How to Use Euler's Number in Python
-
Use
math.e
to Get Euler’s Number in Python -
Use
math.exp()
to Get Euler’s Number in Python -
Use
numpy.exp()
to Get Euler’s Number in Python
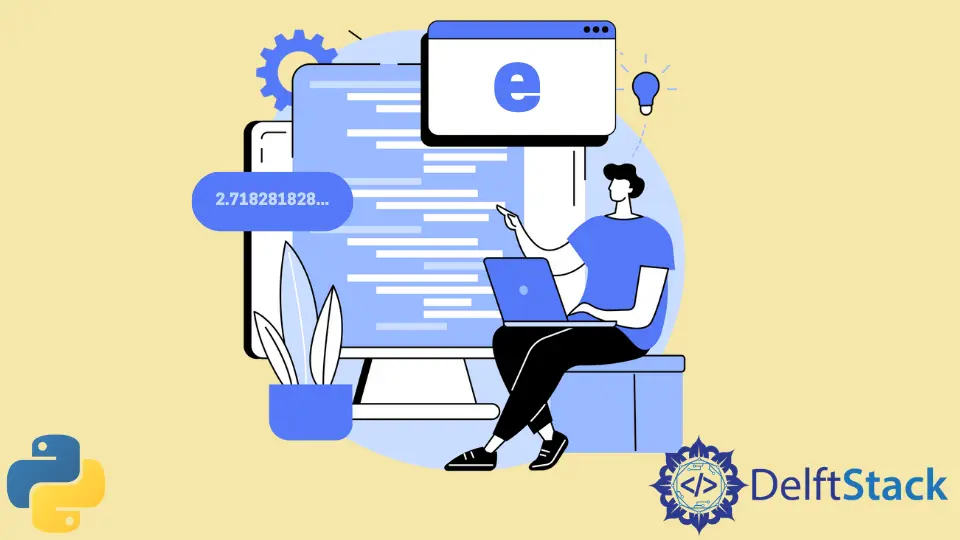
Euler’s number or e
is one of the most fundamental constants in mathematics, much like pi
. e
is the base of natural logarithmic functions. It is an irrational number representing the exponential constant.
This tutorial will demonstrate how to replicate the Euler’s number (e
) in Python.
There are three common ways to get the euler’s number and use it for an equation in Python.
- Using
math.e
- Using
math.exp()
- Uusing
numpy.exp()
Use math.e
to Get Euler’s Number in Python
The Python module math
contains a number of mathematical constants that can be used for equations. Euler’s number or e
is one of those constants that the math
module has.
from math import e
print(e)
Output:
2.718281828459045
The output above is the base value of the e
constant.
As an example equation, let’s create a function get the value of e^n
or e
to the power of a number n
where n = 3
.
Also, note that the syntax for the power operation in Python is double asterisks **
.
from math import e
def getExp(n):
return e ** n
print(getExp(3))
Output:
20.085536923187664
If you prefer to have control over the number of decimal places the result has, a way to achieve this is by formatting the value as a string and printing it out after formatting it.
To format a float value to n
decimal places, we can use the format()
function over a string with this syntax {:.nf}
where n
is the number of decimal places to be displayed.
For example, using the same example above, format the output to 5 decimal places.
def getExp(n):
return "{:.5f}".format(e ** n)
print(getExp(3))
Output:
20.08554
Use math.exp()
to Get Euler’s Number in Python
The module math
also has a function called exp()
that returns the value of e
to the power of the number. Compared to math.e
, the exp()
function performs considerably faster and includes code that validates the given number parameter.
For this example, try using a decimal number as a parameter.
import math
print(math.exp(7.13))
Output:
1248.8769669132553
Another example would be getting the actual base value of e
by setting the parameter to 1
to determine the value.
import math
print(math.exp(1))
Output:
2.718281828459045
The output is the actual value of e
set to 15 decimal places.
Use numpy.exp()
to Get Euler’s Number in Python
The exp()
function within the NumPy
module also does the same operation and accepts the same parameter as math.exp()
.
The difference is that it performs faster than both math.e
and math.exp()
and while math.exp()
only accepts scalar numbers, numpy.exp()
accepts scalar numbers as well as vectors such as arrays and collections.
For example, use the numpy.exp()
function to accept both an array of floating-point numbers and a single integer value.
import numpy as np
arr = [3.0, 5.9, 6.52, 7.13]
singleVal = 2
print(np.exp(arr))
print(np.exp(singleVal))
Output:
[20.08553692 365.03746787 678.57838534 1248.87696691]
7.38905609893065
If an array of numbers is used as a parameter, then it will return back an array of results of the e
constant raised to the power of all the values within the given array. If a single number is given as a parameter, then it will behave exactly like math.exp()
.
In summary, to get the Euler’s number or e
in Python, use math.e
. Using math.exp()
will need a number as a parameter to serve as the exponent value and e
as its base value.
Using exp()
in calculating the exponent of e
over double asterisks, **
performs better than the latter, so if you are dealing with huge numbers, then it’s better to use math.exp()
.
Another option is to use numpy.exp()
, which supports an array of numbers as a parameter and performs faster than both the solutions from the math
module. So if vectors are involved within the equation, use numpy.exp()
instead.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn