Python os.replace() Method
-
Syntax of the
os.replace()
Method -
Example Codes: Rename Files Using the
os.replace()
Method in Python -
Example Codes:
OSError
Exception While Using theos.replace()
Method in Python -
Example Codes:
os.replace()
vsos.rename()
Method in Python -
Example Codes: Handle Possible Errors With the
os.replace()
Method in Python -
Example Codes:
os.replace()
Method With theAccess is denied
Error in Python - Conclusion
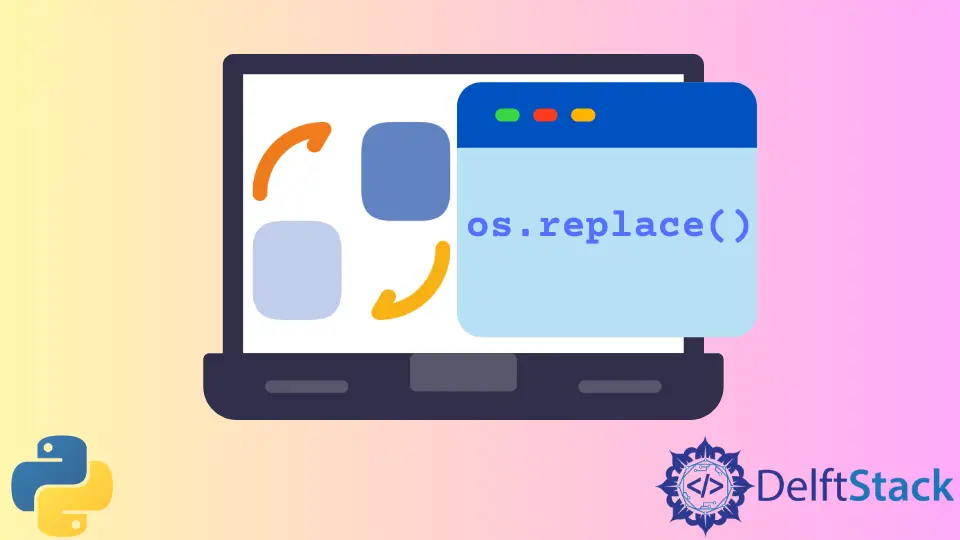
The os.replace()
method is a tool in Python that is responsible for renaming files. It is essential in the field of file manipulation, allowing developers to easily change file names within their Python applications.
In this article, we will look into the internal functions of the os.replace()
method, exploring its syntax, parameters, and practical applications. We’ll go over its features, potential problems, and even how to handle various exceptions that may occur while file renaming.
Syntax of the os.replace()
Method
os.replace(src, dst, *, src_dir_fd=None, dst_dir_fd=None)
Parameters
src |
This is the source file we want to rename. |
dst |
This is the destination name to be given to the newly renamed file. |
src_dir_fd (optional) |
This is the source file directory. |
dst_dir_fd (optional) |
This is the destination file directory. |
Return
This method only changes the name of the given source file and copies it to the destination path but does not return any value.
Example Codes: Rename Files Using the os.replace()
Method in Python
We must import the os
module to use the os.replace()
method. The os.replace()
method uses the two necessary parameters: source and destination.
Remember, the source and destination are the pathes. The rest of the parameters are optional and are not necessary to pass as a parameter.
In the following code, we begin by importing the os
module. Next, we define two variables: source_file
and dest_location
.
The former, "myFile.txt"
, represents the file we wish to rename. The latter, "myRenamedFile.txt"
, is the new name we want for the source file after the renaming process.
We then employ the os.replace()
method, passing in source_file
and dest_location
as arguments. This action effectively renames the source file to the desired destination.
If the operation is successful, we print "File is renamed successfully"
to signal that the renaming process concluded without any issues.
import os
source_file = "myFile.txt"
dest_location = "myRenamedFile.txt"
os.replace(source_file, dest_location)
print("File is renamed successfully.")
Output:
File is renamed successfully.
The output confirms that the file "myFile.txt"
was successfully renamed to "myRenamedFile.txt"
, and the success message was displayed.
Similarly, if we want the same file to be renamed in a different directory, the source
remains the same, and the destination
is in a different folder. Let’s take this example to elaborate further.
In the following code, we then define two variables, source_file
("myFile.txt"
) and dest_location
("DelftStack\myRenamedFile.txt"
). The key action takes place when we call os.replace(source_file, dest_location)
.
This os.replace(source_file, dest_location)
line renames the source file to the name and location specified in dest_location
. It’s important to note that if the destination file already exists, this action will overwrite it, ensuring a consistent file name.
import os
source_file = "myFile.txt"
dest_location = "DelftStack\myRenamedFile.txt"
os.replace(source_file, dest_location)
print("File is renamed successfully.")
Output:
File is renamed successfully.
In the output, we print a success message, "File is renamed successfully"
, confirming the error-free completion of the renaming process.
Example Codes: OSError
Exception While Using the os.replace()
Method in Python
In this method, if we want to rename a file to a new filesystem (another disk drive), then the os.replace()
method will return the OSError
exception.
In this code, we then define two variables: source_file
("myFile.txt"
) located in the main C drive, and dest_location
("E:\myRenamedFile.txt"
), which specifies the new file name and its location in another drive, specifically the E drive.
The critical action occurs when we invoke os.replace(source_file, dest_location)
. This command is intended to rename the source file to the name and location provided in dest_location
.
import os
source_file = "myFile.txt"
dest_location = "E:\myRenamedFile.txt"
os.replace(source_file, dest_location)
print("File is renamed successfully.")
Output:
OSError: The system cannot move the file to a different disk drive: 'myFile.txt' -> 'E:\\myRenamedFile.txt'
As you can see in the output, we encounter an error represented by the output message: "OSError: The system cannot move the file to a different disk drive: 'myFile.txt' -> 'E:\myRenamedFile.txt'"
. This error informs us that we cannot move the file to a different disk drive, as the source and destination are on separate drives.
Example Codes: os.replace()
vs os.rename()
Method in Python
We see that in the os.replace()
method, we have renamed the file by passing the source and destination parameters. Similarly, we have the os.rename()
method, which can also rename the file.
But, the only difference is that in os.rename()
, when the destination file already exists, it will show a FileExistsError
as an output.
Now, let’s code both methods in the same program to evaluate the difference.
import os
source_file = "myFile.txt"
dest_location = "DelftStack\myNewFile.txt"
# replace method
os.replace(source_file, dest_location)
print("File is renamed successfully.")
# rename method
os.rename(source_file, dest_location)
print("File is renamed successfully.")
Output:
File is renamed successfully.
FileExistsError: Cannot create a file when that file already exists: 'myFile.txt' -> 'DelftStack\\myFile.txt'
If we see the output, we can observe that with os.replace()
, it will rename the file and override the same file if it already exists. But the os.rename()
method will show an error if the file is already there.
Example Codes: Handle Possible Errors With the os.replace()
Method in Python
In this code, our objective is to rename a file using Python’s os.replace()
method while simultaneously handling various potential exceptions. We begin by importing the os
module to facilitate file operations.
Then, we define two variables: source
(".myfile.txt"
, and dest
(".myNewFile.txt"
). The renaming operation is wrapped in a try-except
block to manage potential exceptions that might arise during the process.
When we execute the code, it attempts to rename the file from source
to dest
. If this operation completes successfully, we receive the output message, "File is renamed successfully"
.
import os
source = ".myfile.txt"
dest = ".myNewFile.txt"
try:
os.replace(source, dest)
print("File is renamed successfully.")
except IsADirectoryError:
print("source is a file, but the destination is a directory exception.")
except NotADirectoryError:
print("source is a directory, but the destination is a file exception.")
except PermissionError:
print("Permission exception.")
except OSError as error:
print("Other errors")
Output:
File is renamed successfully.
However, the code is also prepared to handle exceptions. For instance, if we encounter an IsADirectoryError
, it indicates that the source is a file, but the destination is a directory, and the corresponding exception message is displayed.
Similarly, if a NotADirectoryError
occurs, it implies that the source is a directory, but the destination is a file, and the appropriate exception message is shown. If a PermissionError
emerges due to any permission issues, we display a "Permission exception"
message.
Lastly, if any other unanticipated errors occur, they are captured under the OSError
exception, and a generic "Other errors"
message is presented.
Example Codes: os.replace()
Method With the Access is denied
Error in Python
In the os.replace()
method, sometimes there is a possibility that we might face an Access is denied
error, which is a permission exception. This situation happens when the destination file path is in a read-only
mode.
To access the destination file path, we need to uncheck the read-only
attribute from the file properties. In the following example, you can see this type of access-denied output in the console.
In this code, we then define two variables: source_file
("myFile.txt"
) and dest_location
("DelftStack\myRenamedFile.txt"
). The pivotal step occurs when we execute the os.replace(source_file, dest_location)
method, which is responsible for renaming the file.
However, in this case, the code encounters a PermissionError
, as indicated by the output message, "PermissionError: Access is denied: '\Junaid'"
.
import os
# source and destination file
source_file = "myFile.txt"
dest_location = "DelftStack\myRenamedFile.txt"
os.replace(source_file, dest_location)
print("File is renamed successfully.")
Output:
PermissionError: Access is denied: '\\Junaid'
This particular error arises when the destination file path is set to a read-only mode, which leads to an "Access is denied"
scenario. It essentially means that we don’t have the necessary permissions to perform the renaming operation successfully.
Conclusion
The os.replace()
method in Python is a handy tool for renaming files, but it comes with specific rules and potential challenges. It ensures that source and destination formats match and allows for renaming files even across different directories.
When renaming, it can overwrite existing files, unlike the os.rename() method
. Handling permissions and potential errors is crucial, as seen in the examples, where a PermissionError
can occur if trying to access read-only files.
Overall, os.replace()
is a powerful asset for file management, offering a robust solution for file renaming while necessitating careful attention to formats and potential exceptions.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn