How to Solve the Graphviz Executables Are Not Found Error in Python
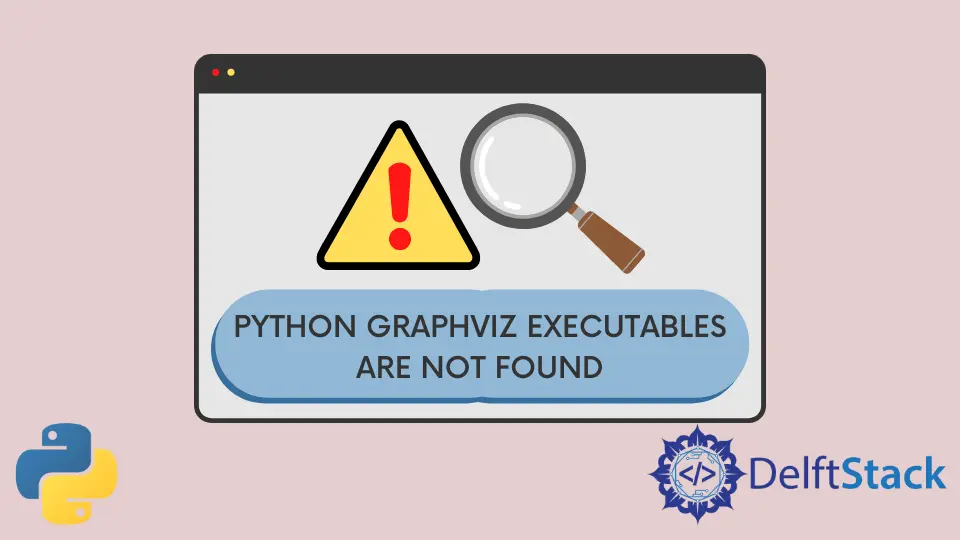
Graphviz is a software package with open-source tools that help draw graphs and is available on Linux, Windows, and macOS.
Within Python, we can create graphs with the Graphviz executables alongside the package. However, people often get into problems when using it for different reasons.
This article explains how to solve the Graphviz executables are not found
error when running our Python scripts.
Install Graphviz to Solve the Graphviz executables are not found
Error in Python
Before we show you how to solve the error, let us write a code to create a simple graph using the Graphviz Python package (assuming you have installed the Graphviz python package).
import graphviz
dot = graphviz.Digraph(comment="The Round Table")
dot.node("A", "King Arthur")
dot.node("B", "Sir Bedevere the Wise")
dot.node("L", "Sir Lancelot the Brave")
dot.edges(["AB", "AL"])
dot.edge("B", "L", constraint="false")
print(dot.source)
dot.render("doctest-output/round-table.gv", view=True)
The output of the code above:
// The Round Table
digraph {
A [label="King Arthur"]
B [label="Sir Bedevere the Wise"]
L [label="Sir Lancelot the Brave"]
A -> B
A -> L
B -> L [constraint=false]
}
Traceback (most recent call last):
File "C:\Python310\lib\site-packages\graphviz\backend\execute.py", line 81, in run_check
proc = subprocess.run(cmd, **kwargs)
File "C:\Python310\lib\subprocess.py", line 501, in run
with Popen(*popenargs, **kwargs) as process:
File "C:\Python310\lib\subprocess.py", line 969, in __init__
self._execute_child(args, executable, preexec_fn, close_fds,
File "C:\Python310\lib\subprocess.py", line 1438, in _execute_child
hp, ht, pid, tid = _winapi.CreateProcess(executable, args,
FileNotFoundError: [WinError 2] The system cannot find the file specified
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\HTML\python\graphz.py", line 11, in <module>
dot.render('doctest-output/round-table.gv', view=True)
File "C:\Python310\lib\site-packages\graphviz\_tools.py", line 171, in wrapper
return func(*args, **kwargs)
File "C:\Python310\lib\site-packages\graphviz\rendering.py", line 122, in render
rendered = self._render(*args, **kwargs)
File "C:\Python310\lib\site-packages\graphviz\_tools.py", line 171, in wrapper
return func(*args, **kwargs)
File "C:\Python310\lib\site-packages\graphviz\backend\rendering.py", line 324, in render
execute.run_check(cmd,
File "C:\Python310\lib\site-packages\graphviz\backend\execute.py", line 84, in run_check
raise ExecutableNotFound(cmd) from e
graphviz.backend.execute.ExecutableNotFound: failed to execute WindowsPath('dot'), make sure the Graphviz executables are on your systems' PATH
The important part of the error message is below:
graphviz.backend.execute.ExecutableNotFound: failed to execute WindowsPath('dot'), make sure the Graphviz executables are on your systems' PATH
The issue is that the Graphviz executables
are unavailable to the Python code and are not present within the PATH
variable. The PATH
variable allows us to access software from any directory besides the software executable directory.
Therefore, if you do not have Graphviz, you must install it. You must add it to your system’s PATH
if you have it installed.
Consequently, we need two things to run a Graphviz code successfully.
- The Library
- The Software itself
We already have the library via pip
:
pip install graphviz
To install Graphviz, go to its download page, and follow the download instructions for each OS. For Linux OS (e.g., Ubuntu, Fedora), you can use the following commands:
sudo apt install graphviz
sudo yum install graphviz
For macOS, you can use the following command.
sudo port install graphviz
However, if you have Homebrew installed, you can use:
brew install graphviz
There are different means and processes to install it for Windows, via Chocolatey, Windows Package Manager, Cygwin, or typical Windows packages. Here, we will use the typical installation package (64-bit version 5.0.0) which is roughly about 4.728 MB.
-
Open the installation file and Agree to the License Agreement.
-
Add Graphviz to the system
PATH
, which is very important to make your code work. Depending on your work environment, you can add the systemPATH
for all users or current users.The image below selects for all users.
-
Select install location and install.
To verify Graphviz is within our environment variable, we can follow the following steps:
-
Search for
environment variables
. -
Go to the highlighted button
Environment variables
. -
Go to the highlighted row with the variable name
Path
and double-click on it. -
If you recently installed Graphviz, you should use the installation path (highlighted) towards the end, as in the image below
Now that we have verified that Graphviz is within our system path as suggested by a sub-part of the error message, make sure the Graphviz executables are on your systems' PATH
, close your IDE and re-run your Graphviz-based code.
The code we ran earlier that resulted in an error should now render and export a PDF.
And your output should be without any error.
// The Round Table
digraph {
A [label="King Arthur"]
B [label="Sir Bedevere the Wise"]
L [label="Sir Lancelot the Brave"]
A -> B
A -> L
B -> L [constraint=false]
}
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python