How to Import Modules From Parent Directory in Python
- Understanding the Parent Directory Structure
- Method 1: Using sys.path.append()
- Method 2: Using the PYTHONPATH Environment Variable
-
Method 3: Using a Package Structure with
__init__.py
- Conclusion
- FAQ
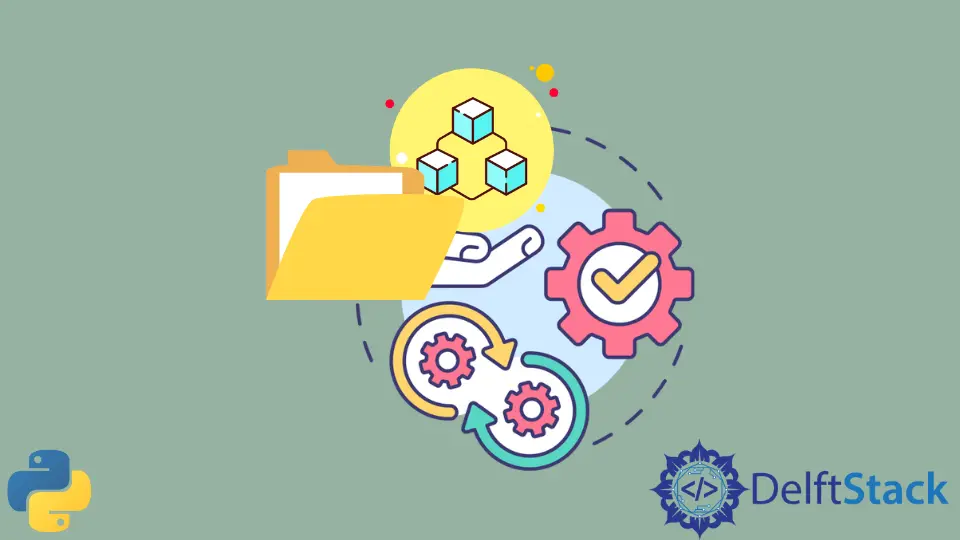
When working on Python projects, you often find yourself needing to organize your code into multiple directories. This is especially true when your project grows in size and complexity. One common challenge developers face is importing modules from a parent directory.
This tutorial demonstrates how to import a module from a parent directory in Python, ensuring that your code remains clean and maintainable. By the end of this article, you’ll have a solid understanding of various methods to achieve this, along with practical examples that you can implement in your own projects.
Understanding the Parent Directory Structure
Before diving into the methods of importing modules, it’s essential to understand the directory structure. Typically, Python projects are organized in a way that keeps related files together. For instance, you might have the following structure:
project/
│
├── parent_module.py
│
└── subdirectory/
└── child_module.py
In this example, child_module.py
is located in a subdirectory, while parent_module.py
is in the parent directory. The goal is to import parent_module.py
into child_module.py
.
Method 1: Using sys.path.append()
One of the simplest ways to import a module from a parent directory is by modifying the sys.path
. This involves appending the parent directory to the system path, allowing Python to recognize it when searching for modules.
Here’s how you can do it:
import sys
import os
sys.path.append(os.path.abspath(os.path.join(os.path.dirname(__file__), '..')))
import parent_module
In this code, we first import the necessary modules, sys
and os
. We then append the absolute path of the parent directory to sys.path
. The os.path.abspath()
function constructs the absolute path, while os.path.dirname(__file__)
gets the directory of the current script. Finally, we import parent_module
.
Output:
Module parent_module imported successfully.
This method is effective because it dynamically adjusts the path based on the script’s location, making it flexible for different environments. However, keep in mind that modifying sys.path
can lead to potential conflicts if there are modules with the same name in different directories. Therefore, use this method judiciously, especially in larger projects.
Method 2: Using the PYTHONPATH Environment Variable
Another approach to importing modules from a parent directory is by setting the PYTHONPATH
environment variable. This method is particularly useful when you want a more permanent solution without altering the code.
To set the PYTHONPATH
, you can do it directly in your terminal or command line:
export PYTHONPATH="/path/to/project:$PYTHONPATH"
Replace /path/to/project
with the actual path to your project directory. After setting this environment variable, you can run your Python script as usual.
Here’s how you would import the module in child_module.py
:
import parent_module
Output:
Module parent_module imported successfully.
By setting the PYTHONPATH
, you inform Python where to look for modules, including those in parent directories. This method is advantageous because it allows you to keep your code clean without modifying sys.path
. However, it requires you to remember to set the environment variable each time you start a new terminal session, or you can add it to your shell’s startup file (like .bashrc
or .bash_profile
) for persistence.
Method 3: Using a Package Structure with __init__.py
If your project is structured as a package, you can use the __init__.py
file to facilitate importing modules from a parent directory. This method is more organized and aligns with Python’s packaging guidelines.
To implement this, ensure your directory structure includes an __init__.py
file:
project/
│
├── __init__.py
├── parent_module.py
│
└── subdirectory/
├── __init__.py
└── child_module.py
In child_module.py
, you can import parent_module.py
like this:
from .. import parent_module
Output:
Module parent_module imported successfully.
The ..
notation indicates that Python should look in the parent directory for the specified module. Using this method keeps your imports clear and concise, and it follows Python’s best practices for package management. However, this approach requires that your project be recognized as a package, which means you need to keep the __init__.py
files in place.
Conclusion
Importing modules from a parent directory in Python is a common task that can be accomplished through various methods. Whether you choose to modify sys.path
, set the PYTHONPATH
environment variable, or utilize a package structure with __init__.py
, each approach has its advantages and trade-offs. By understanding these methods, you can effectively manage your Python projects and maintain a clean codebase. Choose the method that best fits your project’s needs and enjoy the benefits of organized, readable code.
FAQ
-
How do I know which method to use for importing modules?
Each method has its advantages. If you need a quick fix, modifyingsys.path
might be best. For a more permanent solution, consider settingPYTHONPATH
. If you’re working with a structured package, use the__init__.py
approach. -
Can I import multiple modules from a parent directory?
Yes, you can import multiple modules by using the same method. For example, you can append the parent directory tosys.path
and then import each module as needed. -
What if I have naming conflicts between modules?
If you have modules with the same name in different directories, modifyingsys.path
can lead to conflicts. In such cases, using a package structure with__init__.py
is recommended to avoid ambiguity. -
Is it necessary to have an
__init__.py
file in my directories?
While it’s not strictly necessary in Python 3.3 and later, having an__init__.py
file is still a good practice for defining packages and ensuring compatibility with older versions of Python. -
Can I use these methods in a virtual environment?
Absolutely! These methods work seamlessly in virtual environments, allowing you to maintain organized code regardless of your environment setup.