The fnmatch Module in Python
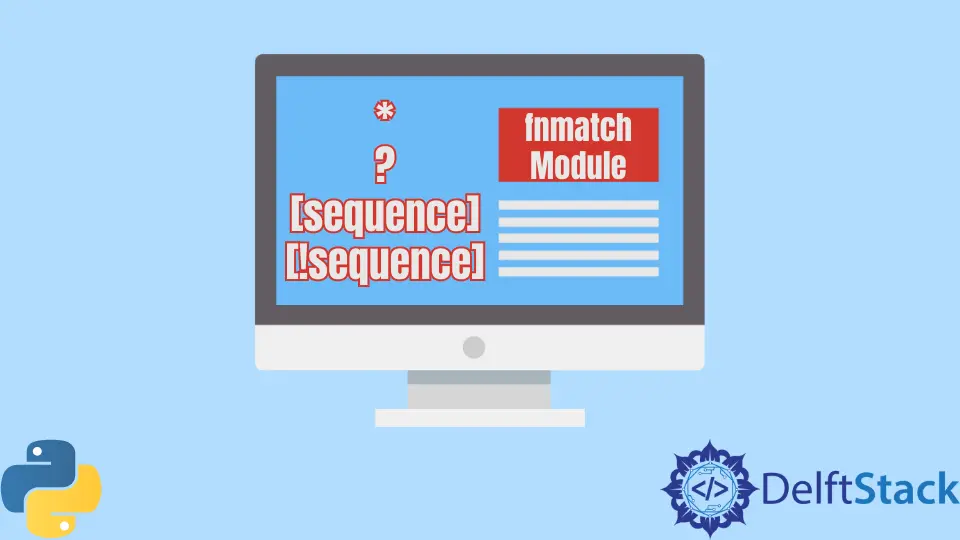
When working with files and file systems, frequently, one has to find several files from a heap of files. Finding the required files from a pile of files will take forever if performed manually.
Hence, operating systems and programming languages offer utilities to find required files dynamically. These utilities tend to target the filenames and try to find the necessary files with the help of pattern matching.
In a UNIX-based
operating system such as macOS and Linux, one can locate files with the help of the fnmatch
library found in the Python programming language.
This article will learn how to perform pattern matching using Python’s fnmatch
library.
the fnmatch
Module in Python
The fnmatch
module is used to match UNIX
operating system shell-style wildcards. Note that these styles are not regex
or regular
expressions.
Following are the special characters that are used in UNIX shell-style wildcards
:
Pattern | Operation |
---|---|
* |
Matches everything |
? |
Matching a single character |
[sequence] |
Matches any character in a sequence |
[!sequence] |
Matches any character, not in a sequence |
The fnmatch
library has the following methods:
- The
fnmatch.fnmatch(filename, pattern)
is thefnmatch()
method, that matches a filename with the specified pattern. If the pattern matches, this returnsTrue
; otherwise,False
. Note that this method is case insensitive, and both the parameters are normalized to lowercase with the help of theos.path.normcase()
method. - The
fnmatch.fnmatchcase(filename, pattern)
- is very similar to thefnmatch()
method but it is case sensitive and does not apply theos.path.normcase()
method to the parameters. - The
fnmatch.filter(names, pattern)
creates a list of filenames that match the specified pattern. This method is similar to iterating over all the filenames and performing thefnmatch()
method but implemented more efficiently. - The
fnmatch.translate(pattern)
converts the shell-style pattern to a regex or regular expression with the help of there.match()
method.
Now that we have looked at some theory, let us understand how to use this library practically with the help of a relevant example.
The example filters all the files that end with the .html
extension.
import os
import fnmatch
for file in os.listdir("."):
if fnmatch.fnmatch(file, "*.html"):
print(file)
Output:
<files with ".html" extension in the current working directory>
The Python code above first reads all the files in the current working directory with the help of the os.listdir()
method. Next, it iterates over all the files and checks if they are HTML files using the fnmatch()
method.
Here, the *.html
pattern matches all the files that end with .html
. Here, *
refers to any number of characters in the filename.
Let us look at another example that filters all the files that start with hello
and end with .js
. Refer to the following Python code for the same.
import os
import fnmatch
for file in os.listdir("."):
if fnmatch.fnmatch(file, "hello*.js"):
print(file)
Output:
<files with filenames of type "hello*.js" in the current working directory>