How to Fix Error: else & elif Statements Not Working in Python
- Understanding the Structure of Conditional Statements
- Common Syntax Errors with else and elif Statements
- Fixing Indentation Errors
- Ensuring Proper Use of Colons
- Using Logical Operators Correctly
- Conclusion
- FAQ
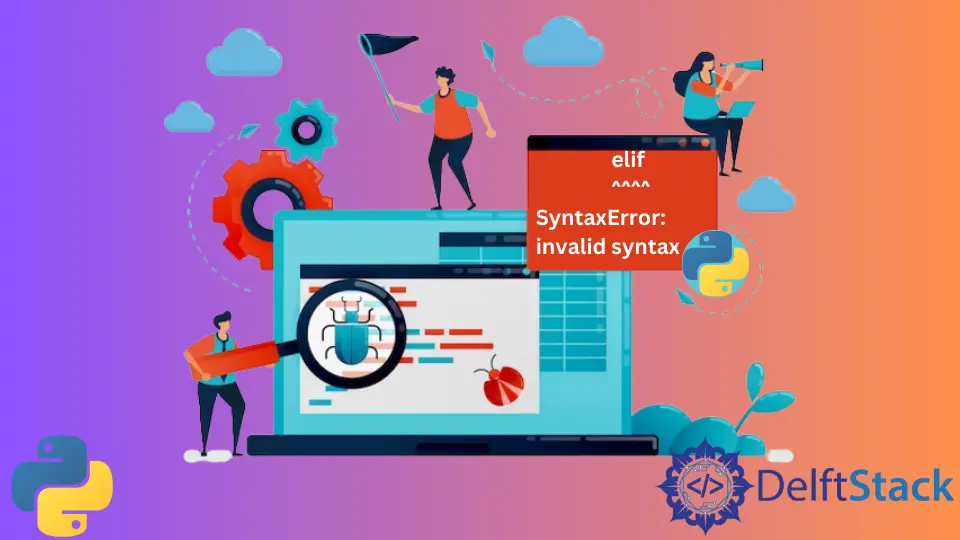
When working with Python, you might encounter frustrating syntax errors related to else
and elif
statements. These errors can halt your program and leave you scratching your head. Understanding how to fix these issues is crucial for anyone looking to master Python programming.
In this tutorial, we will explore common pitfalls associated with else
and elif
statements and provide clear, practical solutions. By the end, you’ll be equipped to troubleshoot and resolve these errors effectively, allowing your Python scripts to run smoothly. Whether you’re a beginner or an experienced developer, this guide will enhance your coding skills and boost your confidence in Python.
Understanding the Structure of Conditional Statements
Before diving into solutions, it’s essential to grasp the structure of Python’s conditional statements. The if
, elif
, and else
statements allow you to execute different blocks of code based on certain conditions. Here’s a simple example:
x = 10
if x > 10:
print("x is greater than 10")
elif x == 10:
print("x is equal to 10")
else:
print("x is less than 10")
Output:
x is equal to 10
In this example, the program checks if x
is greater than, equal to, or less than 10. The correct block executes based on the condition. However, if your indentation is off or if you use incorrect syntax, you might encounter errors.
Common Syntax Errors with else and elif Statements
Syntax errors often arise from incorrect indentation, missing colons, or misused keywords. For instance, consider the following problematic code:
x = 5
if x > 10
print("x is greater than 10")
elif x == 5
print("x is equal to 5")
else
print("x is less than 10")
Output:
SyntaxError: invalid syntax
The missing colons at the end of each conditional statement lead to a SyntaxError
. Python is sensitive to indentation and syntax, so it’s crucial to adhere to its rules.
Fixing Indentation Errors
One of the most common issues with else
and elif
statements is improper indentation. Python relies on indentation to define the scope of loops and conditionals. Here’s how to fix it:
x = 7
if x > 10:
print("x is greater than 10")
elif x == 7:
print("x is equal to 7")
else:
print("x is less than 10")
Output:
x is equal to 7
In this corrected version, each block of code is properly indented. The use of a consistent number of spaces (usually four) helps Python understand the structure of your code. If you mix tabs and spaces, you might encounter indentation errors, so stick to one method throughout your script.
Ensuring Proper Use of Colons
Another common mistake is forgetting to include colons at the end of the if
, elif
, and else
statements. Colons are essential for Python to recognize that a block of code follows. Here’s an example of how to correct this mistake:
x = 3
if x < 5:
print("x is less than 5")
elif x < 10:
print("x is less than 10")
else:
print("x is greater than or equal to 10")
Output:
x is less than 5
In this example, the colons are correctly placed, allowing Python to execute the code without errors. Always double-check your syntax to ensure that colons are present, as this small detail can lead to significant issues.
Using Logical Operators Correctly
Sometimes, issues arise not from syntax but from the logic of your conditions. If the conditions in your if
, elif
, and else
statements are not structured correctly, you may not achieve the desired outcome. Here’s an example:
x = 20
if x < 10 or x > 15:
print("x is either less than 10 or greater than 15")
elif x == 20:
print("x is equal to 20")
else:
print("x is between 10 and 15")
Output:
x is equal to 20
In this case, using the or
operator allows for multiple conditions to be checked effectively. If you had used an incorrect operator or logical setup, you might not get the intended result. Make sure to review your logical operators to avoid confusion.
Conclusion
Fixing errors related to else
and elif
statements in Python can be straightforward if you understand the common pitfalls. By focusing on proper indentation, ensuring the correct use of colons, and structuring your logical conditions effectively, you can troubleshoot and resolve these issues with ease. Mastering these aspects will not only enhance your coding skills but also make your Python programming experience more enjoyable. Remember, practice makes perfect, so keep coding and refining your skills!
FAQ
- What causes else and elif statements to fail in Python?
Common causes include syntax errors, improper indentation, and missing colons.
-
How can I avoid indentation errors in Python?
Use a consistent number of spaces or tabs throughout your code and avoid mixing them. -
Are colons necessary in Python conditional statements?
Yes, colons are essential to indicate that a block of code follows the statement. -
Can logical operators affect the execution of my if statements?
Absolutely. Incorrect use of logical operators can lead to unexpected results in your condition checks. -
What should I do if I encounter a SyntaxError?
Review your code for missing colons, incorrect indentation, or any other syntax issues.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python