
line, C:\Python310\lib\encodings\cp1252.py:19
return, C:\Python310\lib\encodings\cp1252.py:19
return, c:\Users\akinl\Documents\Python\segment.py:9
You can see the event
occurring with each line, call
, line
, and return
. With these, you can track every action the Python interpreter is doing and the output it gives.
You can see the code outputs - Line 8call, C:\Python310\lib\encodings\cp1252.py:18
and Line 9call, C:\Python310\lib\encodings\cp1252.py:18
. Therefore, with a segmentation fault
, we can trace where the problems start and work our way from there.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python