How to Search a List of Dictionaries in Python
-
Use the
next()
Function to Search a List of Dictionaries in Python -
Search a List of Dictionaries With the
filter()
Function in Python - Use List Comprehension to Search a List of Dictionaries in Python
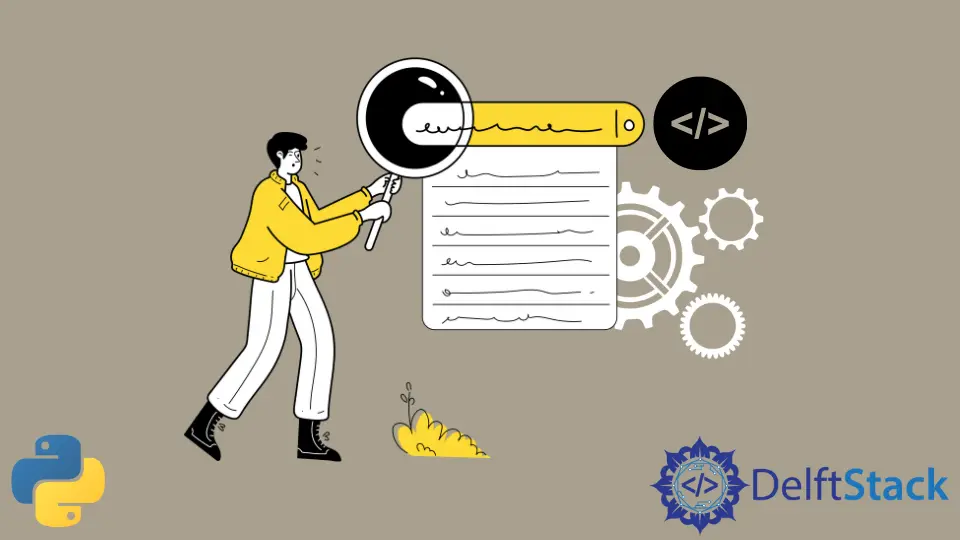
This tutorial will introduce the methods you can use to search a list of dictionaries in Python.
Use the next()
Function to Search a List of Dictionaries in Python
The next()
function can be utilized to provide the result as the next item in the given iterator. This method also requires the use of the for
loop to test the process against all the conditions.
The following code uses the next()
function to search a list of dictionaries in Python.
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print(next(x for x in lstdict if x["name"] == "Klaus"))
print(next(x for x in lstdict if x["name"] == "David"))
Output:
{'name': 'Klaus', 'age': 32}
Traceback (most recent call last):
File "<string>", line 8, in <module>
StopIteration
This method is successfully implemented when we search for a name that already exists in the list of dictionaries. Still, it gives a StopIteration
error when a name that doesn’t exist in the list of dictionaries is searched.
However, this problem can be easily treated in the code above. You simply tweak and provide a default with the use of a slightly different API.
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print(next((x for x in lstdict if x["name"] == "David"), None))
Output:
None
Rather than finding the item itself, we can also find the item’s index in a List of Dictionaries. To implement this, we can use the enumerate()
function.
The following code uses the next()
function and the enumerate()
function to search and find the item’s index.
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print(next((i for i, x in enumerate(lstdict) if x["name"] == "Kol"), None))
Output:
2
Search a List of Dictionaries With the filter()
Function in Python
The filter(function, sequence)
function is used to compare the sequence
with the function
in Python. It checks each element in the sequence to be true or not according to the function. We can easily search a list of dictionaries for an item by using the filter()
function with a lambda
function. In Python3, the filter()
function returns an object of the filter
class. We can convert that object into a list with the list()
function.
The following code example shows us how we can search a list of dictionaries for a specific element with the filter()
and lambda
functions.
listOfDicts = [
{"name": "Tommy", "age": 20},
{"name": "Markus", "age": 25},
{"name": "Pamela", "age": 27},
{"name": "Richard", "age": 22},
]
list(filter(lambda item: item["name"] == "Richard", listOfDicts))
Output:
[{'age': 22, 'name': 'Richard'}]
We searched the list of dictionaries for the element where the name
key is equal to Richard
by using the filter()
function with a lambda
function. First, we initialized our list of dictionaries, listOfDicts
, and used the filter()
function to search the values that match the lambda
function lambda item: item['name'] == 'Richard'
in it. Finally, we used the list()
function to convert the results into a list.
Use List Comprehension to Search a List of Dictionaries in Python
List comprehension is a relatively shorter and very graceful way to create lists that are to be formed based on the given values of an already existing list.
We can use list comprehension to return a list that produces the results of the search of the list of dictionaries in Python.
The following code uses list comprehension to search a list of dictionaries in Python.
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print([x for x in lstdict if x["name"] == "Klaus"][0])
Output:
{'name': 'Klaus', 'age': 32}
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- How to Convert a Dictionary to a List in Python
- How to Get All the Files of a Directory
- How to Find Maximum Value in Python Dictionary
- How to Sort a Python Dictionary by Value
- How to Merge Two Dictionaries in Python 2 and 3
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python