How to Resolve KeyError 0 in Python
-
the
KeyError 0
Exception in Python -
Use the
try-except
Block to ResolveKeyError 0
in Python -
Use
dict.get()
to ResolveKeyError 0
in Python -
Use
if-else
to ResolveKeyError 0
in Python
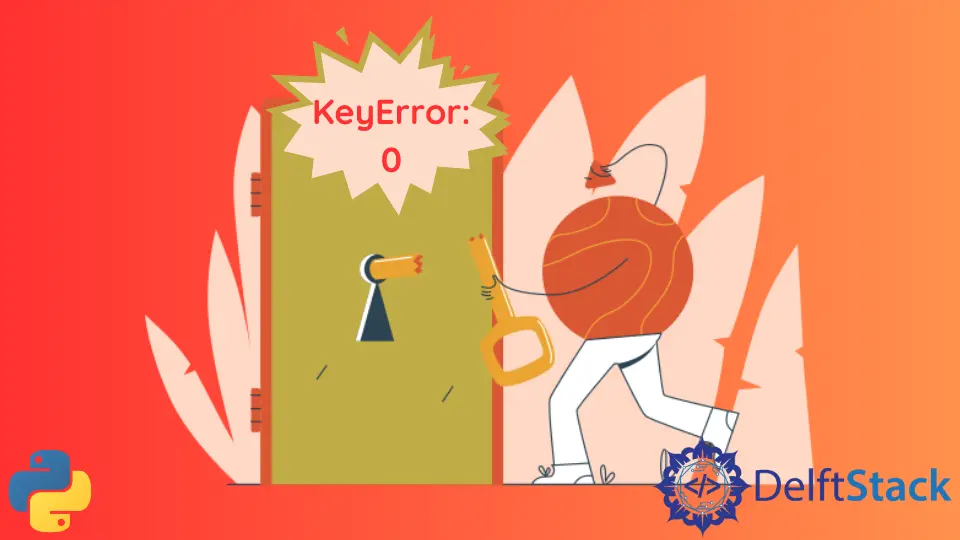
Every programming language encounters many errors. Some occur at the compile time, some at run time.
A KeyError
is a run time error in which the code syntax is correct, but it will throw an error during the execution of the code.
the KeyError 0
Exception in Python
KeyError 0
occurs in Python’s run time when the mapping key 0 is not defined, which we try to access. The mapping key will throw a KeyError 0
exception.
A map is a kind of data structure that maps one value to another by a colon :
. The dictionary is the most common type of mapping.
In the below example, only three keys with their corresponding students’ names are defined in the dictionary. If we try to access any other key in the dictionary, it will throw a KeyError
.
Example Code:
# Python 3.x
dict = {1: "kelvin", 2: "Ron", 3: "James"}
print(dict[0])
Output:
#Python 3.x
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
<ipython-input-1-f0366e7afb22> in <module>()
1 dict = {1: 'kelvin', 2:'Ron' , 3:'James'}
----> 2 print(dict[0])
KeyError: 0
Here, when we access the key 0
in the dictionary, it will generate a KeyError 0
because the key 0
is not present.
There are multiple ways to resolve KeyError 0
in Python. Below are some of the ways with their explanation and code.
Use the try-except
Block to Resolve KeyError 0
in Python
In this example, 0
is not defined in dict, but it will not give a KeyError 0
exception because the try-except
block handles it. Instead of an error, it will print a statement in the exception block.
Example Code:
# Python 3.x
dict = {3: "Kelvin", 5: "James", 6: "Danial"}
try:
print(dict[0])
except KeyError:
print("key not present in the dictionary")
Output:
#Python 3.x
key not present in the dictionary
Use dict.get()
to Resolve KeyError 0
in Python
The get()
method effectively handles KeyError
. Using get()
, we can return a default value or a message for the key that is not present in the dictionary.
In this example, key 0
does not exist, so it will print the default set statement: key 0 does not exist in dictionary
. The method will return None
if we do not provide a default value.
Example Code:
# Python 3.x
dict = {3: "Kelvin", 5: "James", 6: "Danial"}
print(dict.get(0, "key 0 does not exist in dictionary"))
Output:
#Python 3.x
key 0 does not Exist in dictionary
Use if-else
to Resolve KeyError 0
in Python
Before accessing the key 0
, we can check whether the key is present in the dictionary or not by using the for
loop and if-else
statement, as shown in the example. It will only print the values in the dictionary against the key.
Example Code:
# Python 3.x
dict = {3: "Kelvin", 5: "James", 6: "Danial"}
for i in range(1, 7):
if i in dict:
print(dict[i])
else:
continue
Output:
#Python 3.x
Kelvin
James
Danial
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python