How to Solve Reduce Is Not Defined in Python
- Understanding the Reduce Function
- Solution 1: Importing Reduce from functools
- Solution 2: Using Alternative Methods
- Conclusion
- FAQ
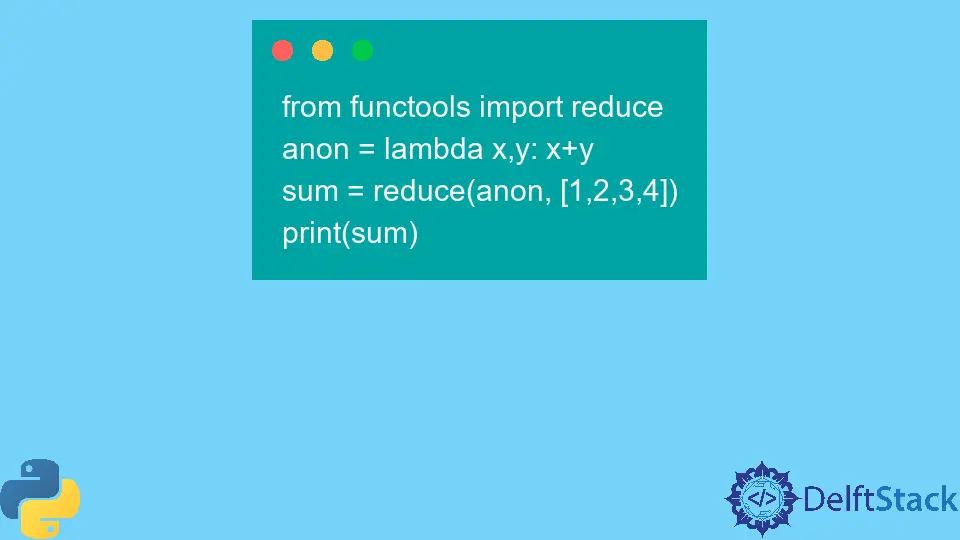
If you’ve encountered the “reduce is not defined” error in Python, you’re not alone. This common issue arises when trying to use the reduce()
function, which is not part of the built-in functions in Python 3 but was available in Python 2. The error can be frustrating, especially when you’re trying to streamline your code with functional programming techniques. Fortunately, this tutorial will guide you through the process of resolving this error effectively. We’ll explore the reasons behind this issue and provide you with clear, practical solutions to get you back on track. Let’s dive in!
Understanding the Reduce Function
Before we jump into solutions, it’s essential to understand what the reduce()
function does. The reduce()
function is used to apply a particular function cumulatively to the items of an iterable, reducing it to a single value. For example, if you want to calculate the product of all numbers in a list, reduce()
can help you achieve that in a concise manner.
However, in Python 3, reduce()
is not included in the global namespace; you need to import it from the functools
module. This is likely where the “reduce is not defined” error originates.
Solution 1: Importing Reduce from functools
The most straightforward solution to resolve the “reduce is not defined” error is to import the reduce()
function from the functools
module. This method is efficient and allows you to continue using reduce()
as intended.
Here’s how you can do it:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
result = reduce(lambda x, y: x * y, numbers)
print(result)
Output:
120
In this example, we first import reduce
from the functools
module. We then define a list of numbers and use reduce()
to multiply all the elements together. The lambda function takes two arguments, x
and y
, and returns their product. The final output is 120
, which is the product of all numbers in the list. By importing reduce
, you can leverage its functionality without encountering the error.
Solution 2: Using Alternative Methods
If you prefer not to use reduce()
, you can achieve similar results using alternative methods available in Python. One common approach is to use a simple loop or the built-in math.prod()
function, which is available in Python 3.8 and later.
Here’s how you can use a loop:
numbers = [1, 2, 3, 4, 5]
result = 1
for number in numbers:
result *= number
print(result)
Output:
120
In this code snippet, we initialize a variable result
to 1. We then loop through each number in the numbers
list, multiplying it with result
. This method effectively calculates the product without needing reduce()
.
Alternatively, if you’re using Python 3.8 or later, you can utilize the math.prod()
function:
import math
numbers = [1, 2, 3, 4, 5]
result = math.prod(numbers)
print(result)
Output:
120
The math.prod()
function simplifies the process, allowing you to compute the product of all elements in a list in a single line. This method is not only concise but also improves code readability. Choosing between these alternatives depends on your specific use case and preferences.
Conclusion
Encountering the “reduce is not defined” error in Python can be a stumbling block, but it’s easily fixable. By importing reduce
from the functools
module, you can restore its functionality and continue using it in your projects. Alternatively, you can opt for loops or the math.prod()
function as effective substitutes. Each method has its advantages, so choose the one that best fits your coding style and project requirements. With these solutions, you’ll be well-equipped to tackle similar issues in the future.
FAQ
-
What is the purpose of the reduce function in Python?
Thereduce
function applies a specified function cumulatively to the items of an iterable, reducing it to a single value. -
Why is reduce not defined in Python 3?
In Python 3,reduce
is not a built-in function; it must be imported from thefunctools
module.
-
Can I use reduce without importing it?
No, you need to importreduce
from thefunctools
module to use it in Python 3. -
What are some alternatives to using reduce?
Alternatives include using loops or themath.prod()
function for multiplication tasks. -
Is reduce available in Python 2?
Yes,reduce
is a built-in function in Python 2, which is why you may not encounter this error in that version.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python