How to Import All Modules in One Directory in Python
- Import All Modules Present in One Directory in Python
-
Use
importlib
for Advanced Module Importing - Conclusion
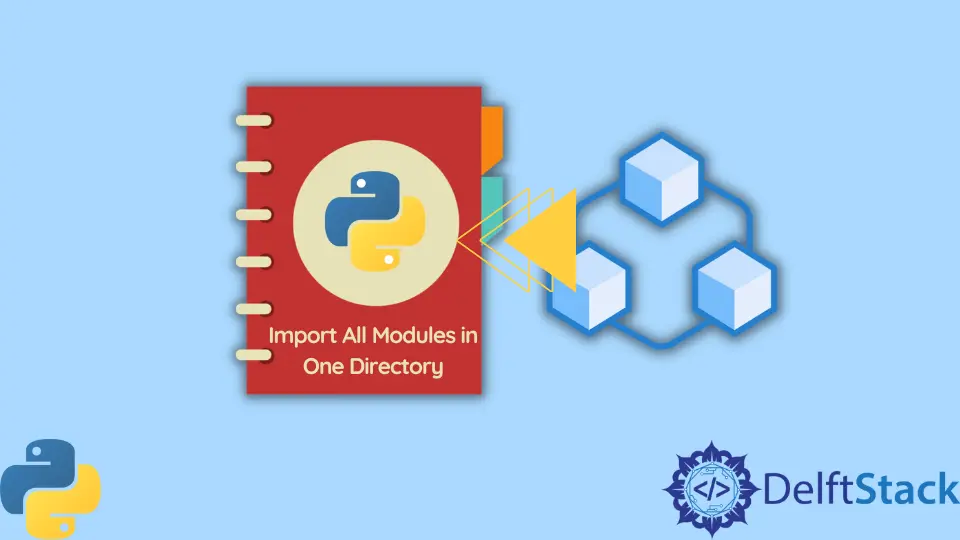
Importing modules is a fundamental aspect of programming in Python, enabling the extension of language capabilities by leveraging various libraries and modules. However, importing modules one by one can be cumbersome and time-consuming.
This tutorial delves into a more efficient approach—importing all modules present in a specific directory in Python.
Import All Modules Present in One Directory in Python
To simplify the process of importing multiple modules from a directory in Python, let’s delve into the directory structure we’ll be working with:
/a
x.py
y.py
z.py
Create the __init__.py
File
To initiate the efficient import of modules from the directory, we need to create a special Python file within the target directory named __init__.py
. This file holds a pivotal role in facilitating module imports.
The __init__.py
file should include the following contents:
import x
import y
import z
Utilize os
to Import Modules
Next, we’ll utilize the os
module to automate the import process for all .py
files present in the directory. This dynamic approach ensures modules are imported without requiring manual imports for each one.
Here’s the code to achieve this:
import os
for module in os.listdir(os.path.dirname(__file__)):
if module == "__init__.py" or module[-3:] != ".py":
continue
__import__(module[:-3], locals(), globals())
del module
When this code snippet is executed, all modules in the directory are imported seamlessly, significantly enhancing the overall efficiency of the Python codebase.
Simplified Access to Imported Modules
Once the modules are imported using the approach above, they can be accessed effortlessly within your code using the namespace a
. Here’s how:
import a
This statement grants access to the modules x
, y
, and z
within the a
namespace, providing a clear and organized structure for utilizing the modules:
a.x
a.y
a.z
Use importlib
for Advanced Module Importing
In newer versions of Python, particularly in the context of Python 3.5 and later, we have the option to leverage the importlib
module for more fine-grained control over imports. This allows us to import modules based on specific conditions, dynamically load modules, and manage the importing and reloading of modules at runtime.
While importlib
provides enhanced capabilities, it may introduce additional complexities, making it essential to carefully weigh the benefits against potential challenges when opting for this approach.
Now, we will discuss how we can import all modules from a directory using the importlib
module.
First, we need to obtain a list of module names within the target directory. We can achieve this by listing all files in the directory and filtering out the Python module files.
import os
directory_path = "/path/to/directory"
# Get a list of all files in the directory
module_files = [f[:-3] for f in os.listdir(directory_path) if f.endswith(".py") and f != "__init__.py"]
Replace "/path/to/directory"
with the actual path to the directory containing the modules you want to import.
Next, we’ll use the importlib
module to import each module dynamically.
import importlib.util
modules = {}
for module_name in module_files:
spec = importlib.util.spec_from_file_location(module_name, os.path.join(directory_path, f"{module_name}.py"))
module = importlib.util.module_from_spec(spec)
spec.loader.exec_module(module)
# Add the module to the modules dictionary
modules[module_name] = module
In this step, we iterate through the module names and use importlib.util.spec_from_file_location
to create a module specification based on the file location. We then use importlib.util.module_from_spec
to create the module from the specification and spec.loader.exec_module
to execute the module.
Now that we’ve imported the modules dynamically, we can access them through the modules
dictionary.
# Access the imported modules and their contents
for module_name, module in modules.items():
# Example: Call a function from the module
module.some_function()
Replace some_function()
with the actual function or attribute you want to use from the modules.
Here’s the complete code that imports all modules from a directory using the importlib
module:
import os
import importlib.util
directory_path = "/path/to/directory"
# Get a list of all files in the directory
module_files = [f[:-3] for f in os.listdir(directory_path) if f.endswith(".py") and f != "__init__.py"]
# Import each module dynamically
modules = {}
for module_name in module_files:
spec = importlib.util.spec_from_file_location(module_name, os.path.join(directory_path, f"{module_name}.py"))
module = importlib.util.module_from_spec(spec)
spec.loader.exec_module(module)
# Add the module to the modules dictionary
modules[module_name] = module
# Access the imported modules and their contents
for module_name, module in modules.items():
# Example: Call a function from the module
module.some_function()
First, this code imports the necessary modules: os
for interacting with the operating system and importlib.util
for dynamically importing modules. The variable directory_path
is set to the path of the directory containing the modules.
Replace "/path/to/directory"
with the actual path to the directory containing the modules you want to import.
Next, it retrieves a list of filenames from the specified directory that end with .py
and are not __init__.py
. It then generates a list of module names by removing the .py
extension from each filename.
Moving on to the dynamic module import, the code iterates through the list of module names. For each module name, it creates a module specification using importlib.util.spec_from_file_location()
based on the module name and its corresponding file path in the specified directory.
Then, it creates a module object using importlib.util.module_from_spec()
and executes the module using spec.loader.exec_module(module)
to import it dynamically. Each imported module is stored in a dictionary called modules
, with the module name as the key and the module object as the value.
In the last part, it iterates over the imported modules stored in the modules
dictionary. For each module, it demonstrates how to call a function (some_function()
in this example) defined in the module.
The code doesn’t include the specific function to be called, so some_function()
is a placeholder for any function defined in each module.
It’s important to use these capabilities judiciously and understand the potential complexities that may arise with dynamic imports.
Conclusion
In conclusion, importing modules from a directory in Python can be streamlined by creating an __init__.py
file and utilizing the os
module to automate the import process. This efficient approach enables easy access to the modules within the code, promoting a more organized and manageable codebase.
Additionally, exploring advanced options like the importlib
module can further enhance the import mechanism, albeit with a consideration of the complexities involved.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Import
- Python Circular Import
- How to Import All Functions From File in Python
- How to Import a Variable From Another File in Python
- How to Import a Module From a Full File Path in Python
- How to Import Modules From Parent Directory in Python