How to Fix the TypeError: Int Object Is Not Iterable Error in Python
- Understanding the TypeError: Int Object Is Not Iterable
- Method 1: Check Your Data Types
- Method 2: Convert the Integer to an Iterable
- Method 3: Use Range for Iteration
- Conclusion
- FAQ
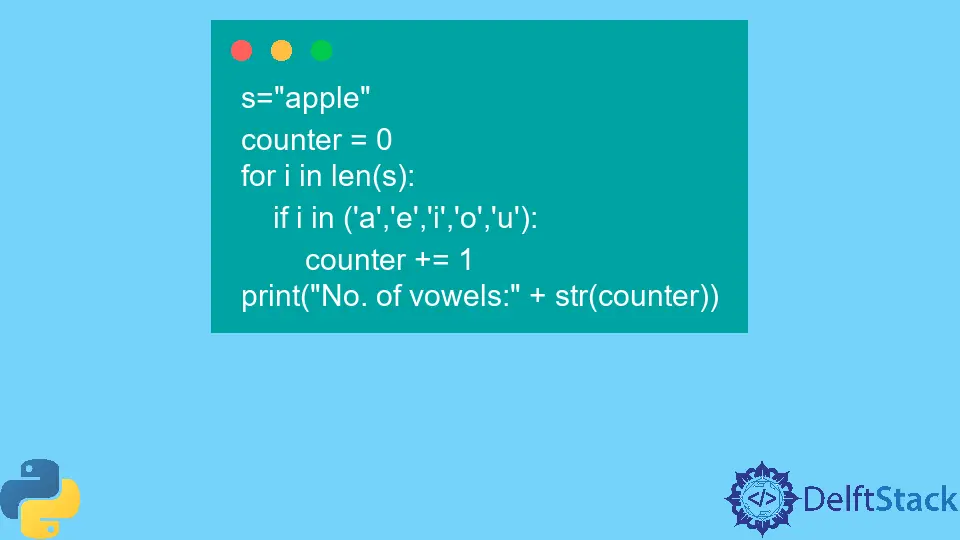
When working with Python, encountering errors is part of the learning process. One common issue developers face is the “TypeError: ‘int’ object is not iterable.” This error can be frustrating, especially for beginners. It typically occurs when you attempt to iterate over an integer as if it were a list or a string.
In this tutorial, we will explore various methods to fix this error, providing clear code examples and detailed explanations to help you understand the underlying concepts. By the end of this article, you will be equipped with the knowledge to troubleshoot and resolve this error effectively.
Understanding the TypeError: Int Object Is Not Iterable
Before we dive into solutions, it’s essential to understand what this error means. In Python, an iterable is any object capable of returning its members one at a time, allowing it to be looped over in a for-loop or comprehended in a list. Common iterables include lists, tuples, strings, and dictionaries. However, integers are not iterable, which means you cannot loop through them using constructs like for-loops or list comprehensions.
For instance, if you mistakenly try to iterate over an integer, Python will raise a TypeError. Let’s look at a simple example:
number = 5
for i in number:
print(i)
Output:
TypeError: 'int' object is not iterable
This code snippet attempts to iterate over the integer 5
, which leads to the TypeError. Understanding this fundamental concept is crucial for fixing the error in more complex scenarios.
Method 1: Check Your Data Types
The first step in resolving the TypeError is to ensure that you are working with the correct data types. Often, the error arises from a misunderstanding of what type of data you are dealing with. For instance, if you expect a list but have an integer, you need to verify your variables.
Here’s a code example that highlights this issue:
data = 10
if isinstance(data, int):
print("Data is an integer, not iterable.")
else:
for item in data:
print(item)
Output:
Data is an integer, not iterable.
In this example, we check if data
is an integer using the isinstance()
function. If it is, we print a message indicating that it is not iterable. This check helps prevent the TypeError by ensuring that the subsequent code only attempts to iterate over iterable objects. By validating your data types early on, you can avoid many common errors in Python programming.
Method 2: Convert the Integer to an Iterable
If you find yourself in a situation where you need to iterate over an integer, consider converting it into an iterable format. This can be done by wrapping the integer in a list or another iterable type. Here’s how you can do it:
number = 5
iterable_number = [number]
for i in iterable_number:
print(i)
Output:
5
In this example, we create a list containing the integer 5
. By doing this, we can safely iterate over iterable_number
. This method is particularly useful when you want to maintain the integer’s value while still needing to perform operations that require iterability. By transforming your data into an iterable format, you can effectively sidestep the TypeError.
Method 3: Use Range for Iteration
Another common scenario where the TypeError arises is when you want to iterate a specific number of times based on an integer value. In such cases, using the range()
function can be an effective solution. The range()
function generates a sequence of numbers, which is iterable. Here’s an example:
count = 5
for i in range(count):
print(i)
Output:
0
1
2
3
4
In this code, we use range(count)
to create an iterable sequence from 0
to 4
. This approach is particularly useful when you need to perform an action a specific number of times, such as looping through a list or performing calculations. By leveraging the range()
function, you can avoid the TypeError while achieving your desired functionality.
Conclusion
In conclusion, the “TypeError: ‘int’ object is not iterable” is a common error in Python that can be easily resolved by understanding the nature of your data. By checking your data types, converting integers to iterable formats, and using the range()
function, you can effectively avoid this error. As you continue your journey in Python programming, keep these strategies in mind to troubleshoot and resolve similar issues. Remember, every error is an opportunity to learn and grow as a developer.
FAQ
-
what is the cause of the TypeError: int object is not iterable?
This error occurs when you try to iterate over an integer as if it were an iterable object like a list or a string. -
how can I check if a variable is iterable in Python?
You can use the isinstance() function to check if a variable is of an iterable type, such as a list or a string. -
can I convert an integer to a list in Python?
Yes, you can convert an integer to a list by wrapping it in square brackets, e.g., list_variable = [integer]. -
what is the range() function used for?
The range() function generates a sequence of numbers and is commonly used for iterations in loops. -
how do I avoid TypeErrors in my Python code?
To avoid TypeErrors, ensure that you are working with the correct data types and validate your variables before performing operations on them.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python