How to Overwrite a File in Python
-
Overwrite a File in Python Using the
open()
Function -
Overwrite a File in Python Using the
file.seek()
andfile.truncate()
Method -
Overwrite a File in Python Using
shutil
Module’scopyfile()
Function -
Overwrite a File in Python Using
os
Module’sremove()
andrename()
Functions -
Overwrite a File in Python Using the
os.replace()
Method -
Overwrite a File in Python Using the
pathlib
Module’swrite_text
Method
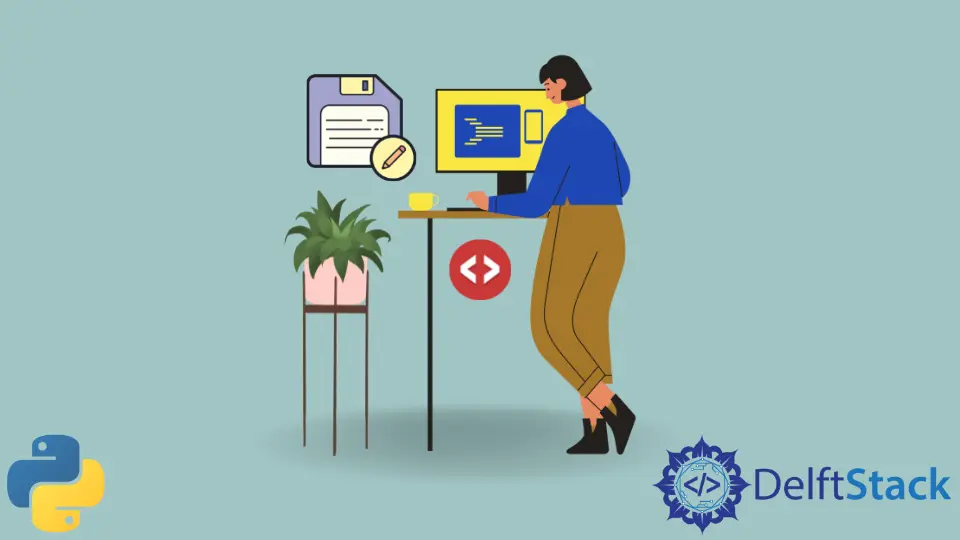
In Python programming, file handling is a critical skill, and overwriting files is a common operation. However, it’s a double-edged sword. While it’s instrumental in updating file content, it can also lead to irreversible data loss if not handled with caution.
This tutorial will demonstrate various methods to overwrite a file in Python. We will look into methods to write new text by deleting the already saved text and how we can first read the data of the file, apply some actions and changes on it, and then overwrite it on the old data.
Overwrite a File in Python Using the open()
Function
The open(file, mode)
function takes file
(a path-like object) as input and returns a file object as output. The file
input can be a string or bytes object and contains the file path. The mode
is the mode we want to open the file in; it can be r
for the read mode, w
for the write or a
for the append mode, etc.
Overwriting Files with Write Mode
When the open()
function is used with the w
mode, it opens the file for writing. If the file already contains data, this mode will erase all existing data and allow new data to be written. It’s a straightforward method for completely overwriting a file.
Example:
with open("myFolder/myfile.txt", "w") as myfile:
myfile.write("newData")
Explanation:
In this example, the with
statement is used to ensure that the file is properly closed after its suite finishes. The open()
function opens myfile.txt
in write mode. If the file exists, it’s overwritten; if it doesn’t exist, it’s created. The write()
method then writes newData
into the file, replacing any existing content.
Reading and Overwriting Files
There might be scenarios where you need to read the existing data before overwriting the file. In such cases, the file can be opened in r+
mode, which allows both reading and writing operations.
Example:
with open("myFolder/myfile.txt", "r+") as myfile:
data = myfile.read()
myfile.write("newData")
Explanation:
Here, the file is opened in r+
mode, enabling both reading and writing. The read()
method is used to read the existing data into the variable data
. The write()
method then writes newData
into the file, starting from the beginning of the file, effectively overwriting the existing content.
Key Takeaways
- The
open()
function is versatile, allowing files to be opened in various modes for different operations. - The
w
mode is used for overwriting files. It erases all existing content and writes new data from the beginning of the file. - The
r+
mode is handy when you need to read the file before overwriting it.
Overwrite a File in Python Using the file.seek()
and file.truncate()
Method
In this session, we will focus on a more nuanced technique involving the seek()
and truncate()
methods. This approach is particularly useful when you need to read the file before overwriting it.
The seek()
Method
Syntax
file.seek(offset, whence)
Parameters
-
offset
: It is the number of bytes the cursor should move, and it can be a positive or negative integer. -
whence
:It is optional and represents the reference position for the offset. Its default value is 0. The possible values are:
- 0: Indicates the beginning of the file.
- 1: Indicates the current cursor position.
- 2: Indicates the end of the file.
Explanation
The seek()
method is used to change the current file cursor’s position. The offset
parameter determines the number of bytes to move the cursor. The whence
parameter is optional and indicates the reference position from where the cursor should move. By default, it starts from the beginning of the file.
The truncate()
Method
Syntax
file.truncate(size)
Parameters
size
: It is optional and represents the size up to which the file should be resized. If not provided, the file is truncated at the current file position.
Explanation
The truncate()
method is used to resize the file to a specified size. If the size is not specified, it truncates the file at the current position, meaning it will remove the content present after the current position.
Example with Detailed Explanation
with open("myFolder/myfile.txt", "r+") as myfile:
data = myfile.read()
myfile.seek(0)
myfile.write("newData")
myfile.truncate()
Explanation
- The file is opened in read-write mode (‘r+’), enabling both reading and writing operations.
- The
read()
method is invoked to read the existing content of the file and store it in the variabledata
. - The
seek(0)
method repositions the cursor to the start of the file, readying it for the overwriting process. - The
write("newData")
method inscribes the new data into the file from the beginning. - Finally, the
truncate()
method is called to eliminate any residual old data, ensuring the file is now exclusively occupied by the new data.
Key Insights
Utilizing the seek()
and truncate()
methods offers a nuanced approach to file overwriting in Python. It’s a technique that marries precision and flexibility, allowing developers to overwrite files with new data efficiently while also having the option to read the file first.
Always ensure to handle files with utmost caution to avert unintended data loss. Implementing practices like creating file backups or utilizing version control systems can be instrumental in safeguarding data integrity.
Overwrite a File in Python Using shutil
Module’s copyfile()
Function
In Python file handling, the shutil
module emerges as a powerful tool, equipped with versatile functions. One such function, copyfile()
, is adept at overwriting files, ensuring that the new content seamlessly replaces the old. Let’s explore this method in detail.
The copyfile()
Method
Syntax
shutil.copyfile(src, dst)
Parameters
src
: The source file path, a string indicating the location of the file to be copied.dst
: The destination file path, a string indicating where the copied file content should be stored. If the destination file already exists, it will be overwritten.
Explanation
The copyfile()
method in the shutil
module is used to copy the content of a source file to a destination file. This method is straightforward and efficient for overwriting files, as it directly replaces the content of the destination file with that of the source file.
The shutil
module part of the Python Standard Library, so you typically won’t need to install anything extra.
Example with Detailed Explanation
import shutil
shutil.copyfile("myFolder/sourcefile.txt", "myFolder/destinationfile.txt")
Explanation
- First, the
shutil
module is imported to gain access to thecopyfile()
method. - The
copyfile()
method is then invoked, withmyFolder/sourcefile.txt
as the source file and ‘myFolder/destinationfile.txt’ as the destination file. - The content of
sourcefile.txt
is copied todestinationfile.txt
. Ifdestinationfile.txt
already exists, its content is overwritten with the content fromsourcefile.txt
; otherwise, a new file is created.
Key Insights
The copyfile()
method is a straightforward and efficient way to overwrite files. It’s particularly useful when you want to replace the content of a file completely. However, it doesn’t allow for partial overwriting or appending; it’s an all-or-nothing operation.
Given that this method overwrites the destination file completely, caution is paramount to avoid accidental data loss. Always ensure that overwriting is intentional and consider creating backups of important files before proceeding.
Overwrite a File in Python Using os
Module’s remove()
and rename()
Functions
The os
module in Python provides a plethora of functions to interact with the operating system. Among these, the remove()
and rename()
functions are instrumental for file handling, especially when the task involves overwriting files. In this section, we will delve into the intricate process of utilizing these functions to overwrite files effectively.
The remove()
and rename()
Functions
Syntax
os.remove(path)
os.rename(src, dst)
Parameters
path
: The path of the file that needs to be removed. It should be a string representing the file’s location.src
: The source file path, a string that indicates the location of the file to be renamed.dst
: The destination file path, a string that specifies the new name or location of the file.
Explanation
The os.remove()
function is used to delete a file, specified by the file path. It does not work on directories; for removing directories, os.rmdir()
or shutil.rmtree()
should be used.
The os.rename()
function is used to rename a file or directory. It takes two arguments, the current filename and the new filename. If the specified file is in the current working directory, only the filename needs to be passed; otherwise, the file path should be included.
Example with Detailed Explanation
import os
# Create a new file with the new content
with open("myFolder/newfile.txt", "w") as file:
file.write("newData")
# Remove the old file
os.remove("myFolder/oldfile.txt")
# Rename the new file to have the name of the old file
os.rename("myFolder/newfile.txt", "myFolder/oldfile.txt")
Explanation
- We begin by importing the
os
module to access theremove()
andrename()
functions. - A new file named
newfile.txt
is created in themyFolder
directory, andnewData
is written into this file. - The
os.remove()
function is then called to delete theoldfile.txt
file from themyFolder
directory. - Subsequently, the
os.rename()
function is invoked to renamenewfile.txt
tooldfile.txt
. This effectively overwrites the old file with the new content.
Key Insights
This method of overwriting files is particularly useful when you need to replace the entire content of a file. It involves creating a new file with the updated content, deleting the old file, and then renaming the new file to have the name of the old file.
It’s crucial to exercise caution when using the os.remove()
function as it permanently deletes files. Always ensure that you have a backup of important data to prevent accidental loss.
Overwrite a File in Python Using the os.replace()
Method
The os.replace()
method emerges as a handy tool for overwriting files. It is used to rename or move a file or a directory, and it’s atomic, meaning it ensures that the operation is completed successfully or not executed at all, reducing the risk of file corruption. In this session, we will explore how to leverage this method to overwrite files seamlessly.
The os.replace()
Method
Syntax
os.replace(src, dst)
Parameters
src
: This is a string representing the path of the source file that you want to move or rename.dst
: This is a string representing the path of the destination, where the source file will be moved or the name it will be changed to.
Explanation
The os.replace()
method is used for renaming or moving files and directories. The operation is atomic, meaning that if the operation fails, no changes will be made, ensuring that your files remain intact. This is particularly useful to prevent file corruption or partial overwrites, ensuring that the file is either completely overwritten or left unchanged.
Example with Detailed Explanation
import os
# Create a new file with new content
with open("myFolder/newfile.txt", "w") as file:
file.write("newData")
# Atomically replace the old file with the new file
os.replace("myFolder/newfile.txt", "myFolder/oldfile.txt")
Explanation
- We start by importing the
os
module to gain access to thereplace()
method. - A new file named
newfile.txt
is created in themyFolder
directory, andnewData
is written into this file. - The
os.replace()
method is then invoked to atomically replaceoldfile.txt
withnewfile.txt
. This operation ensures thatoldfile.txt
is overwritten with the content ofnewfile.txt
.
Key Insights
The os.replace()
method is especially beneficial when you need an atomic operation to overwrite files, ensuring data integrity and reducing the risk of file corruption. It’s a straightforward and efficient way to replace the content of a file.
Overwrite a File in Python Using the pathlib
Module’s write_text
Method
The pathlib
module in Python simplifies the way we handle files and directories, offering a more readable and easier syntax compared to the traditional method of handling paths. One of the powerful methods provided by this module is write_text
, which is used for writing text to a file. In this section, we will delve into how to use this method to overwrite a file.
The write_text
Method
Syntax
Path.write_text(data, encoding=None, errors=None)
Parameters
data
: The string data to be written to the file.encoding (optional)
: The encoding format used to represent the data. Default is ‘utf-8’.errors (optional)
: Specifies how encoding errors are handled. Default is ‘strict’.
Explanation
The write_text
method is part of the Path
object in the pathlib
module. It opens the file in text mode and writes a string to it. If the file already contains data, the write_text
method will overwrite it. This method returns the number of characters written to the file.
Example with Detailed Explanation
from pathlib import Path
# Specify the file path
file_path = Path("myFolder/myfile.txt")
# Write new data to the file, overwriting existing data if the file exists
num_chars_written = file_path.write_text("This is the new content")
Explanation
- We begin by importing the
Path
class from thepathlib
module. - A
Path
object is created with the specified file pathmyFolder/myfile.txt
. - The
write_text
method is invoked on thePath
object, and a string “This is the new content” is written to the file. If the file already exists, its content is overwritten; otherwise, a new file is created. - The method returns the number of characters written to the file, which is stored in the variable
num_chars_written
.
Key Insights
The pathlib
module, and particularly the write_text
method, provides a more object-oriented and intuitive approach to file handling. It not only simplifies the syntax but also enhances the readability of the code, making it a preferred option for many Python developers.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn