Python File Open Modes
-
r
Mode in Python File Opening -
r+
Mode in Python File Opening -
w
Mode in Python File Opening -
w+
Mode in Python File Opening -
a
Mode in Python File Opening -
a+
Mode in Python File Opening -
x
Mode in Python File Opening
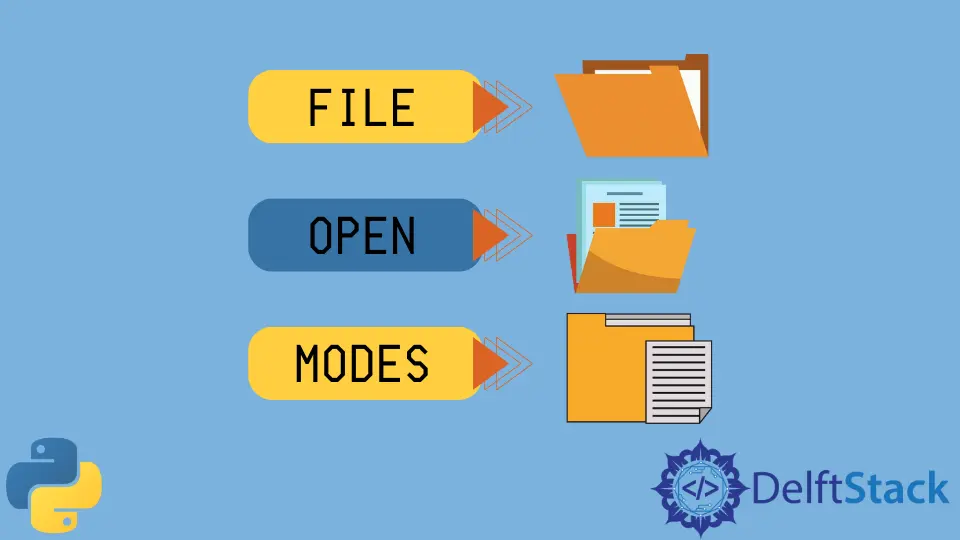
Python, just like other programming languages, supports file handling, and it allows the programmers to deal with files and essentially perform some basic operations like reading, writing, and some other file handling options to operate on files.
The handiest and most important function in dealing with files is the open()
function. It is used to open the file and return an equivalent file object. The open()
function mainly takes two parameters, filename
and mode
.
In this tutorial, we will learn about the different modes in which a file can be opened in Python.
r
Mode in Python File Opening
The r
mode is used when we want to open the file for reading. The file pointer in this mode is placed at the starting point of the file.
The r
mode can be used in the open()
function in the following way:
f1 = open("god.txt", "r")
r+
Mode in Python File Opening
The r+
mode is used to open a file for both reading and writing. Just like in the previous mode, the file pointer in this mode is placed at the starting point of the file as well.
The r+
mode can be used in the open()
function in the following way:
f1 = open("god.txt", "r+")
For opening the file for writing and reading in the binary format, we can use the rb+
mode.
f1 = open("god.txt", "rb+")
w
Mode in Python File Opening
The w
mode is used to open a file for the purpose of writing only. If the file already exists, it truncates the file to zero length and otherwise creates a new file if it doesn’t exist yet. The file pointer in this mode is placed at the starting point of the file.
The w
mode can be used in the open()
function in the following way:
f1 = open("god.txt", "w")
w+
Mode in Python File Opening
The w+
mode opens the file for reading and writing. If the file already exists, it is truncated, and otherwise, a new file is created if it doesn’t exist. The file pointer in this mode is placed at the starting point of the file.
The w+
mode can be used in the open()
function in the following way.
f1 = open("god.txt", "w+")
For opening the file in binary format, we can use the wb+
mode.
f1 = open("god.txt", "wb+")
a
Mode in Python File Opening
The a
mode opens the file for the purpose of appending. The file pointer in this mode is placed at the end of the file if it already exists in the system. If the file does not exist, then it is created for writing.
The a
mode can be used in the open()
function in the following way.
f1 = open("god.txt", "a")
a+
Mode in Python File Opening
The a+
mode opens the file for both reading and appending. The file pointer in this mode is placed at the end of the file if it already exists in the system. The file opens in the append mode. If the file does not exist, then it is created for writing.
The a+
mode can be used in the open()
function in the following way.
f1 = open("god.txt", "a+")
For opening the file in binary mode, we can add the ab+
mode.
f1 = open("god.txt", "ab+")
x
Mode in Python File Opening
This mode is available for the versions Python 3 and above. The x
mode opens the file for exclusive creation, failing if the file with that name is already existent. When exclusive creation is specified, it means that this mode will not create a file if the file with the specified name already exists. In the x
mode, the file is only writeable, but in x+
mode, the file is opened as both readable and writeable.
This mode is significant and comes in handy when we don’t want to accidentally truncate an already existing file with the a
or w
modes.
The x
mode can be used in the open()
function in the following way.
f1 = open("god.txt", "x")
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn