How to Fix Python TypeError: Int Object Is Not Callable
-
Add Missing Operator to Fix the
TypeError: 'int' object is not callable
in Python -
Change Variable Name to Fix the
TypeError: 'int' object is not callable
in Python
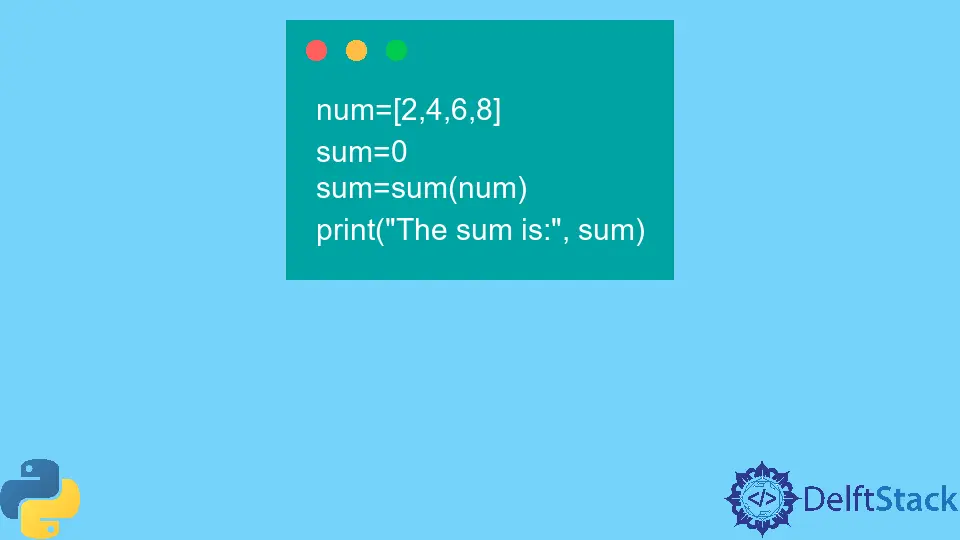
This is one of the common errors you have encountered while coding in Python. There are multiple conditions when Python can throw this error.
Mainly it occurs when you miss the mathematical operator while performing the calculations and use the built-in function as a variable in your code.
This tutorial will discuss all scenarios and the solutions to fix the TypeError: 'int' object is not callable
in Python.
Add Missing Operator to Fix the TypeError: 'int' object is not callable
in Python
Sometimes you might forget to add a mathematical operator to your code. As a result, you will get a TypeError: 'int' object is not callable
.
Let’s take an example of this simple Python script.
marks_obtained = 450
total_marks = 600
percentage = 100(marks_obtained / total_marks)
print("The percentage is:", percentage)
Output:
Traceback (most recent call last):
File "c:\Users\rhntm\myscript.py", line 3, in <module>
percentage=100(marks_obtained/total_marks)
TypeError: 'int' object is not callable
It returns an error because there is a missing multiplication operator in the percentage calculation code. You can fix this issue by adding a multiplication operator *
in your code.
marks_obtained = 450
total_marks = 600
percentage = 100 * (marks_obtained / total_marks)
print("The percentage is:", percentage)
Output:
The percentage is: 75.0
Change Variable Name to Fix the TypeError: 'int' object is not callable
in Python
If you use the built-in function name for the variable and call the function later, you will get an error saying 'int' object is not callable
in Python.
In the following example, we have declared a sum
variable and the sum()
is a built-in function in Python to add the items of an iterator.
num = [2, 4, 6, 8]
sum = 0
sum = sum(num)
print("The sum is:", sum)
Output:
Traceback (most recent call last):
File "c:\Users\rhntm\myscript.py", line 3, in <module>
sum=sum(num)
TypeError: 'int' object is not callable
Since we have used a sum
variable and later called the sum()
function to calculate the sum of the numbers in a list, it raises a TypeError
because the sum
variable overrides the sum()
method.
You can fix such types of errors by renaming the variable name. Here, we will change the variable sum
to total
.
num = [2, 4, 6, 8]
total = 0
total = sum(num)
print("The sum is:", total)
Output:
The sum is: 20
As you can see, the code runs successfully this time.
Now you know how to fix 'int' object is not callable
errors in Python. We hope you found the solutions helpful.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python