Type Hints in Python
- What Are Type Hints in Python
- How to Add Type Hints to Python Variables
- How to Add Type Hints to Functions in Python (Function Annotations)
- Typing Module in Python
-
How to Use Type Hints With
mypy
Library in Python - Why Should I Use Type Hint in Python
- Why Should I Avoid Type Hints in Python
- How to Use Type Comments in Python
- Conclusion
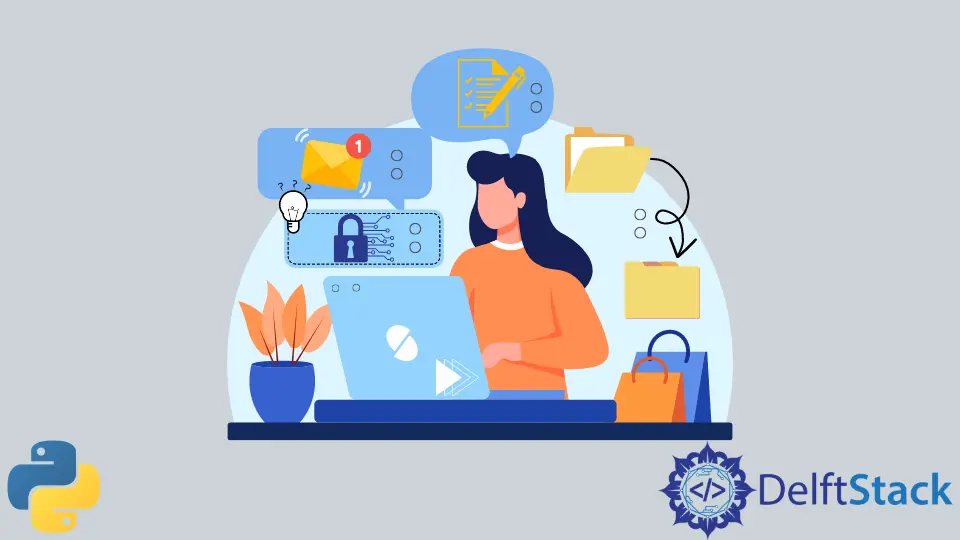
Type Hints are a new feature released with Python 3.5 that allows you to indicate the variable’s data type within your code statically.
Python language is dynamically typed, leading to bugs in particular cases and unnecessary coding problems in longer programs.
Inferring or checking the type of an object is difficult due to the dynamic nature of the Python language, which is solved by Python’s type hints.
What Are Type Hints in Python
Specifying data types in a new concept for users in Python. Data types are a popular notion in C, C++, and Java.
The static nature of such programming languages means that the compiler performs type checks before executing the code.
Python’s dynamic nature means it is compiled at runtime. PEP 484
introduced type hints in Python for static type checking of the code. Type Hints in Python define the data type of variables and return type of functions.
How to Add Type Hints to Python Variables
You can quickly add type hints to variables by declaring the variable followed by :
and the data type in the following manner. You can also initiate the variable’s value after mentioning the data type.
val1: float = 4.71
print(val1)
val2: str = "Example of String"
print(val2)
val3: bool = True
print(val3)
lis1: list = ["p", "q", "r"]
print(lis1)
tup1: tuple = (47, 55, 30)
print(tup1)
dic1: dict = {"John": 98, "Frank": 99, "Lisa": 100}
print(dic1)
Output:
4.71
Example of String
True
['p', 'q', 'r']
(47, 55, 30)
{'John': 98, 'Frank': 99, 'Lisa': 100}
How to Add Type Hints to Functions in Python (Function Annotations)
You can easily add type hints to any function by adding a :
after your variable and specifying the data type. You specify the return data type by adding ->
after defining a function in the following manner.
def product(no1: int, no2: int) -> int:
return no1 * no2
print(product(6, 4))
Output:
24
Function annotations were provided in Python 3.0 and used by type hints to provide return values to methods. The function’s execution is not hindered by adding a return data type.
You can use None
for functions that do not return anything. You can use Union
from the typing
module to specify more than one data type.
Typing Module in Python
In Python, type hints offer increased functionality with the typing
module introduced in Python 3.5. Python’s typing
module allows you to specify data types explicitly.
You can define a tuple
of integers, a list
of floats, and more efficiently, as shown below.
from typing import List, Tuple, Dict
lis1: List[int] = [70, 18, 29]
print(lis1)
tup1: Tuple[float, int, int] = (21.52, 2, 3)
print(tup1)
dic1: Dict[int, str] = {1: "Type Hints", 2: "Python"}
print(dic1)
Output:
[70, 18, 29]
(21.52, 2, 3)
{1: 'Type Hints', 2: 'Python'}
The typing
module provides support for type hints, including:
Type Aliases
Type Aliases allows you to specify a word that you can use as an alias interchangeably to simplify code, as shown.
Vector = List[float]
NewType
The NewType
assistance class makes it simple to spot logical flaws.
RollNo = NewType("RollNo", int)
Generics
Abstract base classes have been enhanced to support subscriptions to indicate anticipated types for container components since type information about objects held in containers cannot be statically deduced generically.
X = TypeVar("X")
Any
Type
Any
type is a special data type compatible with every other type. Any
is used when your code requires both dynamic and static lines to indicate that the variable needs a dynamically typed value.
x: Any = "Python"
Union
The Union
operator allows you to accept and return the specified data types without showing any warnings. You can also select more than one type of data type in Python using the Union
operator.
def numsqr(n1: Union[float, int]) -> Union[float, int]:
return n1 ** 2
You can define Union easily in Python 3.10 and newer versions by using |
, as shown below.
def numsqr(n1: float | int) -> float | int:
return n1 ** 2
How to Use Type Hints With mypy
Library in Python
Python’s mypy
library allows you to force type checks at runtime. PEP 484
does not enforce any constraints for using type hints and only provides directions and guidelines to be followed for performing type checks.
All code snippets run smoothly, regardless of the number or presence of annotations or type hints, as Python does not use them. You can install the mypy
library using pip
or conda
.
pip install mypy
conda install mypy
Using the mypy
library can help make your coding easier by performing type checks and giving warnings at runtime. Mypy
throws a warning explaining the wrong usage of a data type at a specific line, as shown below.
def findsqr(n1: int) -> int:
return n1 ** 2
if __name__ == "__main__":
print(findsqr(4))
print(findsqr(4.5))
Output without mypy
:
16
20.25
Output with mypy
:
main.py:5: error: Argument 1 to "findsqr" has incompatible type "float"; expected "int"
Found 1 error in 1 file (checked 1 source file)
Why Should I Use Type Hint in Python
Python’s mypy
library and type hints provide several boons for developers, including:
- Code Documentation - Type hints allow for better documentation of your code so others who refer to it can get it quickly as data types for variables and functions are already specified.
- Increased IDE and Tool Support - If you have used type hints in your code, IDEs (Integrated Development Environments) can provide better code completion suggestions, methods, and attributes.
- Easy Debugging - Type hints in Python make finding and correcting errors easier as mypy can pinpoint the bug’s exact location. Type hints also create a clean flow for big code blocks in large projects, providing a more pristine architecture.
Why Should I Avoid Type Hints in Python
Python’s type hints provide many advantages for developers, but has some drawbacks as well, such as:
- Increased Coding - Additional lines of code, especially containing variables, will be included in type hints, resulting in a greater effort and code length.
- Unsupported Versions - Type hints provide an excellent experience with Python 3.5, or later as older versions of Python (Before Python 3) do not run variable annotations. Type comments can be used in older versions of Python to use variable annotations and type hints.
How to Use Type Comments in Python
Type comments in Python are special comments that allow you to add type hints in older versions using comments not available in the annotations dictionary.
The syntax for type comments is below.
def circle_area(r):
# type: (float) -> float
return 3.14 * r * r
As shown above, you can add type comments in the older versions as a compromise, but Python recommends using type hints.
Conclusion
We have discussed Type Hints and the typing module in Python in this text. Type Hints provide the advantage of other programming languages by providing static code checking.
Type Hints in Python are an excellent way of improving your code’s documentation and readability. Type Hints also assist you greatly while debugging your code and allow others to understand it well.
However, type hints can make your code longer, which is avoided as Python is known for its concise and straightforward structure.
Type hints provide a cleaner approach towards coding and allow you to define the architecture of your code and functions better.