How to Import All Functions From File in Python
-
Use the
import *
Statement to Import All Functions From a File in Python -
Reason to Not Use the
import *
Approach in Python -
Advantages of Using
import *
in Python -
Potential Pitfalls and Best Practices of Using
import *
in Python - Conclusion
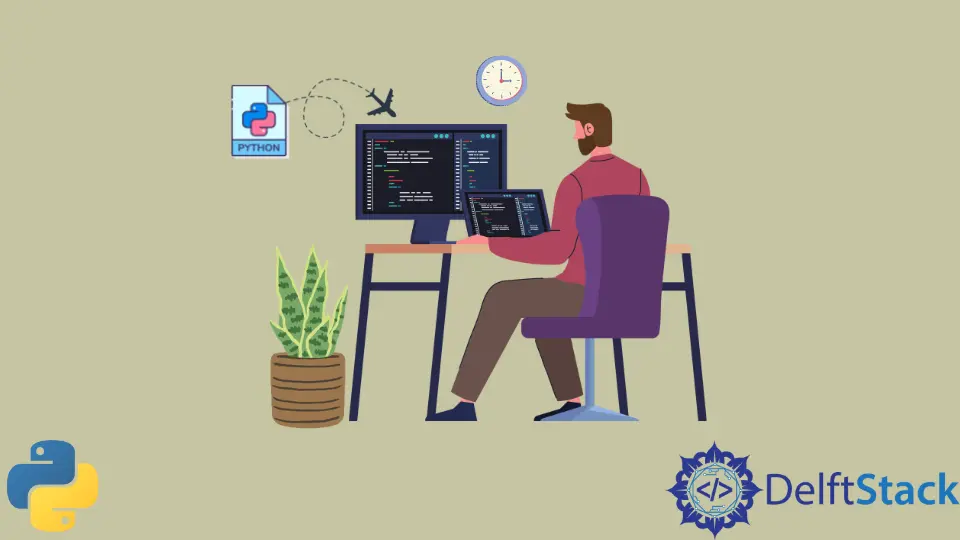
Python’s import *
statement is a powerful tool for bringing all functions and variables from an external file into your current script. While convenient, it’s essential to use it wisely to avoid namespace collisions and maintain code readability.
This tutorial will discuss the complexity of using import *
to import all the functions from a file in Python.
Use the import *
Statement to Import All Functions From a File in Python
The import
statement is used to import packages, modules, and libraries in our Python code. If you want to import all functions from a file, you can use the import *
statement.
The import *
statement allows you to import all functions, classes, and variables from a module or file. This means that you can use these functions and variables directly without having to prefix them with the module name.
It is a concise way of importing all functions and classes from a module or file in Python. This statement instructs Python to bring in everything from the specified file, thereby making all functions and classes available in the current namespace.
Syntax:
from module_name import *
Here, module_name
is the name of the Python file (without the .py
extension) that you want to import from.
The following code snippets show a working implementation of this approach. In this example, we have a file named my_functions.py
that contains two functions: greet(name)
and farewell(name)
.
File: my_functions.py
def greet(name):
return f"Hello, {name}!"
def farewell(name):
return f"Goodbye, {name}!"
In the main script, we use from my_functions import *
to import all functions from my_functions.py
. This allows us to directly call greet()
without prefixing it with my_functions
.
File: main.py
# Main script
from my_functions import *
message = greet("Alice")
print(message)
Since we call the greet()
function in the main script from my_functions.py
, it will print Hello, Alice!
.
Output:
Hello, Alice!
Reason to Not Use the import *
Approach in Python
This approach uses an implicit import
statement, whereas in Python, we are always advised to use explicit import
statements.
According to the Zen of Python, “Explicit is better than implicit”. There are two key reasons for this statement.
The first reason is that it is very hard to understand which function is coming from which file as the size of the project increases, and we end up importing functions from multiple files. It is especially hard for someone else to read our code and fully understand what is happening.
It makes our code very hard to debug and maintain. This problem is highlighted in the following code snippet.
from functions import *
from functions1 import *
from functions2 import *
print(square(2))
Output:
4
In the above code snippet, it’s impossible to know where the original square()
function is defined by just looking at the code. To fully understand the origins of the square()
function, we have to explore all the files manually.
The second key reason is that if we have two functions with the same name in multiple files, the interpreter will use the most recent import
statement. This phenomenon is demonstrated in the following code snippet.
from functions2 import *
from functions import *
print(hello())
print(hello())
print(hello())
Output:
hello from functions
hello from functions2
hello from functions2
The two files, functions.py
and functions2.py
, both contain a hello()
function.
In the first line of output, we imported the functions.py
file, and hence the hello()
function inside this file is executed. In the second and third lines of output, we have also imported the functions2.py
file, containing a hello()
function.
So, the new hello()
function is executed in the last two output lines.
Advantages of Using import *
in Python
-
Conciseness and Readability
Using
import *
can significantly reduce the number of lines of code needed to import multiple functions or classes. This can lead to cleaner, more readable code.from module_name import func1, func2, func3 # Multiple imports
VS.
from module_name import * # All functions are imported at once
-
Simplifies Access
All functions and classes are directly accessible in the current namespace, which means you can use them without having to prefix them with the module name.
# Without import * from module_name import function_name # With import * function_name() # No need to use module_name.function_name()
-
Efficient for Interactive Sessions
In interactive Python sessions, such as in the Python shell or Jupyter notebooks,
import *
can be invaluable for quick experimentation with the functions in a module. It saves you from repeatedly typing out individual function names.from module_name import *
This can be a time-saver during exploratory data analysis and debugging.
Potential Pitfalls and Best Practices of Using import *
in Python
While import *
offers conveniences, it’s important to be aware of potential pitfalls and use it judiciously. Here are some considerations and best practices:
-
Name Conflicts
Importing all functions and classes may lead to name conflicts if there are identically named functions in different modules. To avoid this, consider using more specific import statements or aliasing functions.
```python
from module_name import func1, func2 # Import only what's needed
```
-
Reduced Readability
While
import *
can make code concise, it might also reduce code readability. Future maintainers may find it challenging to determine the source of a particular function or class. -
Avoid in Production-Level Code
In production-level code, it’s generally recommended to be explicit about which functions and classes you’re importing. This makes code more understandable and helps prevent unexpected behavior due to name conflicts.
-
Use With Caution
While
import *
can be convenient, it’s not always the best choice. Consider using it selectively and only in situations where it genuinely improves code readability and maintainability.
Conclusion
While using import *
can be convenient, it’s important to exercise caution. The approach can lead to code that is harder to understand and maintain as projects grow in size. Additionally, potential namespace collisions and lack of clarity regarding the origin of functions can introduce unexpected behavior.
As a general best practice, it’s recommended to use explicit import
statements, specifying the functions or variables you need from a module. This provides clarity in code and avoids potential conflicts.
By adhering to the principle of “Explicit is better than implicit”, you can write more readable, maintainable, and error-resistant Python code. Remember, code should not only work correctly but also be easy for others (and yourself in the future) to comprehend.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn