Dictionary Comprehension in Python
- Understanding Dictionary Comprehension
- Creating Dictionaries from Lists
- Filtering Items in Dictionary Comprehension
- Nested Dictionary Comprehension
- Conclusion
- FAQ
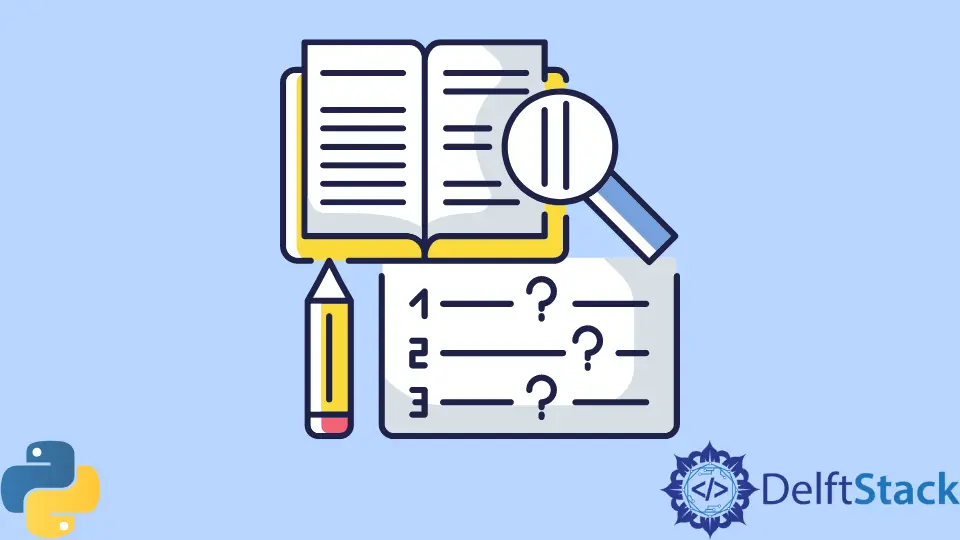
Dictionary comprehension is a powerful feature in Python that allows you to create dictionaries in a concise and readable way. Instead of using traditional loops and conditional statements, dictionary comprehension enables you to generate dictionaries in a single line of code. This not only makes your code cleaner but also enhances performance.
In this tutorial, we will delve into the basics of dictionary comprehension, explore its syntax, and provide practical examples to illustrate how you can leverage this feature in your Python programming. Whether you are a beginner or an experienced developer, understanding dictionary comprehension will undoubtedly improve your coding efficiency.
Understanding Dictionary Comprehension
At its core, dictionary comprehension provides a syntactically elegant way to create dictionaries. The general syntax follows this pattern:
{key_expression: value_expression for item in iterable if condition}
- key_expression: This defines the key of the dictionary.
- value_expression: This defines the value associated with the key.
- iterable: This is any iterable object (like a list or a range).
- condition: This is optional and allows you to filter items.
Let’s break this down with a simple example. Suppose you want to create a dictionary that maps numbers to their squares. Instead of using a for loop, you can achieve this with dictionary comprehension.
squares = {x: x**2 for x in range(1, 6)}
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example, we created a dictionary called squares
where each number from 1 to 5 is mapped to its square. The code is compact and straightforward, showcasing the power of dictionary comprehension.
Creating Dictionaries from Lists
One of the most common uses of dictionary comprehension is to create dictionaries from lists. Imagine you have a list of fruits and their corresponding prices, and you want to create a dictionary that maps each fruit to its price. Here’s how you can do this using dictionary comprehension.
fruits = ['apple', 'banana', 'cherry']
prices = [0.5, 0.25, 0.75]
fruit_prices = {fruits[i]: prices[i] for i in range(len(fruits))}
Output:
{'apple': 0.5, 'banana': 0.25, 'cherry': 0.75}
In this example, we used the fruits
and prices
lists to create a dictionary called fruit_prices
. The comprehension iterates over the indices of the fruits
list, assigning each fruit its corresponding price. This method is not only efficient but also makes the code easier to read.
Filtering Items in Dictionary Comprehension
Another powerful feature of dictionary comprehension is the ability to filter items based on certain conditions. Suppose you want to create a dictionary of even numbers and their squares from a list of numbers. You can easily achieve this by adding a condition to your comprehension.
numbers = range(1, 11)
even_squares = {x: x**2 for x in numbers if x % 2 == 0}
Output:
{2: 4, 4: 16, 6: 36, 8: 64, 10: 100}
In this example, the comprehension iterates through the numbers
range and checks if each number is even. If the condition is met, it adds the number and its square to the even_squares
dictionary. This approach allows you to create more meaningful dictionaries by filtering out unnecessary data.
Nested Dictionary Comprehension
Dictionary comprehension can also be nested, allowing for the creation of complex dictionaries. Consider a scenario where you have a list of students and their scores in different subjects. You can create a nested dictionary where each student has a dictionary of their scores.
students = ['Alice', 'Bob', 'Charlie']
scores = [[85, 90, 95], [80, 85, 83], [78, 88, 92]]
student_scores = {students[i]: {f'Subject {j+1}': scores[i][j] for j in range(len(scores[i]))} for i in range(len(students))}
Output:
{'Alice': {'Subject 1': 85, 'Subject 2': 90, 'Subject 3': 95}, 'Bob': {'Subject 1': 80, 'Subject 2': 85, 'Subject 3': 83}, 'Charlie': {'Subject 1': 78, 'Subject 2': 88, 'Subject 3': 92}}
In this example, student_scores
is a dictionary where each student’s name maps to another dictionary containing their scores in various subjects. This nested structure is particularly useful for organizing complex data in a clear and accessible way.
Conclusion
Dictionary comprehension is an invaluable tool in Python that can significantly enhance your coding efficiency and readability. By allowing you to create dictionaries in a single line of code, it simplifies the process of data manipulation and organization. Whether you’re creating simple mappings or complex nested structures, dictionary comprehension provides a clean and effective solution. With practice, you’ll find that this feature not only makes your code more elegant but also helps you think more critically about data structures in Python.
FAQ
-
What is dictionary comprehension in Python?
Dictionary comprehension is a concise way to create dictionaries in Python using a single line of code. -
How does dictionary comprehension improve code readability?
It allows you to express complex dictionary creation logic in a compact form, making the code easier to understand. -
Can I filter items while creating a dictionary using comprehension?
Yes, you can include a condition in the comprehension to filter items based on specific criteria. -
Is it possible to create nested dictionaries using dictionary comprehension?
Absolutely! You can nest dictionary comprehensions to create complex data structures. -
Are there performance benefits to using dictionary comprehension?
Yes, dictionary comprehension is generally faster than traditional loops for creating dictionaries due to its optimized implementation.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn