How to Python TypeError: Can't Convert 'List' Object to STR
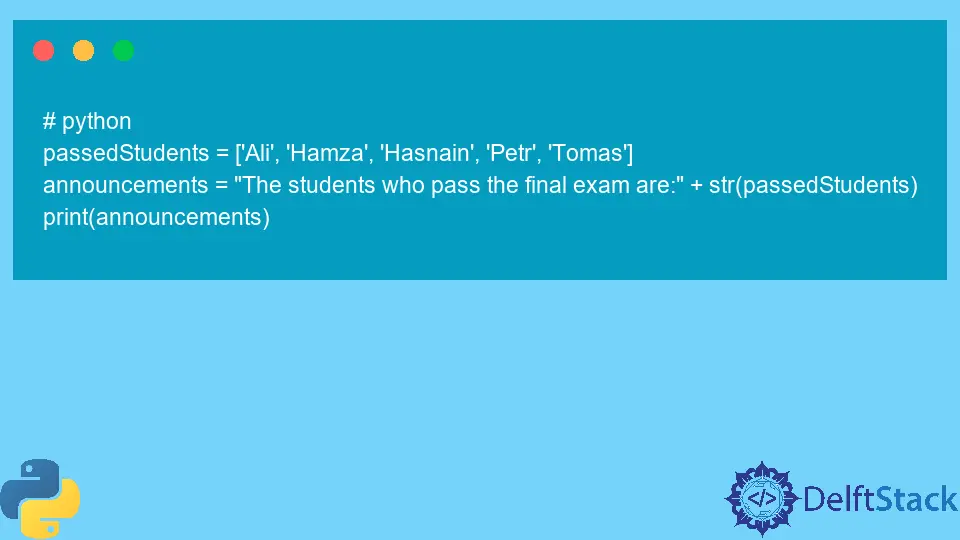
We will use examples to convert a list object to a string in Python. We will also introduce how to concatenate the string with list objects in Python with examples.
Convert List Object to String in Python
While programming in Python, there always comes a time when we need to concatenate objects from lists and arrays into strings to log out an activity or user-friendly messages. If we call a list or arrays directly inside a string, it gives us an error saying we cannot concatenate the string with a list or an array, as shown below.
From the above example, while getting the value from the list, we cannot concatenate it into the string. Two methods in Python can be used to resolve this issue.
We will discuss these methods one by one with some examples.
Use the Join()
Method in Python
If we want to display the objects as a string inside a string, we can use join()
method in Python. The syntax of this method is pretty straightforward, as shown below.
Code Example:
# python
passedStudents = ["Ali", "Hamza", "Hasnain", "Petr", "Tomas"]
announcements = "The students who pass the final exam are:" + " ".join(passedStudents)
print(announcements)
Output:
From the above example, using the join()
method, the list objects are printed one by one and concatenated into the string easily.
Use the str()
Method in Python
The other method that can be used is str()
, which converts any variable, object, array, or list into a string. The syntax of the str()
method is very simple, as shown below.
Code Example:
# python
passedStudents = ["Ali", "Hamza", "Hasnain", "Petr", "Tomas"]
announcements = "The students who pass the final exam are:" + str(passedStudents)
print(announcements)
Output:
From the above example, the str()
method converts the list into a string instead of converting objects one by one. Whereas if we use the join()
method, it converts and concatenates the objects one by one.
It is preferred to use the str()
method on variables only for a better result, and the join()
method can be used on lists and arrays. But you can use both of these methods according to your requirements.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python