列出 Python 模块的所有方法
Vaibhav Vaibhav
2024年2月15日
Python
Python Module
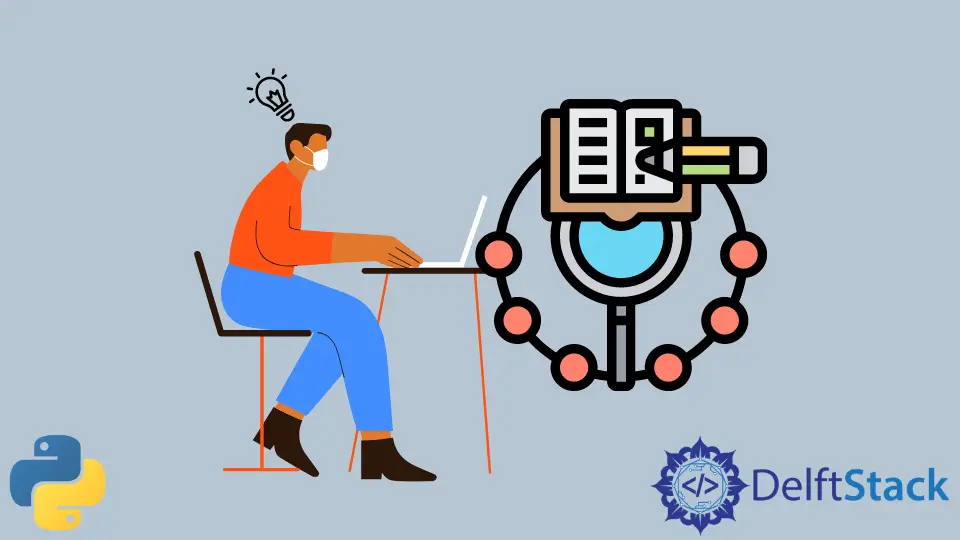
Python 模块、包或库是一个文件或一组文件,其中包含 Python 函数、Python 类和 Python 变量的定义。在 Python 中,我们可以导入一个模块并使用它的实现来坚持计算机科学世界的两个重要概念; 不要重新发明轮子
和不要重复自己
。
这些包或模块可以小到几行,大到数百万行。随着大小的增长,分析模块或查看包内容的清晰轮廓变得困难。但是 Python 开发人员也为我们解决了这个问题。
在 Python 中,我们可以通过多种方式列出 Python 模块的方法和类。在本文中,我们将借助相关示例来讨论两种此类实践。请注意,例如,我们将考虑 NumPy
Python 模块。如果你的系统或虚拟环境中没有 NumPy
软件包,你可以使用 pip install numpy
或 pip3 install numpy
命令下载它。
使用 dir()
方法列出 Python 模块的所有方法
dir()
方法是 Python 中的内置方法。它打印对象或 Python 包的所有属性和方法。检查以下代码以了解它。
class A:
a = 10
b = 20
c = 200
def __init__(self, x):
self.x = x
def get_current_x(self):
return self.x
def set_x(self, x):
self.x = x
def __repr__(self):
return f"X: {x}"
print(dir(int))
print(dir(float))
print(dir(A))
输出:
['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__init_subclass__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'as_integer_ratio', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes']
['__abs__', '__add__', '__bool__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getformat__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__int__', '__le__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__pos__', '__pow__', '__radd__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rmod__', '__rmul__', '__round__', '__rpow__', '__rsub__', '__rtruediv__', '__set_format__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', 'as_integer_ratio', 'conjugate', 'fromhex', 'hex', 'imag', 'is_integer', 'real']
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'a', 'b', 'c', 'get_current_x', 'set_x']
我们可以使用 dir()
方法列出所有模块方法。请参阅以下代码以了解如何操作。
import numpy
print("NumPy Module")
print(dir(numpy))
输出:
正如我们所见,dir()
方法列出了所有 NumPy
方法。
使用 inspect()
模块列出 Python 模块的所有方法
inspect
模块是一个 Python 模块,它有几个有用的函数来获取有关模块、类、类对象、函数、回溯和对象的有用信息。我们可以将此模块用于我们的简单目的。参考以下代码了解其用法。
import numpy
from inspect import getmembers, isfunction
print("NumPy Module")
print(getmembers(numpy, isfunction))
输出:
getmembers()
函数,顾名思义,获取存在于类或模块中的所有成员,例如方法、变量、类等。由于我们只需要方法或函数,因此我们使用了 isfunction()
,这是一个 Python 函数,可以从成员中过滤掉所有函数。还有其他类似的功能,如下所示。
ismodule()
:如果对象是模块,则返回 True。isclass()
:如果对象是一个类,则返回 True。istraceback()
:如果对象是回溯,则返回 True。isgenerator()
:如果对象是生成器,则返回 True。iscode()
:如果对象是代码,则返回 True。isframe()
:如果对象是框架,则返回 True。isabstract()
:如果对象是抽象基类,则返回 True。iscoroutine()
:如果对象是协程,则返回 True。
要了解有关此模块的更多信息,请参阅官方文档。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav