How to Get Dictionary Intersection in Python
- Python Dictionary
- Use Dictionary Comprehension to Intersect Two Dictionaries in Python
-
Use the Bitwise
&
Operator to Intersect Two Dictionaries in Python -
Use the Set
intersection()
Method to Intersect Two Dictionaries in Python - Methods Used in Intersecting Multiple Dictionaries in Python
- Conclusion
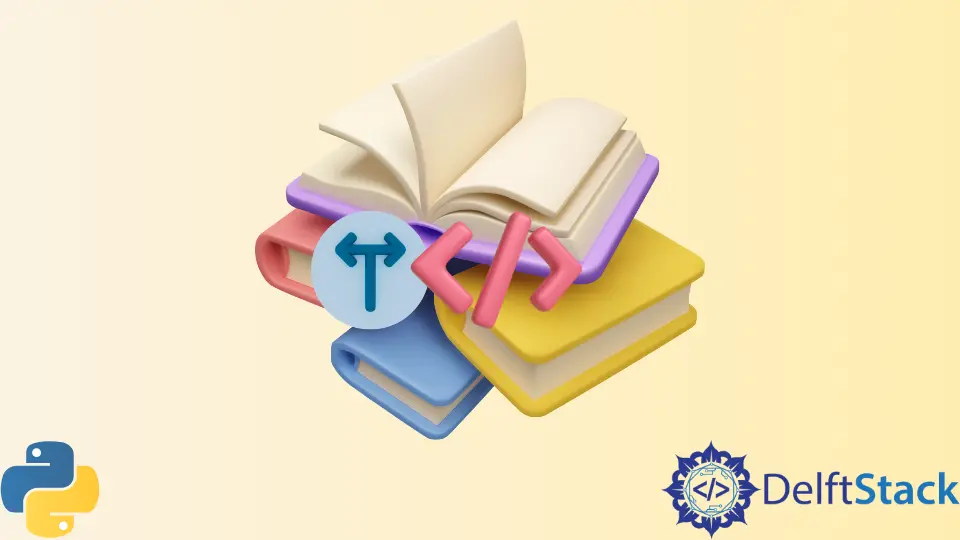
This article will discuss the different methods to perform intersection on two or multiple dictionaries in Python.
Python Dictionary
The data is kept in a key-value pair format using a Python dictionary, and it is a mutable data structure. Python’s dictionary data type can mimic real-world data arrangements where a certain value exists for a given key.
Keys and values are the elements used to define the dictionary:
- Keys should be a single element.
- Value can be any type, such as a list, integer, tuple, etc.
A dictionary is, in other words, a group of key-value pairs where the value can be any Python object. The keys, in contrast, are immutable Python objects, such as strings, tuples, or numbers.
Multiple key-value pairs can be used to build a dictionary, with a colon separating each key from its value before each pair is contained in curly brackets.
The syntax is as follows:
dict = {"Name": "John", "Age": 18, "Grade": "13"}
Let’s look at an example:
Use Dictionary Comprehension to Intersect Two Dictionaries in Python
We can use different approaches to perform the intersection of dictionaries via their keys in Python.
-
First, initialize the original dictionaries into
dict01
anddict02
to start with. -
Print the original dictionaries.
-
Next, consider the key of
dict01
ask1
and execute thefor
loop to check whether thek1
indict01
exists indict02
. If it does have the common key, its value is pushed into a new dictionary calledintersection
(the dictionary can be preferred per the requirement). -
Print the new dictionary
intersection
with the common keys and their values.
Example:
# inititializing the dictionaries
dict01 = {"A": 1, "B": 6, "C": 3, "D": 5}
dict02 = {"A": 1, "C": 4, "D": 5, "E": 2}
# printing original dictionary
print("First original dictionary: ", dict01)
print("Second original dictionary: ", dict02)
# performing intersection
intersection = {k1: dict01[k1] for k1 in dict01 if k1 in dict02}
# printing output
print("Intersected Dictionary: ", str(intersection))
You will receive the output listed below if you run the above code.
Consider the key of dict02
as k2
. Execute the for
loop to check whether k2
in dict02
also exists in dict01
by following the same code.
Additionally, if the values are of the string type:
# inititializing the dictionaries
dict01 = {1: "My", 2: "I", 3: "age"}
dict02 = {1: "name", 2: "live", 4: "year"}
# defining a function to merge strings
def mergeStrings(str01, str02):
return str01 + " " + str02
# performing intersection
intersectString = {
k1: mergeStrings(dict01[k1], dict02[k1]) for k1 in dict01.keys() if k1 in dict02
}
# printing output
print(intersectString)
Let us look into the outcome of the above code:
Use the Bitwise &
Operator to Intersect Two Dictionaries in Python
It simply filters the common keys and the key’s corresponding value from the dictionaries. It only filters keys with the same value, and this approach is much simpler to understand and apply.
-
First, set
dict01
anddict02
with the original dictionaries initially. -
Print the original dictionaries next.
-
Next, convert the dictionaries
dict01
anddict02
into the list format using theitems()
function. -
Then, perform the AND using the
&
operator. The common key-value pairs are then converted into a dictionary using thedict()
function and stored in the new dictionaryintersection
. -
Finally, print the new dictionary.
Example:
# inititializing dictionary
dict01 = {"A": 1, "B": 6, "C": 3, "D": 5}
dict02 = {"A": 1, "C": 4, "D": 5, "E": 2}
# printing original dictionary
print("First original dictionary: ", dict01)
print("Second original dictionary: ", dict02)
# performing intersection
intersection = dict(dict01.items() & dict02.items())
# printing output
print("Intersected Dictionary", str(intersection))
You will receive the following outcome if you run the above code.
When performing the Bitwise &
operator for Python dictionary intersection, both the keys and values of the respective keys should also be equal.
Use the Set intersection()
Method to Intersect Two Dictionaries in Python
The set intersection()
method returns a set that is made up of the elements that are present in both sets m
and n
as follows:
m = {"A": 1, "B": 6, "C": 3, "D": 5}
n = {"A": 1, "D": 5, "E": 2}
setM = set(m)
setN = set(n)
setM.intersection(setN)
for item in setM.intersection(setN):
print(item)
If you run the above example code, you will get the results below.
Methods Used in Intersecting Multiple Dictionaries in Python
We can use both the bitwise &
operator and the Set intersection()
method to easily find the elements that intersect in multiple dictionaries with further coding.
Using Bitwise &
Operator:
# inititializing dictionary
dict01 = {"A": 1, "B": 6, "C": 3, "D": 5}
dict02 = {"A": 1, "C": 4, "D": 5, "E": 2}
dict03 = {"A": 1, "C": 9, "D": 5, "E": 1}
# printing original dictionary
print("First original dictionary: ", dict01)
print("Second original dictionary: ", dict02)
print("Third original dictionary: ", dict03)
# performing intersection
intersection = dict(dict01.items() & dict02.items() & dict03.items())
# printing output
print("Intersected Dictionary", str(intersection))
Let us look into the result after executing the above code:
Using Set intersection()
method:
m = {"A": 1, "B": 6, "D": 5, "E": 2}
n = {"A": 1, "D": 5, "E": 2}
t = {"A": 1, "C": 4, "D": 5, "E": 2}
setM = set(m)
setN = set(n)
setT = set(t)
setM.intersection(setN).intersection(setT)
for item in setM.intersection(setN).intersection(setT):
print(item)
Let’s have a look at the result after executing the above code:
Conclusion
To wrap up, we considered quick and efficient ways to perform intersection on two or multiple dictionaries in Python to create a new one. You can select any approach you need based on your preference and use case.
We found the intersection of two or more dictionaries, all at the level of only keys and key-value pairs, sometimes using different functions for the keys in common. These methods are certainly not the only ones; you can think of more, and perhaps more explicit methods for these tasks will be added in later versions of Python.
Though almost all of the proposed bits of code are one-liners, they are all clear and elegant.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.