在 Python 中搜尋字典列表
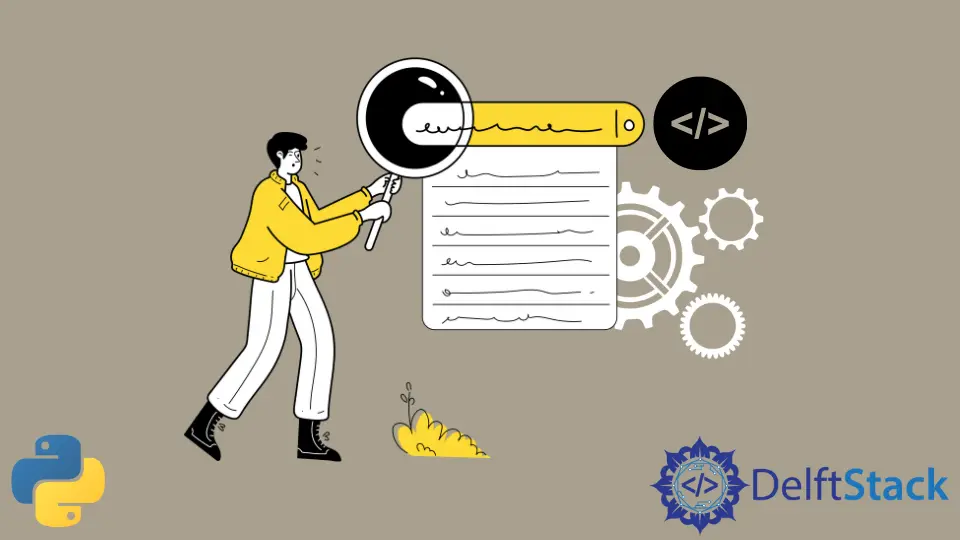
本教程將介紹可用於在 Python 中搜尋字典列表的方法。
在 Python 中使用 next()
函式搜尋字典列表
next()
函式可用於提供結果作為給定迭代器中的下一項。此方法還需要使用 for
迴圈來針對所有條件測試流程。
以下程式碼使用 next()
函式在 Python 中搜尋字典列表。
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print(next(x for x in lstdict if x["name"] == "Klaus"))
print(next(x for x in lstdict if x["name"] == "David"))
輸出:
{'name': 'Klaus', 'age': 32}
Traceback (most recent call last):
File "<string>", line 8, in <module>
StopIteration
當我們搜尋字典列表中已經存在的名稱時,該方法成功實現。儘管如此,當搜尋字典列表中不存在的名稱時,它會給出 StopIteration
錯誤。
但是,在上面的程式碼中可以很容易地處理這個問題。你只需使用稍微不同的 API 來調整並提供預設值。
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print(next((x for x in lstdict if x["name"] == "David"), None))
輸出:
None
除了查詢專案本身,我們還可以在字典列表中查詢專案的索引。為了實現這一點,我們可以使用 enumerate()
函式。
以下程式碼使用 next()
函式和 enumerate()
函式來搜尋和查詢專案的索引。
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print(next((i for i, x in enumerate(lstdict) if x["name"] == "Kol"), None))
輸出:
2
在 Python 中使用 filter()
函式搜尋字典列表
filter(function, sequence)
function 用於將 sequence
與 Python 中的 function
進行比較。它根據函式檢查序列中的每個元素是否為真。通過使用 filter()
函式和 lambda
函式,我們可以輕鬆地在字典列表中搜尋一個專案。在 Python3 中,filter()
函式返回 filter
類的物件。我們可以使用 list()
函式將該物件轉換為列表。
下面的程式碼示例向我們展示瞭如何使用 filter()
和 lambda
函式在字典列表中搜尋特定元素。
listOfDicts = [
{"name": "Tommy", "age": 20},
{"name": "Markus", "age": 25},
{"name": "Pamela", "age": 27},
{"name": "Richard", "age": 22},
]
list(filter(lambda item: item["name"] == "Richard", listOfDicts))
輸出:
[{'age': 22, 'name': 'Richard'}]
我們使用 filter()
函式和 lambda
函式在字典列表中搜尋 name
鍵等於 Richard
的元素。首先,我們初始化了字典列表 listOfDicts
,並使用 filter()
函式搜尋與 lambda
函式 lambda item: item['name'] == 'Richard'
匹配的值它。最後,我們使用 list()
函式將結果轉換為列表。
在 Python 中使用列表推導搜尋字典列表
列表推導式是一種相對較短且非常優雅的方式來建立基於現有列表的給定值形成的列表。
我們可以使用列表推導返回一個列表,該列表生成 Python 中字典列表的搜尋結果。
以下程式碼使用列表推導在 Python 中搜尋字典列表。
lstdict = [
{"name": "Klaus", "age": 32},
{"name": "Elijah", "age": 33},
{"name": "Kol", "age": 28},
{"name": "Stefan", "age": 8},
]
print([x for x in lstdict if x["name"] == "Klaus"][0])
輸出:
{'name': 'Klaus', 'age': 32}
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相關文章 - Python Dictionary
- 在 Python 中將字典轉換為列表
- Python 如何得到資料夾下的所有檔案
- 在 Python 字典中尋找最大值
- 如何按值對字典排序
- 如何在 Python 2 和 3 中合併兩個字典
- 如何從 Python 字典中刪除元素