How to List All the Methods of a Python Module
-
List All the Methods of a Python Module Using the
dir()
Method -
List All the Methods of a Python Module Using the
inspect()
Module
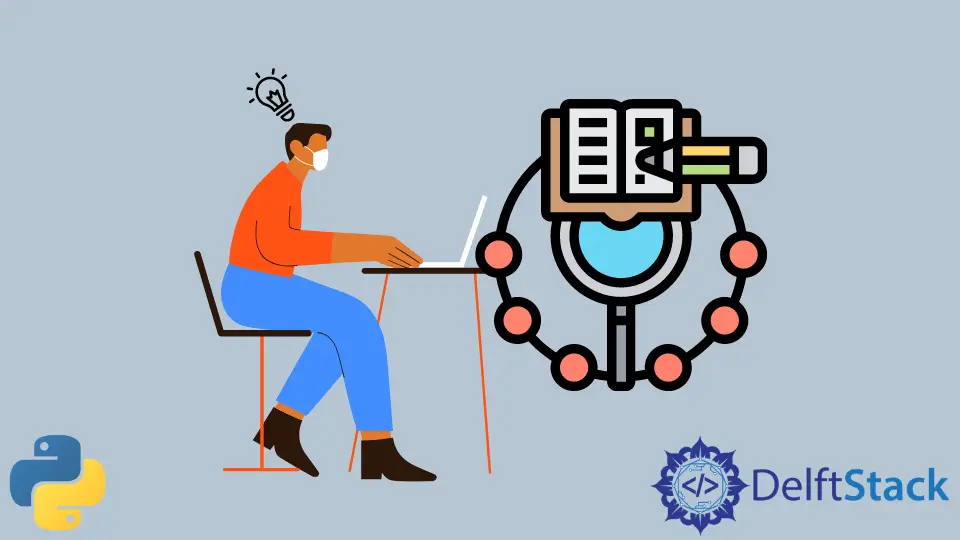
A Python module, package, or library is a file or group of files containing definitions of Python functions, Python classes, and Python variables. In Python, we can import a module and use its implementations to stick with two important concepts of the world of computer science; Don't reinvent the wheel
and Don't repeat yourself
.
These packages or modules can be as small as a few lines and as big as millions of lines. As the size grows, it becomes difficult to analyze modules or see a clear outline of the content of the package. But Python developers have solved that problem for us as well.
In Python, there are many ways in which we can list down methods and classes of a Python module. In this article, we will talk about two such practices with the help of relevant examples. Note that, for instance, we will consider the NumPy
Python module. If you don’t have the NumPy
package on your system or virtual environment, you can download it using either the pip install numpy
or the pip3 install numpy
command.
List All the Methods of a Python Module Using the dir()
Method
The dir()
method is an in-built method in Python. It prints all the attributes and methods of an object or a Python package. Check the following code to understand it.
class A:
a = 10
b = 20
c = 200
def __init__(self, x):
self.x = x
def get_current_x(self):
return self.x
def set_x(self, x):
self.x = x
def __repr__(self):
return f"X: {x}"
print(dir(int))
print(dir(float))
print(dir(A))
Output:
['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__init_subclass__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'as_integer_ratio', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes']
['__abs__', '__add__', '__bool__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getformat__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__int__', '__le__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__pos__', '__pow__', '__radd__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rmod__', '__rmul__', '__round__', '__rpow__', '__rsub__', '__rtruediv__', '__set_format__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', 'as_integer_ratio', 'conjugate', 'fromhex', 'hex', 'imag', 'is_integer', 'real']
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'a', 'b', 'c', 'get_current_x', 'set_x']
We can use the dir()
method to list down all the module methods. Refer to the following code to see how.
import numpy
print("NumPy Module")
print(dir(numpy))
Output:
The getmembers()
function, as the name suggests, gets all the members, such as methods, variables, classes, etc., present inside a class or a module. Since we only require methods or functions, we used the isfunction()
, a Python function that filters out all the functions from the members. There are other such functions as well like below.
ismodule()
: Return True if the object is a module.isclass()
: Return True if the object is a class.istraceback()
: Return True if the object is a traceback.isgenerator()
: Return True if the object is a generator.iscode()
: Return True if the object is a code.isframe()
: Return True if the object is a frame.isabstract()
: Return True if the object is an abstract base class.iscoroutine()
: Return True if the object is a coroutine.
To learn more about this module, refer to the official documentation.