Python 사전 교차점
- 파이썬 사전
- Dictionary Comprehension을 사용하여 Python에서 두 개의 사전 교차
-
Bitwise
&
연산자를 사용하여 Python에서 두 개의 사전 교차 -
Set
intersection()
메서드를 사용하여 Python에서 두 사전을 교차 - Python에서 여러 사전을 교차하는 데 사용되는 메서드
- 결론
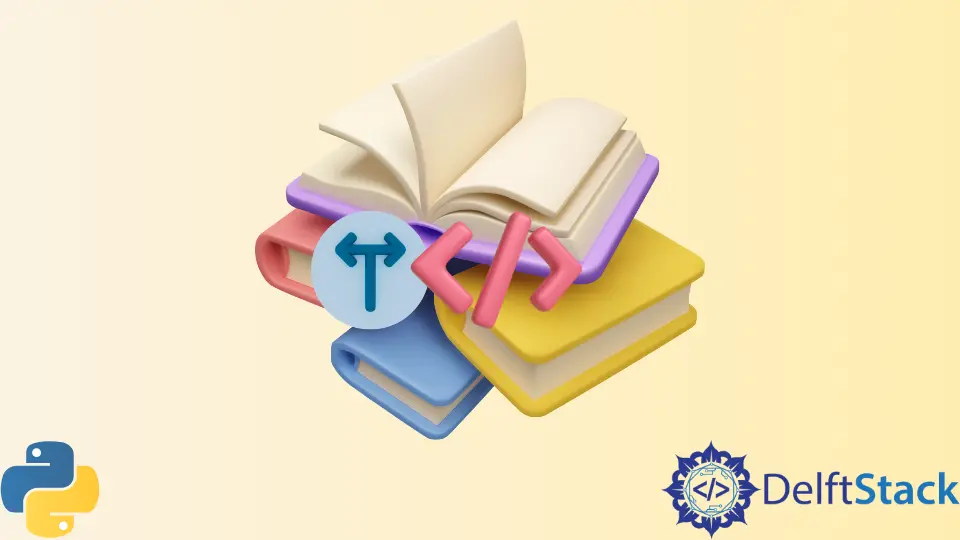
이 기사에서는 Python에서 두 개 또는 여러 개의 사전에서 교차를 수행하는 다양한 방법에 대해 설명합니다.
파이썬 사전
데이터는 Python 사전을 사용하여 키-값 쌍 형식으로 보관되며 변경 가능한 데이터 구조입니다. Python의 사전 데이터 유형은 주어진 키에 대해 특정 값이 존재하는 실제 데이터 배열을 모방할 수 있습니다.
키와 값은 사전을 정의하는 데 사용되는 요소입니다.
- 키는 단일 요소여야 합니다.
- 값은 목록, 정수, 튜플 등과 같은 모든 유형이 될 수 있습니다.
즉, 딕셔너리는 값이 파이썬 객체가 될 수 있는 키-값 쌍의 그룹입니다. 반대로 키는 문자열, 튜플 또는 숫자와 같은 변경할 수 없는 Python 객체입니다.
여러 키-값 쌍을 사용하여 사전을 작성할 수 있으며 각 쌍이 중괄호 안에 포함되기 전에 콜론으로 각 키와 해당 값을 구분합니다.
구문은 다음과 같습니다.
dict = {"Name": "John", "Age": 18, "Grade": "13"}
예를 살펴보겠습니다.
Dictionary Comprehension을 사용하여 Python에서 두 개의 사전 교차
Python에서 키를 통해 사전 교차를 수행하기 위해 다양한 접근 방식을 사용할 수 있습니다.
-
먼저 원래 사전을
dict01
및dict02
로 초기화하여 시작합니다. -
원본 사전을 인쇄합니다.
-
다음으로
dict01
의 키를k1
로 간주하고for
루프를 실행하여dict01
의k1
이dict02
에 존재하는지 확인합니다. 공통 키가 있는 경우 해당 값은intersection
이라는 새 사전으로 푸시됩니다(사전은 요구 사항에 따라 선호될 수 있음). -
공통 키와 해당 값을 사용하여 새 사전
intersection
을 인쇄합니다.
예:
# inititializing the dictionaries
dict01 = {"A": 1, "B": 6, "C": 3, "D": 5}
dict02 = {"A": 1, "C": 4, "D": 5, "E": 2}
# printing original dictionary
print("First original dictionary: ", dict01)
print("Second original dictionary: ", dict02)
# performing intersection
intersection = {k1: dict01[k1] for k1 in dict01 if k1 in dict02}
# printing output
print("Intersected Dictionary: ", str(intersection))
위의 코드를 실행하면 아래 나열된 출력을 받게 됩니다.
dict02
의 키를 k2
로 간주하십시오. 동일한 코드를 따라 for
루프를 실행하여 dict02
의 k2
가 dict01
에도 존재하는지 확인합니다.
또한 값이 문자열 유형인 경우:
# inititializing the dictionaries
dict01 = {1: "My", 2: "I", 3: "age"}
dict02 = {1: "name", 2: "live", 4: "year"}
# defining a function to merge strings
def mergeStrings(str01, str02):
return str01 + " " + str02
# performing intersection
intersectString = {
k1: mergeStrings(dict01[k1], dict02[k1]) for k1 in dict01.keys() if k1 in dict02
}
# printing output
print(intersectString)
위 코드의 결과를 살펴보겠습니다.
Bitwise &
연산자를 사용하여 Python에서 두 개의 사전 교차
단순히 사전에서 공통 키와 키의 해당 값을 필터링합니다. 동일한 값을 가진 키만 필터링하며 이 접근 방식은 이해하고 적용하기가 훨씬 더 간단합니다.
-
먼저 초기에 원래 사전으로
dict01
및dict02
를 설정합니다. -
다음으로 원본 사전을 인쇄합니다.
-
다음으로
dict01
및dict02
사전을items()
함수를 사용하여 목록 형식으로 변환합니다. -
그런 다음
&
연산자를 사용하여 AND를 수행합니다. 공통 키-값 쌍은dict()
함수를 사용하여 사전으로 변환되고 새 사전intersection
에 저장됩니다. -
마지막으로 새 사전을 인쇄합니다.
예:
# inititializing dictionary
dict01 = {"A": 1, "B": 6, "C": 3, "D": 5}
dict02 = {"A": 1, "C": 4, "D": 5, "E": 2}
# printing original dictionary
print("First original dictionary: ", dict01)
print("Second original dictionary: ", dict02)
# performing intersection
intersection = dict(dict01.items() & dict02.items())
# printing output
print("Intersected Dictionary", str(intersection))
위의 코드를 실행하면 다음과 같은 결과가 나타납니다.
Python 사전 교차에 대해 Bitwise &
연산자를 수행할 때 각 키의 키와 값도 동일해야 합니다.
Set intersection()
메서드를 사용하여 Python에서 두 사전을 교차
set intersection()
메서드는 다음과 같이 m
및 n
집합 모두에 있는 요소로 구성된 집합을 반환합니다.
m = {"A": 1, "B": 6, "C": 3, "D": 5}
n = {"A": 1, "D": 5, "E": 2}
setM = set(m)
setN = set(n)
setM.intersection(setN)
for item in setM.intersection(setN):
print(item)
위의 예제 코드를 실행하면 아래와 같은 결과를 얻을 수 있습니다.
Python에서 여러 사전을 교차하는 데 사용되는 메서드
비트 단위 &
연산자와 Set intersection()
메서드를 모두 사용하여 추가 코딩을 통해 여러 사전에서 교차하는 요소를 쉽게 찾을 수 있습니다.
비트 &
연산자 사용:
# inititializing dictionary
dict01 = {"A": 1, "B": 6, "C": 3, "D": 5}
dict02 = {"A": 1, "C": 4, "D": 5, "E": 2}
dict03 = {"A": 1, "C": 9, "D": 5, "E": 1}
# printing original dictionary
print("First original dictionary: ", dict01)
print("Second original dictionary: ", dict02)
print("Third original dictionary: ", dict03)
# performing intersection
intersection = dict(dict01.items() & dict02.items() & dict03.items())
# printing output
print("Intersected Dictionary", str(intersection))
위의 코드를 실행한 후 결과를 살펴보겠습니다.
Set intersection()
메서드 사용:
m = {"A": 1, "B": 6, "D": 5, "E": 2}
n = {"A": 1, "D": 5, "E": 2}
t = {"A": 1, "C": 4, "D": 5, "E": 2}
setM = set(m)
setN = set(n)
setT = set(t)
setM.intersection(setN).intersection(setT)
for item in setM.intersection(setN).intersection(setT):
print(item)
위의 코드를 실행한 후 결과를 살펴보겠습니다.
결론
마무리하기 위해 Python에서 두 개 또는 여러 개의 사전에 대해 교차를 수행하여 새 사전을 만드는 빠르고 효율적인 방법을 고려했습니다. 기본 설정 및 사용 사례에 따라 필요한 접근 방식을 선택할 수 있습니다.
우리는 두 개 이상의 사전이 교차하는 부분을 찾았습니다. 모두 키와 키-값 쌍의 수준에서 공통적으로 키에 대해 서로 다른 기능을 사용하기도 했습니다. 이러한 방법이 유일한 방법은 아닙니다. 더 많은 것을 생각할 수 있으며 아마도 이러한 작업에 대한 더 명시적인 메서드가 이후 버전의 Python에 추가될 것입니다.
거의 모든 제안된 코드는 한 줄짜리이지만 모두 명확하고 우아합니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.