How to Import Files in Python
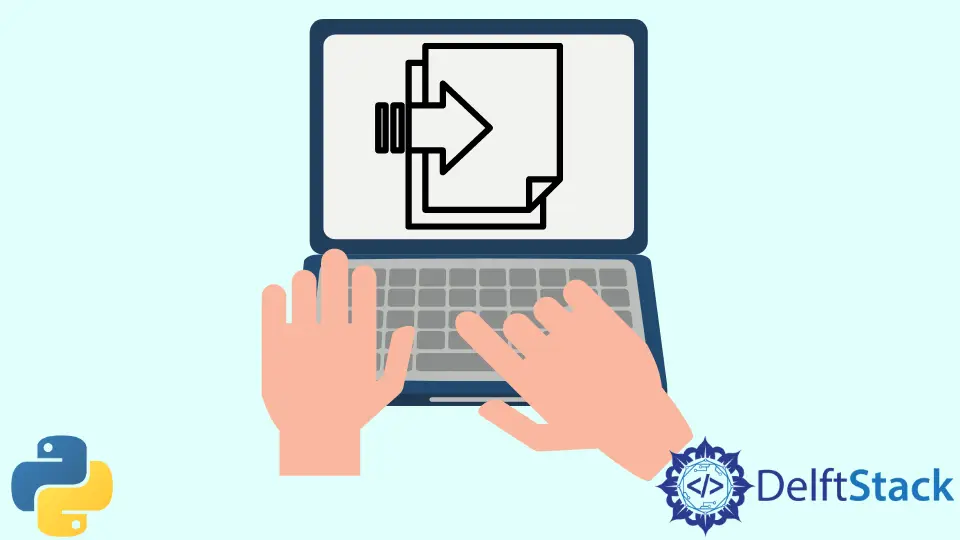
A module is a file consisting of Python code containing functions, classes, and variables.
This article will explain how to import other files or modules in Python.
Import Files in Python
There are many different ways to import another file or module in Python. The rest of the article will explain these methods.
The contents of the test.py file to be used in the examples are below.
def func_from_test():
print("func_from_test called")
def func_from_test_2():
print("func_from_test_2 called")
Use import
to Import the Whole Module
The import
statement finds a module, loads, and initializes. If the as
statement is used, it defines a name in the local namespace for the scope where the import statement occurs.
The use of the as
expression is optional. If not used, the module is named in its original form.
To import multiple modules, you can write them with a comma.
The following program will import all functions in the test.py.
import test as t
t.func_from_test()
t.func_from_test_2()
Use from .. import ..
to Import the Specific Module
The from
with the import
statement finds the module specified in the from clause, loads, and initializes. It checks if the imported module has an attribute with that name, and if the attribute is not found, an ImportError is raised.
The following program will import only the func_from_test
function in the test.py.
from test import func_from_test
func_from_test()
You can use the asterisk (*
) to import all functions.
from test import *
func_from_test()
func_from_test_2()
When using the from
statement, there is no need to use the module’s name when calling the imported function.
Use exec
to Execute Functions From Another Python File
The exec()
function provides dynamic execution of Python code. Suppose a string is given as a parameter; it is parsed as a Python package of statements to be executed later.
The file specified in the open()
function is opened in the example below. The file contents are read with the read()
function and given as a string parameter to the exec()
function.
You can then call the functions in the test.py.
exec(open("test.py").read())
func_from_test()
Use os.system
to Execute a Python File
The system
command is included in the os
module and executes the command given as a string in a subshell. In the following example, the file.py file is executed.
import os
os.system("python test.py")
Import File From a Different Location
The sys.path
statement includes a list of strings that specify modules’ search path. We can add the directory where we want to install the modules to this list.
For this purpose, we use the os.path.abspath()
method. This method returns a normalized absolutized version of the pathname given as a parameter.
Thus, the file in a different location is added to the path, and we can import it with the import
statement.
from test import *
import sys
import os
sys.path.append(os.path.abspath("/home/user/files"))
func_from_test()
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn