How to Fix Python File Not Found Error
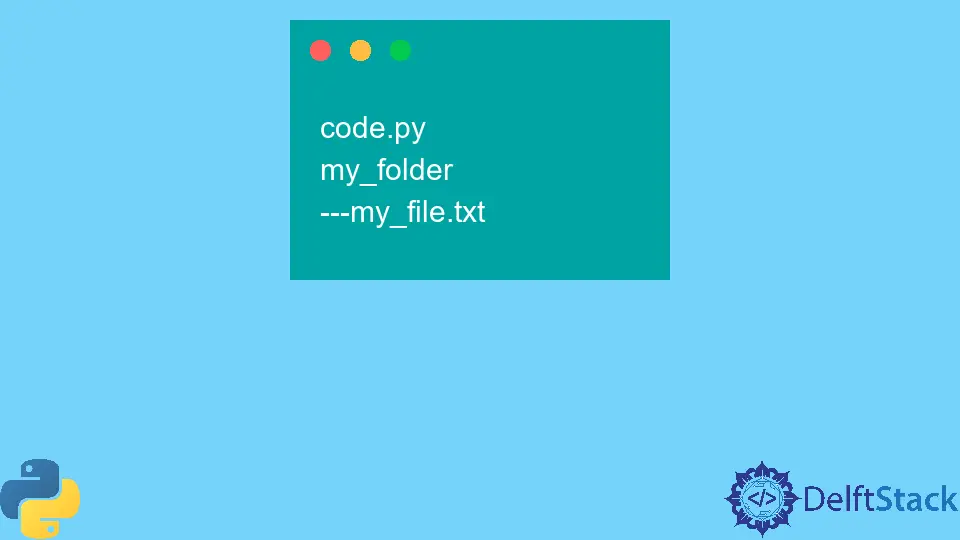
The FileNotFoundError
is a popular error that arises when Python has trouble finding the file you want to open.
This article will discuss the FileNotFoundError
in Python and its solution.
File I/O in Python
Python has built-in functions which are used to modify files. A file is an object stored in the storage device on the computer.
Python’s open()
function is used to open files. It has two parameters.
Parameter | Description |
---|---|
filename |
The file’s name that you want to open. |
mode |
The operation which you want to do on the file. |
There are several modes that allow different operations.
Mode | Usage |
---|---|
r |
Open and read a file that already exists. |
a |
Open and append data to a file that already exists. |
w |
Open an existing file to write data onto it. Creates a new file if no file of the same name exists. |
Causes of FileNotFoundError
in Python
When opening files, an error called FileNotFoundError
may arise if Python cannot find the specified file to be opened. The example code below will produce the error that follows it.
Example Code:
# Python 3.x
file = open("text.txt", "r")
Output:
#Python 3.x
Traceback (most recent call last):
File "c:/Users/LEO/Desktop/Python/main.py", line 2, in <module>
datafile = open('text.txt','r')
FileNotFoundError: [Errno 2] No such file or directory: 'text.txt'
Reason 1 - File Not Present in the Current Directory
Usually, the primary reason is that the file is not in the same folder from which the code is being run. By default, the open()
function looks for the file in the same folder as where the code file is.
Suppose the directory structure looks like this.
code.py
my_folder
---my_file.txt
The error will raise if the user opens the my_file.txt
using the following code.
Example Code:
# Python 3.x
file = open("my_file.txt", "r")
Output:
#Python 3.x
---------------------------------------------------------------------------
FileNotFoundError Traceback (most recent call last)
<ipython-input-4-0fc1710b0ae9> in <module>()
----> 1 file = open('my_file.txt','r')
FileNotFoundError: [Errno 2] No such file or directory: 'my_file.txt'
Reason 2 - Incorrect File Name or Extension
The user specifies an incorrect file name or extension if the error is faced even when the file is in the correct directory.
Suppose the user has a file named my_file.txt
. If the filename or the extension is incorrect, the error will raise in both situations.
Example Code:
# Python 3.x
file = open("my_file2.txt", "r")
Output:
#Python 3.x
---------------------------------------------------------------------------
FileNotFoundError Traceback (most recent call last)
<ipython-input-5-4dd25a062671> in <module>()
----> 1 file = open('my_file2.txt','r')
FileNotFoundError: [Errno 2] No such file or directory: 'my_file2.txt'
Here’s another example.
Example Code:
# Python 3.x
file = open("my_file.jpg", "r")
Output:
#Python 3.x
---------------------------------------------------------------------------
FileNotFoundError Traceback (most recent call last)
<ipython-input-6-d1645df0ff1f> in <module>()
----> 1 file = open('my_file.jpg','r')
FileNotFoundError: [Errno 2] No such file or directory: 'my_file.jpg'
Now let’s discuss the solution to the FileNotFoundError
in Python.
Solution 1 - Specify the Complete File Path
The first thing to check is whether the target file is in the correct folder. If it is not in the same folder as the code file, then it should be moved to the same folder as the code file.
If this is not an option, then you should give the complete file’s path in the file name parameter of the open function. File paths work in the following way on Windows:
C:\Users\username\filename.filetype
The complete path to the file must be given in the open function. An example code with a dummy path is mentioned below.
Example Code:
# Python 3.x
file = open(r"C:\Folder1\Subfolder1\text.txt")
Solution 2 -Specify Correct File Name and Extension
You can re-check the file name and the extension you want to open. Then write its correct name in the open()
method.
Suppose you want to open my_file.txt
. The code would be like the following.
Example Code:
# Python 3.x
file = open("my_file.txt", "r")
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python