How to Fix Python ValueError: Invalid Literal for Float()
-
the
ValueError: invalid literal for float()
in Python -
Fix the
ValueError: invalid literal for float()
in Python
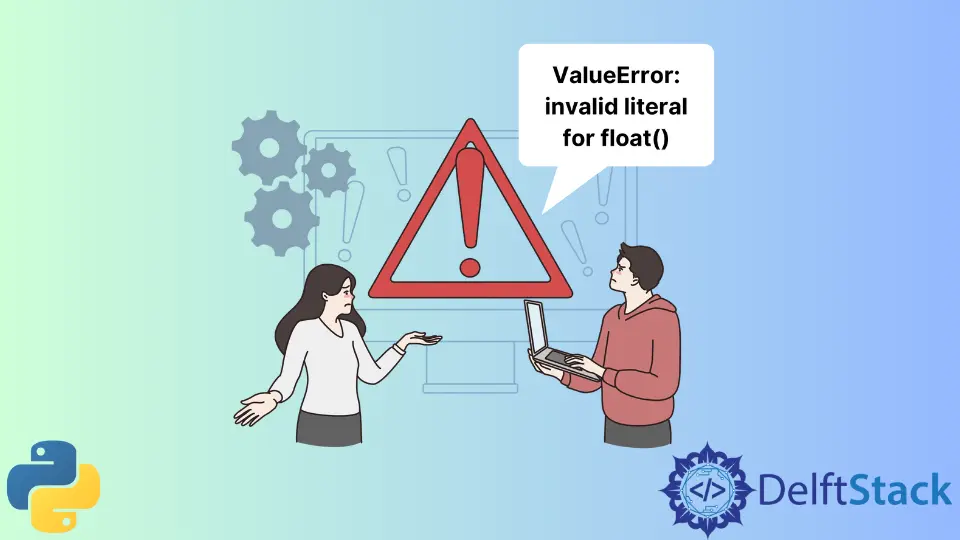
When you pass an unrecognized argument that isn’t supported by the float()
function, the Python compiler will arise an error such as ValueError: invalid literal for float()
. The python compiler arises this ValueError: invalid literal for float()
in Python 2x versions and ValueError: could not convert string to float
in Python 3x versions when you pass the string value as an argument to the float()
function.
the ValueError: invalid literal for float()
in Python
The float()
function isn’t capable of typecasting a string into a floating point number. Rather it throws a ValueError which can be different depending on your Python version.
Let’s see an example for both Python 2
and Python 3
.
Code - Python 2
:
# python 2.7
import sys
print("The Current version of Python:", sys.version)
x = "123xyx"
y = float(x)
print(y)
Output:
The current version of Python: 2.7.18
ValueError: invalid literal for float(): 123xyx
Code - Python 3
:
# >= Python 3.7
import sys
print("The Current version of Python:", sys.version)
x = "123xyx"
y = float(x)
print(y)
Output:
The current version of Python: 3.7.4
ValueError: could not convert string to float: '123xyx'
We ran the same code in Python 2.7
and Python 3.7
, but the error isn’t the same because of improvement and continuous development of Python’s compiler.
Fix the ValueError: invalid literal for float()
in Python
To fix the value error invalid literal for float()
or could not convert string to float
, you need to provide a valid literal as an argument to the float()
function so it can parse it correctly.
You can provide a valid numeric string
(only digits) or an integer
value to the float function to work perfectly.
Code:
h = input("Enter you height:")
print(type(h))
height = float(h)
print("\nYour height is:", height)
print(type(height))
Output:
Enter you height:5.4
<class 'str'>
Your height is: 5.4
<class 'float'>
The value of height is always in float, but the input statement takes every input as a string. So, in the above case, the user entered their height "5.4"
whose class is str
, but later we have type cast it to 'float'
.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python