How to Parse String to List in Python
-
Parse String to List With the
str.split()
Function in Python -
Parse String to List With the
str.strip()
Function in Python -
Parse String to List With the
json.loads()
Function in Python -
Parse String to List With the
ast.literal_eval()
Function in Python
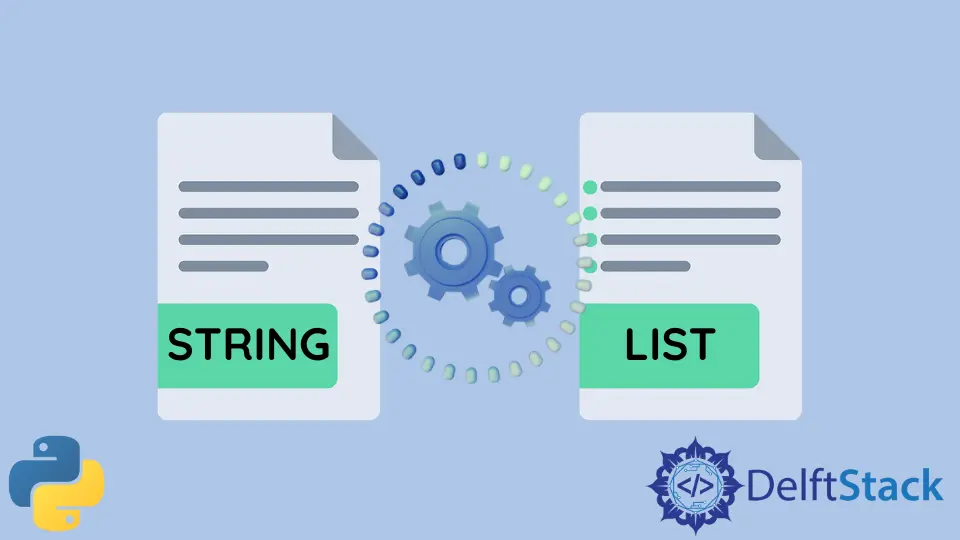
In this tutorial, we are going to learn the methods to parse a string to a list in Python.
Parse String to List With the str.split()
Function in Python
If in a scenario, we have a string representation of a list like '[ "A","B","C" , " D"]'
and want to convert that representation into an actual list of strings, we can use the str.split()
function to split the string on the basis of each ,
. The str.split()
function takes a delimiter/separator as an input parameter, splits the calling string based on the delimiter, and returns a list of substrings. The code sample below shows us how to parse a string representation of a list into an actual list with the str.split()
function.
stringlist = '[ "A","B","C" , " D"]'
print(stringlist.split(","))
Output:
['[ "A"', '"B"', '"C" ', ' " D"]']
We converted the stringlist
string into a list by splitting it based on ,
with the stringlist.split(",")
function. As is apparent from the output, this approach has several problems and does not properly meet our requirements.
Parse String to List With the str.strip()
Function in Python
To further convert a string like this into a list, we can use the str.strip()
function. This str.strip()
function also takes the delimiter/separator as an input parameter, strips the calling string based on the delimiter, and returns a list of much cleaner substrings. The sample code below shows us how to parse a string representation of a list into an actual list with the str.strip()
function.
stringlist = '[ "A","B","C" , " D"]'
print(stringlist.strip(","))
Output:
[ "A","B","C" , " D"]
We converted the stringlist
string into a list by splitting it on the basis of ,
with the stringlist.split(",")
function. We get a much cleaner list of strings this time around. The only disadvantage of this approach is that there are some unwanted blank spaces like the space in the fourth element of the list.
Parse String to List With the json.loads()
Function in Python
We can also use the json
module for our specific problem. The json.loads()
function takes a JSON object as parameter, deserializes JSON object, and returns the results in a list. The JSON object parameter, in this case, can also be a string. The sample code below shows us how to parse a string representation of a list into an actual list with the json.loads()
function.
import json
stringlist = '[ "A","B","C" , " D"]'
print(json.loads(stringlist))
Output:
['A', 'B', 'C', ' D']
We converted our stringlist
string into a cleaner list with the json.loads(stringlist)
function in Python. The only difference between the json.loads()
function and our previous approaches is that we don’t have to specify any delimiter or separator character here. The json.loads()
function automatically determines the delimiter for us. This method also contains the problem of unwanted blank spaces.
Parse String to List With the ast.literal_eval()
Function in Python
One other method to solve our specific problem is the ast
module. The ast.literal_eval()
function takes a string representation of a Python literal structure like tuples, dictionaries, lists, and sets. If we pass the string into that literal structure, it returns the results. In our case, we have a string representation of a list. So, the ast.literal_eval()
function takes this string, parses it into a list, and returns the results. The following code snippet shows us how to parse a string representation of a list into an actual list with the ast.literal_eval()
function.
import ast
stringlist = '[ "A","B","C" , " D"]'
print(ast.literal_eval(stringlist))
Output:
['A', 'B', 'C', ' D']
We converted the stringlist
string into a cleaner list with the ast.literal_eval()
function in Python. Similar to the previous approach, we don’t have to specify a delimiter or a separator. Also similar to the previous approach, this method has the same problem of unwanted blank spaces. But these blank spaces can be easily removed.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python