How to Fix Python Math Domain Errors in math.log Function
- Understanding the math.log Function
- Solution 1: Validate Input Before Calling math.log
- Solution 2: Use Exception Handling
- Solution 3: Use Conditional Logic for Logarithm Base
- Conclusion
- FAQ
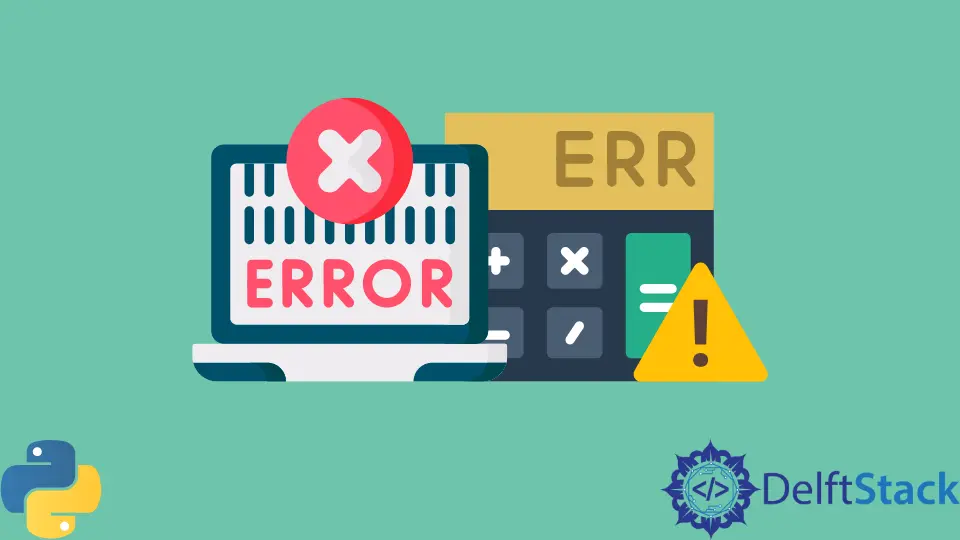
When working with Python, you may come across the dreaded ValueError: math domain error while using the math.log function. This error typically occurs when you attempt to calculate the logarithm of a non-positive number, which is outside the valid range for logarithmic functions.
In this tutorial, we’ll explore how to fix this error, ensuring your code runs smoothly and efficiently. We’ll cover the common causes of this error, provide practical solutions, and walk you through clear examples. By the end of this article, you’ll have a solid understanding of how to handle math domain errors in Python, allowing you to write more robust and error-free code.
Understanding the math.log Function
Before diving into solutions, it’s crucial to understand how the math.log function operates. The math.log function in Python is used to calculate the natural logarithm of a number. Its primary requirement is that the input must be a positive number; otherwise, it will raise a ValueError indicating a math domain error.
For example, calling math.log(-1)
or math.log(0)
will result in an error. This behavior is consistent with the mathematical definition of logarithms, where the logarithm of zero or a negative number is undefined. Therefore, ensuring that your input is valid is the key to avoiding this error.
Solution 1: Validate Input Before Calling math.log
One of the simplest and most effective ways to prevent the math domain error is to validate the input before passing it to the math.log function. By checking if the input number is greater than zero, you can avoid the error altogether. Here’s how you can implement this:
import math
def safe_log(x):
if x > 0:
return math.log(x)
else:
return "Input must be greater than zero."
result = safe_log(10)
print(result)
result = safe_log(-5)
print(result)
Output:
2.302585092994046
Input must be greater than zero.
In this code, we define a function called safe_log
that checks if the input x
is greater than zero. If it is, the function calculates and returns the logarithm. If not, it returns a message indicating that the input must be greater than zero. This simple validation step can save you from encountering a math domain error and make your code more user-friendly.
Solution 2: Use Exception Handling
Another effective way to handle the math domain error is through exception handling. By using a try-except block, you can catch the ValueError when it occurs and respond appropriately without crashing your program. Here’s an example:
import math
def log_with_exception_handling(x):
try:
return math.log(x)
except ValueError:
return "ValueError: Input must be greater than zero."
result = log_with_exception_handling(5)
print(result)
result = log_with_exception_handling(0)
print(result)
Output:
1.6094379124341003
ValueError: Input must be greater than zero.
In this example, we define a function log_with_exception_handling
that tries to calculate the logarithm of x
. If x
is not valid, the ValueError is caught, and a friendly message is returned instead. This method not only prevents your program from crashing but also provides clear feedback to the user regarding the issue.
Solution 3: Use Conditional Logic for Logarithm Base
If your application requires the use of logarithms with different bases, it’s essential to ensure that the input is valid for the base you intend to use. For instance, if you’re calculating logarithms in a base other than e (like base 10), you can implement conditional logic to handle the math domain error effectively. Here’s a sample implementation:
import math
def log_with_base(x, base=math.e):
if x > 0 and base > 1:
return math.log(x, base)
else:
return "Input must be greater than zero and base must be greater than one."
result = log_with_base(100, 10)
print(result)
result = log_with_base(-10, 10)
print(result)
Output:
2.0
Input must be greater than zero and base must be greater than one.
In this code, we define the log_with_base
function, which checks both the input number x
and the logarithm base
. If either condition is not met, it returns a message indicating the requirements. This approach allows for flexibility in calculating logarithms while ensuring that you avoid domain errors.
Conclusion
Encountering a math domain error when using the math.log function in Python can be frustrating, but it’s a common issue that can be easily fixed. By validating input, using exception handling, or implementing conditional logic for different logarithm bases, you can prevent this error and enhance your code’s robustness. Understanding how to handle these errors not only improves your programming skills but also makes your applications more user-friendly. With the methods outlined in this tutorial, you can confidently navigate through any math domain errors and keep your Python projects running smoothly.
FAQ
-
What causes a math domain error in Python?
A math domain error in Python occurs when you attempt to calculate the logarithm of a non-positive number using the math.log function. -
How can I handle math domain errors in my code?
You can handle math domain errors by validating input before calling math.log, using exception handling, or implementing conditional logic for different logarithm bases. -
Is there a way to calculate logarithms for negative numbers?
Logarithms are not defined for negative numbers or zero. You must ensure that the input is positive before calculating the logarithm. -
Can I use math.log with different bases?
Yes, you can use math.log with different bases by providing a second argument that specifies the base, but make sure the input number is positive. -
What should I do if I encounter a ValueError?
If you encounter a ValueError, check the input value you are using with the math.log function and ensure it is greater than zero.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python