How to Import a Module From a Full File Path in Python
- Import a Module With a Full File Path in Python 3.5+
- Import a Module With a Full File Path in Python 3.3 and 3.4
- Import a Module With a Full File Path in Python 2
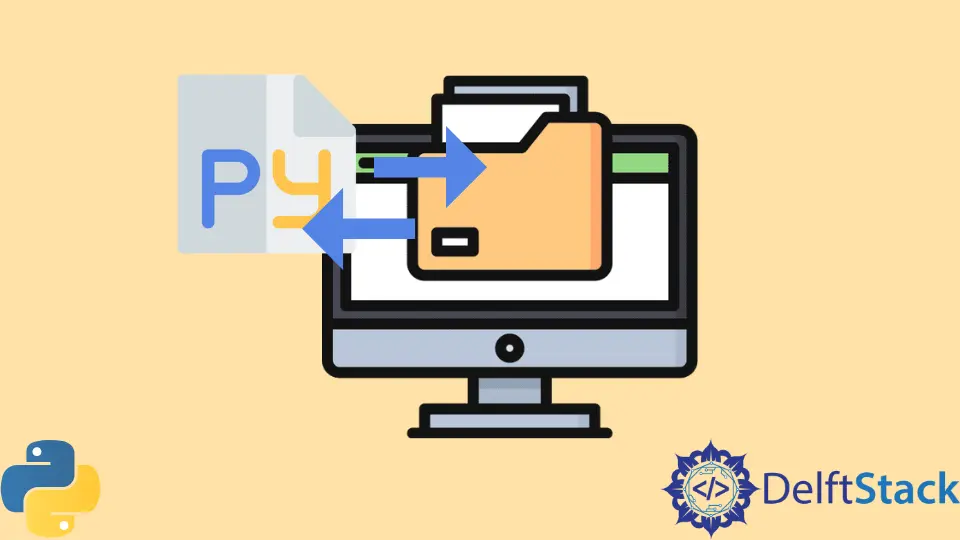
To import a Python file from a given path, use the Python libraries based on the Python version. This article explains how to import a module given the full path to the module in Python.
Use the following libraries’ functions based on the Python version to import modules.
- For Python 3.5+, import
importlib.util
. - For Python 3.3 and 3.4, import
importlib.machinery
,SourceFileLoader
. - For Python 2, import
imp
.
Before we begin, please get the file setup ready to demonstrate the examples.
- Mention the following code in the
addmodule.py
file.
def printingstatement():
print("From addmodule.py")
- Place
addmodule.py
inside a folder. In the examples below, it is insidec:\\Users\\Rexjohn\\Folder-1\\
.
Import a Module With a Full File Path in Python 3.5+
For Python 3.5+, use the importlib.util
library’s functions to import a module:
import importlib.util
MODULE_PATH = "c:\\Users\\Rexjohn\\Folder-1\\addmodule.py"
MODULE_NAME = "addmodule"
spec = importlib.util.spec_from_file_location(MODULE_NAME, MODULE_PATH)
modulevar = importlib.util.module_from_spec(spec)
spec.loader.exec_module(modulevar)
modulevar.printingstatement()
Here, use the following functions from the importlib.util
library.
spec_from_file_location(MODULE_NAME, MODULE_PATH)
. Please mention the full file path of the module nameMODULE_NAME
inMODULE_PATH
.module_from_spec(spec)
exec_module(modulevar)
Output:
From addmodule.py
Import a Module With a Full File Path in Python 3.3 and 3.4
If you’re working with Python 3.3 and 3.4, you can use the importlib.machinery
, SourceFileLoader
library’s functions to import a module:
from importlib.machinery import SourceFileLoader
MODULE_PATH = "c:\\Users\\Rexjohn\\Folder-1\\addmodule.py"
MODULE_NAME = "addmodule"
modulevar = SourceFileLoader(MODULE_NAME, MODULE_PATH).load_module()
modulevar.printingstatement()
Here, use the following functions from the importlib.machinery
, SourceFileLoader
libraries by mentioning.
SourceFileLoader(MODULE_NAME, MODULE_PATH).load_module()
Output:
From addmodule.py
Import a Module With a Full File Path in Python 2
For Python 2, use the imp
library’s functions to import a module:
import imp
MODULE_PATH = "c:\\Users\\Rexjohn\\Folder-1\\addmodule.py"
MODULE_NAME = "addmodule"
modulevar = imp.load_source(MODULE_NAME, MODULE_PATH)
modulevar.printingstatement()
Here, use the following function from the imp
library.
load_source(MODULE_NAME, MODULE_PATH)
Output:
From addmodule.py