How to Print Object's Attributes in Python
-
Print Attributes of an Object in Python Using
print
and Attribute Access -
Print Attributes of an Object in Python Using the
getattr
Function -
Print Attributes of an Object in Python Using the
__dict__
Attribute -
Print Attributes of an Object in Python Using the
dir()
Function in Python -
Print Attributes of an Object in Python Using the
vars()
Function in Python - Conclusion
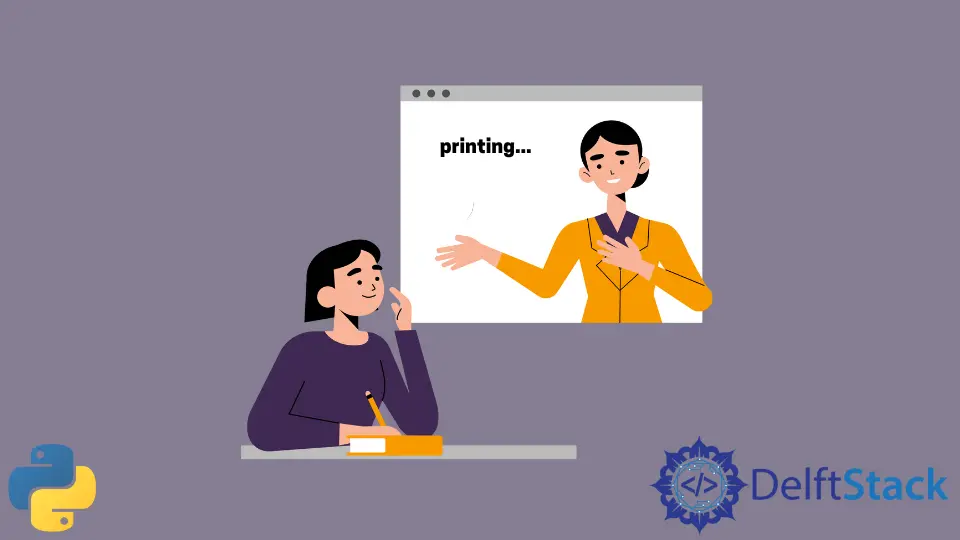
This tutorial will explain various methods to print the attributes of an object in Python.
An attribute in object-oriented programming is the property of a class or an instance. For example, a class named student
can have name
, roll no
, and marks
, as its attributes. Every instance of the class shares all the attributes of a class.
In this tutorial, we will look into how to get and print an object’s attributes in Python.
Let’s consider a simple class named Person
with a few attributes, such as name
, age
, and city
. We will use instances of this class to demonstrate the different ways to print object attributes.
class Person:
def __init__(self, name, age, city):
self.name = name
self.age = age
self.city = city
# Creating an instance of the Person class
person_instance = Person("John Doe", 30, "New York")
The class
keyword is used to define a new class named Person
. Inside the class, there is a special method called __init__
. This method is the constructor, which is automatically called when an object of the class is created.
The __init__
method takes four parameters: self
, name
, age
, and city
.
self
is a reference to the instance of the class itself. It is a convention to name it self
, but you could technically use any name. The other parameters (name
, age
, city
) are used to initialize the attributes of the class. self.name
, self.age
, and self.city
are attributes of the class, and they are used to store information about each instance of the Person
class.
Print Attributes of an Object in Python Using print
and Attribute Access
print()
is a built-in function in Python that is used to output text or other data to the console. It allows you to display information, variables, or results of computations during the execution of a Python script or program.
The print()
function takes one or more arguments and displays them on the standard output (usually the console).
In the first method, we will only need the print()
method to directly access the attribute name
using dot notation and print its value.
print(person_instance.name)
Output:
John Doe
In our variable person_instance
, we used the class Person
and declared the values on the attributes such as name
, age
, and city
. The John Doe
is printed as output because we only called the attribute named name
in the print
syntax.
The advantages of this method are the following:
- This method is straightforward and easy to use. It directly prints the value of an attribute, making it suitable for quick debugging or displaying information.
- The code is clean and readable, making it accessible for programmers at various skill levels.
- By using attribute access (
object.attribute
), you directly retrieve the value associated with the attribute, enhancing code clarity.
However, this method is suitable when you want to print specific attributes individually. If you need to print all attributes dynamically or iterate over them, other methods like vars
, dir
, or __dict__
may be more appropriate.
Print Attributes of an Object in Python Using the getattr
Function
In the second method, the getattr()
function dynamically fetches the attribute specified by attribute_name
and prints its value.
getattr()
is a built-in Python function that is used to get the value of an attribute of an object. The function takes three parameters:
object
: The object whose attribute you want to retrieve.name
: A string representing the name of the attribute.default
(optional): The value to be returned if the attribute is not found. If this parameter is not provided, anAttributeError
is raised if the attribute is not found.
Let us try the code below.
attribute_name = "name"
print(getattr(person_instance, attribute_name))
Output:
John Doe
In this example, we use the getattr
function to dynamically access and print the value of an attribute. The function takes two arguments: the object (in this case, person_instance
) and the attribute name
(stored in the variable attribute_name
).
The getattr(person_instance, attribute_name)
retrieves and prints the value of the attribute specified by attribute_name
. We can repeat this process with city
to print its value.
Print Attributes of an Object in Python Using the __dict__
Attribute
In the third method, the __dict__
attribute directly provides a dictionary of the object’s attributes, allowing us to access and print the desired attribute.
In Python, __dict__
is a special attribute of a class or an object that provides a dictionary-like view of the attributes of the class or instance.
For a class, it contains the namespace used by instances of the class, and for an instance, it contains the instance’s attributes. Ensure that you filter or handle them appropriately if necessary.
attributes_dict = person_instance.__dict__
print(attributes_dict["name"])
Output:
John Doe
As we review above, we have an instance of a person_instance
with values of Person("John Doe", 30, "New York")
.
The __dict__
attribute of person_instance
is accessed. This attribute contains a dictionary-like view of the instance’s attributes, where keys are attribute names, and values are their corresponding values.
The value corresponding to the name
attribute is accessed and printed. In this case, it prints John Doe
.
Print Attributes of an Object in Python Using the dir()
Function in Python
As we move with the fourth method, the built-in dir()
function, when called without arguments, returns the list of the names in the current local scope, and when an object is passed as the argument, it returns the list of the object’s valid attributes.
To print the object’s attributes, we need to pass the object to the dir()
function and print the object’s attributes returned by the dir()
object. We can use the pprint()
method of the pprint
module to print the attributes in a well-formatted way.
pprint
stands for “pretty-print,” and it is a module in the Python standard library that provides a way to print data structures in a more human-readable and aesthetically pleasing format compared to the standard print
function. The pprint
module is part of the pprint
standard library.
The below example code demonstrates how to use the dir()
function to print the attributes of the object:
from pprint import pprint
mylist = list()
pprint(dir(mylist))
Output:
['__add__',
'__class__',
'__contains__',
'__delattr__',
'__delitem__',
'__dir__',
'__doc__',
'__eq__',
...
'__new__',
'__reduce__',
'__reduce_ex__',
'__repr__',
'__reversed__',
'__rmul__',
'__setattr__',
'__setitem__',
'__sizeof__',
'__str__',
'__subclasshook__',
'append',
'clear',
'copy',
...
'remove',
'reverse',
'sort']
This output shows the attributes and methods available for a Python list. It includes both special methods (those with double underscores, such as __add__
and __getitem__
) and regular methods (such as append
, clear
, copy
, etc.).
The special methods are used for operator overloading and customization, while the regular methods are standard list operations.
In summary, the output provides a comprehensive list of the attributes and methods that can be used with a Python list.
Print Attributes of an Object in Python Using the vars()
Function in Python
Lastly, the vars()
functions, when called without arguments, return the dictionary with the current local symbol table.
If an object is passed to the vars()
function, it returns the __dict__
attribute of the object. If the object provided as input does not have the __dict__
attribute, a TypeError
will be raised.
The below code example demonstrates how to use the vars()
function to print an object’s attributes in Python.
from pprint import pprint
pprint(vars(person_instance))
Output:
{"age": 30, "city": "New York", "name": "John Doe"}
Here, vars(person_instance)
returns a dictionary containing the instance variables of the person_instance
object, and pprint()
is used to print it in a more readable format.
Conclusion
In conclusion, delving into diverse methods for printing object attributes in Python equips developers with a versatile toolbox to address various programming challenges.
The print
statement, coupled with direct attribute access, ensures simplicity and readability for displaying individual attributes.
For a more dynamic approach, the getattr
function proves invaluable, enabling the use of variable attribute names for attribute retrieval.
The __dict__
attribute presents a comprehensive and dynamic solution, facilitating the iteration over all object attributes.
Furthermore, the dir
function provides an extensive list of object attributes and methods, offering an option for those seeking a detailed overview. Similarly, the vars
function simplifies attribute access by returning the __dict__
attribute.
Understanding these techniques empowers you to work efficiently with object attributes in your Python projects.