How to Pretty Print a Dictionary in Python
-
Use
pprint()
to Pretty Print a Dictionary in Python -
Use
json.dumps()
to Pretty Print a Dictionary in Python -
Use
yaml.dump()
to Pretty Print a Dictionary in Python
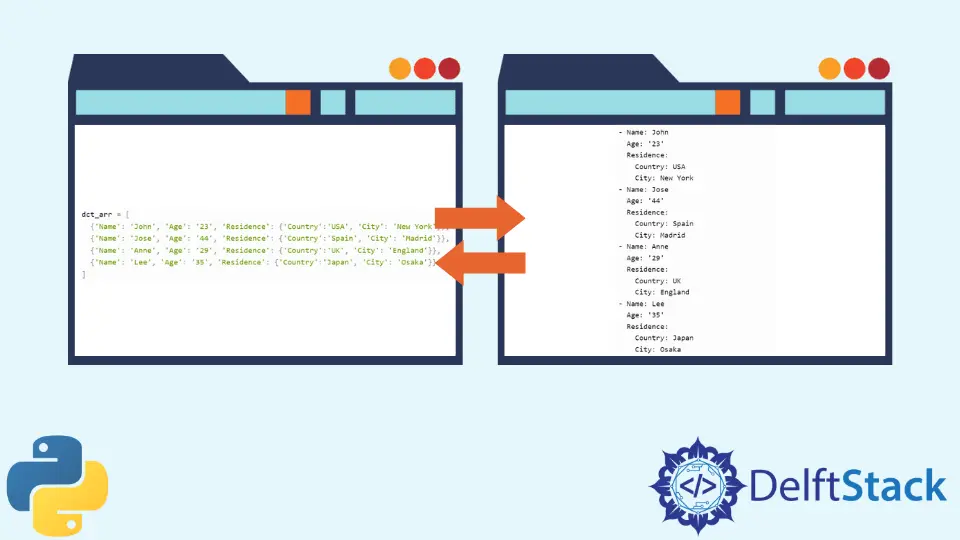
This tutorial will introduce how to pretty print a dictionary in Python. Pretty printing means to present something in a more readable format or style.
Use pprint()
to Pretty Print a Dictionary in Python
pprint
is a Python module that provides the capability to pretty print Python data types to be more readable. This module also supports pretty-printing dictionary.
Within the pprint
module there is a function with the same name pprint()
, which is the function used to pretty-print the given string or object.
First, declare an array of dictionaries. Afterward, pretty print it using the function pprint.pprint()
.
import pprint
dct_arr = [
{"Name": "John", "Age": "23", "Country": "USA"},
{"Name": "Jose", "Age": "44", "Country": "Spain"},
{"Name": "Anne", "Age": "29", "Country": "UK"},
{"Name": "Lee", "Age": "35", "Country": "Japan"},
]
pprint.pprint(dct_arr)
Output:
[{'Age': '23', 'Country': 'USA', 'Name': 'John'},
{'Age': '44', 'Country': 'Spain', 'Name': 'Jose'},
{'Age': '29', 'Country': 'UK', 'Name': 'Anne'},
{'Age': '35', 'Country': 'Japan', 'Name': 'Lee'}]
To compare, below is the output of a normal print()
statement:
[
{"Name": "John", "Age": "23", "Country": "USA"},
{"Name": "Jose", "Age": "44", "Country": "Spain"},
{"Name": "Anne", "Age": "29", "Country": "UK"},
{"Name": "Lee", "Age": "35", "Country": "Japan"},
]
The pprint()
output is definitely more readable. What it does is to break each dictionary element in the array right after the commas while also sorting the dictionary’s values by key.
If you don’t want your key-value pairs sorted by key, then you should set the sort_dicts
parameter to be False
in the pprint()
function.
Another thing to note is that pprint()
will not pretty print nested objects, including nested dictionaries. So if you expect your values to be nested, then this is not the solution for that as well.
Use json.dumps()
to Pretty Print a Dictionary in Python
Within the Python json
module, there is a function called dumps()
, which converts a Python object into a JSON string. Aside from the conversion, it also formats the dictionary into a pretty JSON format, so this can be a viable way to pretty print a dictionary by first converting it into JSON.
The dumps()
function accepts 3 parameters used for pretty printing: the object for conversion, a boolean value sort_keys
, which determines whether the entries should be sorted by key, and indent
, which specifies the number of spaces for indentation.
We will use the same example dictionary as above for this solution. sort_keys
is set to False
to disable sorting, and indent
is set to 4
spaces.
import json
dct_arr = [
{"Name": "John", "Age": "23", "Country": "USA"},
{"Name": "Jose", "Age": "44", "Country": "Spain"},
{"Name": "Anne", "Age": "29", "Country": "UK"},
{"Name": "Lee", "Age": "35", "Country": "Japan"},
]
print(json.dumps(dct_arr, sort_keys=False, indent=4))
Output:
[
{
"Age": "23",
"Country": "USA",
"Name": "John"
},
{
"Age": "44",
"Country": "Spain",
"Name": "Jose"
},
{
"Age": "29",
"Country": "UK",
"Name": "Anne"
},
{
"Age": "35",
"Country": "Japan",
"Name": "Lee"
}
]
Compared to the output of the pprint()
function, this is much more readable, although it costs more lines since it’s in pretty JSON format.
What if the values given have a nested dictionary within them? Let’s edit the example a bit and take a look at the output.
import json
dct_arr = [
{'Name': 'John', 'Age': '23', 'Residence': {'Country':'USA', 'City': 'New York'}},
{'Name': 'Jose', 'Age': '44', 'Residence': {'Country':'Spain', 'City': 'Madrid'}},
{'Name': 'Anne', 'Age': '29', 'Residence': {'Country':'UK', 'City': 'England'}},
{'Name': 'Lee', 'Age': '35', 'Residence': {'Country':'Japan', 'City': 'Osaka'}}
]
print(json.dumps(dct_arr, sort_keys=False, indent=4))
Output:
[
{
"Name": "John",
"Age": "23",
"Residence": {
"Country": "USA",
"City": "New York"
}
},
{
"Name": "Jose",
"Age": "44",
"Residence": {
"Country": "Spain",
"City": "Madrid"
}
},
{
"Name": "Anne",
"Age": "29",
"Residence": {
"Country": "UK",
"City": "England"
}
},
{
"Name": "Lee",
"Age": "35",
"Residence": {
"Country": "Japan",
"City": "Osaka"
}
}
]
Evidently, pretty JSON nested dictionaries are supported using json.dump()
, and visually it looks clean and very readable even if it’s nested.
Use yaml.dump()
to Pretty Print a Dictionary in Python
Another way to pretty print a dictionary is by using the dump()
function of the yaml
module. It serves the same purpose as the json.dumps()
function but in YAML format instead of JSON.
First off, install the YAML module using pip
:
pip install pyyaml
or if using Python 3 and pip3
:
pip3 install pyyaml
Let’s try it out with the same nested example used in the JSON example.
Take note of the new parameter default_flow_style
, which determines whether the dump’s output style should be inline
or block
. In this case, the output should be block-style since we want it to be readable, so set this parameter to False
.
import yaml
dct_arr = [
{"Name": "John", "Age": "23", "Residence": {"Country": "USA", "City": "New York"}},
{"Name": "Jose", "Age": "44", "Residence": {"Country": "Spain", "City": "Madrid"}},
{"Name": "Anne", "Age": "29", "Residence": {"Country": "UK", "City": "England"}},
{"Name": "Lee", "Age": "35", "Residence": {"Country": "Japan", "City": "Osaka"}},
]
print(yaml.dump(dct_arr, sort_keys=False, default_flow_style=False))
Output:
- Name: John
Age: '23'
Residence:
Country: USA
City: New York
- Name: Jose
Age: '44'
Residence:
Country: Spain
City: Madrid
- Name: Anne
Age: '29'
Residence:
Country: UK
City: England
- Name: Lee
Age: '35'
Residence:
Country: Japan
City: Osaka
In summary, it is subjective whether or not the YAML dump()
function is more readable than the JSON dumps()
. It’s up to personal preference or what type of output is required. Both functions beat the output of pprint
when it comes to more complex data structures or nested objects.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- How to Convert a Dictionary to a List in Python
- How to Get All the Files of a Directory
- How to Find Maximum Value in Python Dictionary
- How to Sort a Python Dictionary by Value
- How to Merge Two Dictionaries in Python 2 and 3