How to Write Dictionary Into CSV in Python
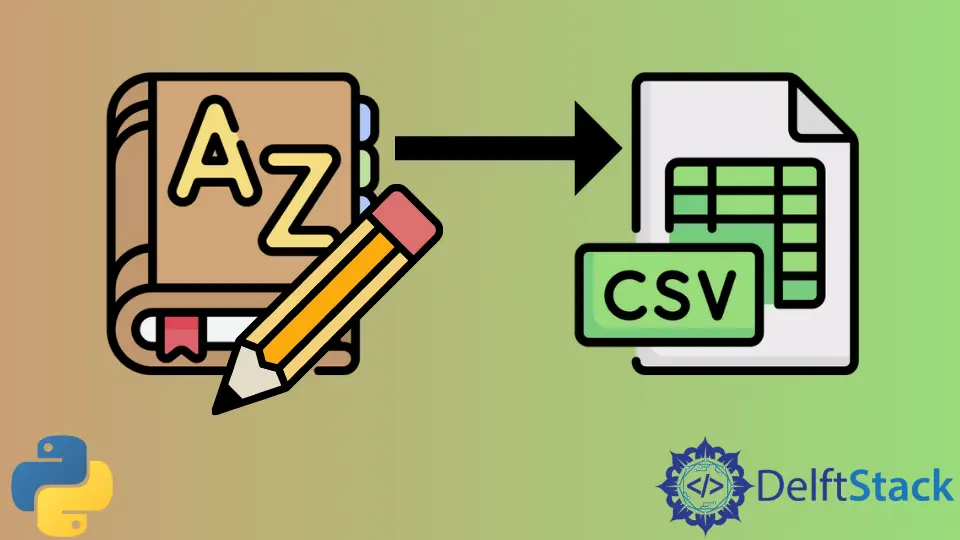
This tutorial will introduce how to write a dictionary variable into a csv file in Python.
Use the csv
Module to Write a Dictionary Into CSV File in Python
The Python module csv
contains tools and functions to manipulate csv files. There are two easy methods to use for writing dictionaries into a csv file: writer()
and DictWriter()
.
These two methods have similar functions; the only difference is that DictWriter()
is a wrapper class that contains more functions.
Let’s set an initial example with a single dictionary with a few key-value pairs:
dct = {"Name": "John", "Age": "23", "Country": "USA"}
In the first example, we use writer()
to access a new csv file and insert the dictionary into it.
import csv
dct = {"Name": "John", "Age": "23", "Country": "USA"}
with open("dct.csv", "w") as f:
writer = csv.writer(f)
for k, v in dct.items():
writer.writerow([k, v])
Since the file access type is w
, the contents of the csv file dct.csv
will be overridden by the new changes. If the file does not exist, then it will be automatically created in the same directory.
The content of the csv file will output:
Since there is only one dictionary entry, the csv file’s layout contains all the keys in the first column, and the values are in the second column.
Example of Using an Array of Dictionaries
That was an example of a single dictionary. What if you want to insert multiple dictionaries in a single csv file?
For this example, the function DictWriter()
will be used to write into a csv file. The first example’s CSV layout would need also to be changed since there are multiple values with the same keys.
The first row should contain the key labels, and the consequent rows would contain the values for each dictionary entry.
First, declare an array of dictionaries with the same key values.
dct_arr = [
{"Name": "John", "Age": "23", "Country": "USA"},
{"Name": "Jose", "Age": "44", "Country": "Spain"},
{"Name": "Anne", "Age": "29", "Country": "UK"},
{"Name": "Lee", "Age": "35", "Country": "Japan"},
]
Now write this array of dictionaries into a csv file using the csv
module and DictWriter()
.
import csv
labels = ["Name", "Age", "Country"]
dct_arr = [
{"Name": "John", "Age": "23", "Country": "USA"},
{"Name": "Jose", "Age": "44", "Country": "Spain"},
{"Name": "Anne", "Age": "29", "Country": "UK"},
{"Name": "Lee", "Age": "35", "Country": "Japan"},
]
try:
with open("csv_dct.csv", "w") as f:
writer = csv.DictWriter(f, fieldnames=labels)
writer.writeheader()
for elem in dct_arr:
writer.writerow(elem)
except IOError:
print("I/O error")
The parameter fieldnames
in DictWriter()
is assigned the variable labels
, which is an array of the array of dictionaries’ key labels.
It’s also good to practice wrapping code that manipulates files with exception handling (try ... except
). This is in case there would be external errors or incompatibilities regarding the file writing process.
Output:
In summary, the csv
module contains all the necessary functions and tools to write a dictionary into a csv file. Using the functions writer()
and DictWriter()
both can easily be used to achieve this.
It’s also good to note to wrap blocks that manipulate files with exception handling to ensure IO errors are caught before it breaks something.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python