How to Check if a Number Is Even or Odd in Python
-
Check Whether a Number Is Even or Odd With the
%
Operator in Python -
Check Whether a Number Is Even or Odd With the
&
Operator in Python
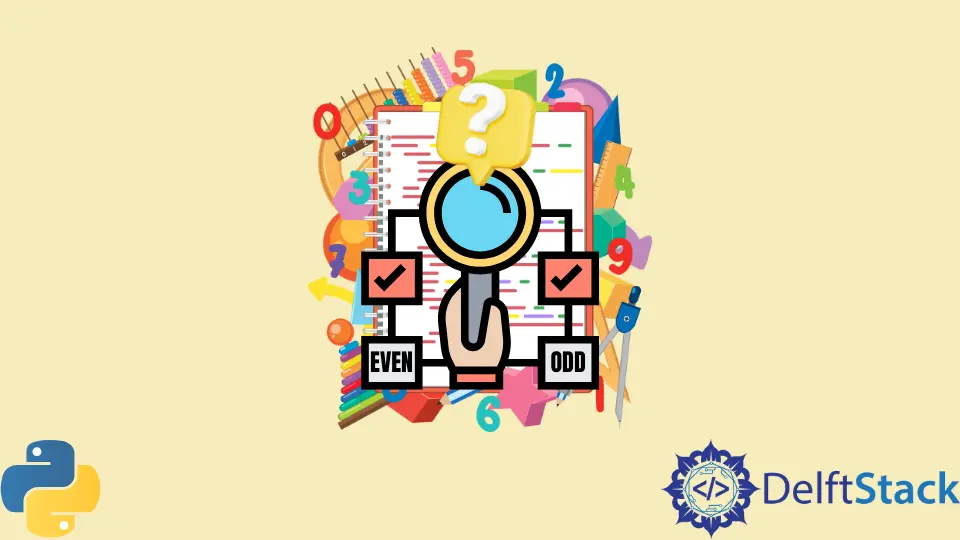
This tutorial will discuss the methods to check whether a number is even or odd in Python.
Check Whether a Number Is Even or Odd With the %
Operator in Python
By definition, a whole number that is completely divisible by 2 is known as an even number. In other words, a whole number is even if after division by 2 we get 0 as remainder. In mathematics, all whole numbers other than even numbers are odd numbers. According to another definition, even numbers are called even because we can evenly split them into two halves. For example, 10 is an even number because we can evenly divide 10 into two halves of 5. On the contrary, 11 cannot be divided into two whole equal numbers because 11 is an odd number.
In python, the modulus operator %
divides the first number by the second number and gives us the remainder of the division. There is a way to determine whether a number is odd or even by checking if the remainder after division is equal to 0 or not. The following code snippet shows us how to check whether a number is even or odd with the modulus operator %
.
def check(num):
if num % 2 == 0:
print("even")
else:
print("odd")
check(22)
Output:
even
We defined the check(num)
that checks whether the num
is completely divisible by 2 with the help of the %
operator. If the remainder is equal to 0, the number is even. If the remainder isn’t 0, the number is odd.
Check Whether a Number Is Even or Odd With the &
Operator in Python
Another clever way of determining whether a number is even or odd is by using the bitwise AND operator &
. As we all know, everything in the computer is stored in the form of 1s and 0s, or binary language in other words. The bitwise AND operator &
converts the values to binary and then performs an AND operation on each bit of the binary expression.
For example, the binary value of the decimal number 11 is (1011), and the decimal number 1 is (0001), respectively. If we perform bitwise and operation on these two decimal numbers, the &
operator takes each bit in both numbers, performs AND operator on them, and returns the results bit-by-bit. In the above case, the returned value would be (0001), which is equal to 1 in decimal.
Another interesting fact about this phenomenon is that if we take the bitwise AND operation of an even number and 1, the result would always be 0. Otherwise, if we take the bitwise AND operation of an odd number and 1, the result would always be 1.
The sample code below shows how we can use the bitwise AND operator &
to check whether a number is odd or even.
def check(num):
if num & 1 == 0:
print("even")
else:
print("odd")
check(33)
Output:
odd
We defined the check(num)
that checks whether the bitwise AND operation of num
and 1 is equal to 0 or not. If the result is equal to 0, the number is even. If the result isn’t 0, the number is odd.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn